In my last post, I explained how easily we can add a CheckBox
column to a GridView
in ASP.NET. Having a CheckBox
column in GridView
, now we can select multiple records from the GridView
. We can now write some piece of code with the help of which we can perform multiple actions on the selected rows, like deleting the records, updating records, etc. Continuing to the last post, today we will see how we can use these checkbox
es to get selected rows from the GridView in a PostBack in ASP.NET and display them to the user for deletion.
We will use the same example we used in our last example where we have populated a GridView
with 10 students. We will add a Delete button at the bottom of the GridView
and handle the Button
’s click event handler to get all selected rows of the GridView
and display them to the user for confirmation.
Below is the complete code for ASPX page:
<asp:label ID="lblMsg" runat="server"
Visible="false"
Text="The below records are selected for deletion"></asp:label>
<asp:gridview ID="grdData" runat="server"
AutoGenerateColumns="False" CellPadding="4"
CssClass="grdData"
ForeColor="#333333" GridLines="None"
Width="200" AllowPaging="True"
OnPageIndexChanging="grdData_PageIndexChanging">
<alternatingrowstyle BackColor="White"
ForeColor="#284775"></alternatingrowstyle>
<columns>
<asp:templatefield HeaderText="Select">
<itemtemplate>
<asp:checkbox ID="cbSelect"
CssClass="gridCB" runat="server"></asp:checkbox>
</itemtemplate>
</asp:templatefield>
<asp:boundfield DataField="ID"
HeaderText="ID"></asp:boundfield>
<asp:boundfield DataField="Name"
HeaderText="Name"></asp:boundfield>
</columns>
<editrowstyle BackColor="#999999"></editrowstyle>
<footerstyle BackColor="#5D7B9D"
Font-Bold="True" ForeColor="White"></footerstyle>
<headerstyle BackColor="#5D7B9D"
Font-Bold="True" ForeColor="White"></headerstyle>
<pagerstyle BackColor="#284775"
ForeColor="White" HorizontalAlign="Center"></pagerstyle>
<rowstyle BackColor="#F7F6F3"
ForeColor="#333333"></rowstyle>
<selectedrowstyle BackColor="#E2DED6"
Font-Bold="True"
ForeColor="#333333"></selectedrowstyle>
<sortedascendingcellstyle
BackColor="#E9E7E2"></sortedascendingcellstyle>
<sortedascendingheaderstyle
BackColor="#506C8C"></sortedascendingheaderstyle>
<sorteddescendingcellstyle
BackColor="#FFFDF8"></sorteddescendingcellstyle>
<sorteddescendingheaderstyle
BackColor="#6F8DAE"></sorteddescendingheaderstyle>
</asp:gridview>
<asp:button ID="btnDelete" runat="server"
Text="Delete" OnClick="btnDelete_Click"></asp:button>
Below is the code written for the Delete button’s click handler. To understand the data bound to the GridView
, please refer to my previous article.
protected void btnDelete_Click(object sender, EventArgs e)
{
DataTable dt = new DataTable();
dt.Columns.Add("Id");
dt.Columns.Add("Name");
foreach (GridViewRow item in grdData.Rows)
{
if ((item.Cells[0].FindControl("cbSelect") as CheckBox).Checked)
{
DataRow dr = dt.NewRow();
dr["Id"] = item.Cells[1].Text;
dr["Name"] = item.Cells[2].Text;
dt.Rows.Add(dr);
}
}
lblMsg.Visible = true;
grdData.DataSource = dt;
grdData.DataBind();
}
Below are 2 screenshots showing the rows selected on 1st screen and then the selected rows in 2nd screen.
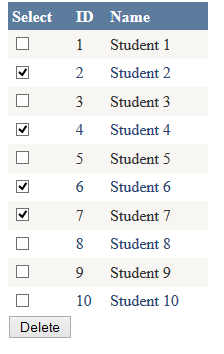
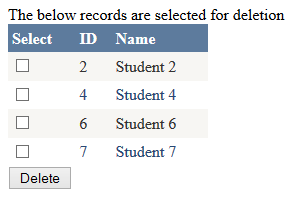
Hope you like this! Keep learning and sharing! Cheers!
A passionate developer with over 10 years of experience and building my software company code by code. Experience withMS Technologies like .Net | MVC | Xamarin | Sharepoint | MS Project Server and PhP along with open source CMS Systems like Wordpress/DotNetNuke etc.
Love to debug problems and solve them. I love writing articles on my website in my spare time. Please visit my Website for more details and subscribe to get technology related tips/tricks. #SOreadytohelp