In the last blog, we learnt about Angular.JS
, today, we will write some code and see how this works.
First let’s understand how Angular script works.
This is a simple example of Browser, what happen when we do request for any web page.
Browser requests URL to server and Server response with HTML and JS files that loads in Browser.
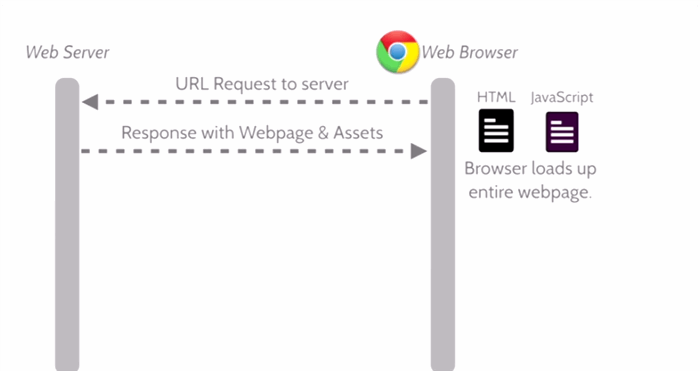
Now, what if we do another request in the traditional way, here we can see again.
Browser again sends URL request to server and server again sends HTML & JS file that entire page loads again.

This is time consuming, congestion in network, flickering of webpage all the time.
So we need something that loads faster without flickering and yes, uses low bandwidth in network.
Here comes Angular in action. We can see this image.

When user request for the second times, server only sends required data and that loads into existing HTML page and pages load faster.
How to implement this??? It is very simple!!!
But before writing any piece of code, we need to learn some part of Angular, i.e., what Angular JS consists of. Basically, it consists of 5 things:
- Module
- Controller
- Router
- Service
- Dependency Injection
For basics, we required only Module, Controller, Directives and Expressions.
Let’s start with Module:
Module is like a container for the different parts of your app – controllers, services, filters, directives, etc.
var myApp = angular.module('myApp',[]);
}]);
Controller: It is a JavaScript constructor function that is used to augment the Angular Scope. We use ng-controller
directive to use controller.
For example:
var myApp = angular.module('myApp',[]);
myApp.controller('BaseController', ['$scope', function($scope) {
$scope.greeting = 'Hola!';
}]);
Directive: These are various Angular defined keywords that are used to take Angular in action.
For example: ng-app
, ng-controller
, ng-click
, ng-show
, etc.
Expression: These are JavaScript-like code snippets that are usually placed in bindings such as {{ expression }} in HTML.
ex:- {{ 1+2 }}, {{a+b}}
Now, let’s start with simple coding.
We want to display the sum of two number using Angular JS.
Open any Editor and create an HTML file, let’s say angular.html and write this piece of code inside.
<!DOCTYPE html>
<html>
<head>
<script data-require="angular.js@*" data-semver="1.3.0"
src="//code.angularjs.org/1.3.0/angular.js"></script>
<link rel="stylesheet" href="style.css" />
<script src="script.js"></script>
</head>
<body ng-app="myApp">
<h1>Hello Angular</h1>
<div ng-controller="MainCtrl">
1st Number : <input type="text" ng-model="num1"><br/>
2nd Number : <input type="text" ng-model="num2">
<input type="button" ng-click="add(num1,num2)" value="ADD"><br/>
Sum : {{result}}
<div>
</body>
</html>
Here, we use ‘ng-app=”myApp”
‘ this tells angular that within this region, our Angular script will work and invoke.
We also used ‘ng-controller=”MainCtrl”
‘. This tells angular where the controller exists and helps to manipulate DOM element.
ng-model=”num1″
, i.e., used for holding variable value and passing to controller.
ng-click=”add(num1,num2)”
i.e., used to call “add()
” method inside controller.
{{result}}
i.e., angular Expression, used how we can display result.
We are done with HTML code, create another file for JS and name it as “script.js” and write the below piece of code inside.
var app = angular.module('myApp', []);
app.controller('MainCtrl', function($scope) {
$scope.add=function(num1,num2)
{
$scope.result=parseInt(num1)+parseInt(num2);
}
});
1st line defines how we write module in script and 3rd line defines how we can use controller.
4th line invokes add
method and returns result back to HTML.
You can see how this works by clicking here.
Hmmm… nice :). Now we are done with a simple mathematical workout for getting output of two number addition using Angular JS. What do we feel!! Isn’t it really easy :). Yes. it is. So, now you can change the logic a bit and check for subtraction, multiplication, division and so on …
Thanks and hope you will like this blog. :)
CodeProject

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.