Introduction
Hi Guys, I would like to introduce PHP functions to connect your Server to FTP server.
I have created an application using PHP functions do it. I have published this application in some websites before.
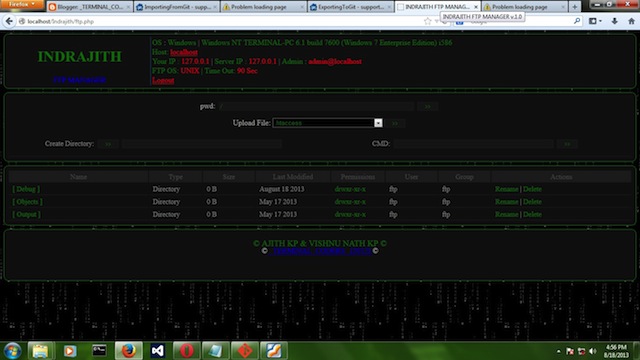
Advantages
I would like to inform its advantages because you will think whats
the use of this PHP script when there are highly advanced application
like "FILEZILLA" is available.
It have its own uses.
- It allows to connect your Server to FTP Server
- You don't want an intermediate computer to upload a file from FTP Server to your Server
Background
This idea of FTP Client using PHP is originated when I want to upload
a file to my server. It is not good when I download the file to my
computer and upload it to my server.
From here the idea of making FTP Client that will help to connect Server to FTP Server
Using the code
Lets check how the application is implemented in PHP.
First of all, I want a storage medium to store the host, username and password of FTP Server. It is not good when use
$user = $_GET['usrname'];
$passwd = $_GET['passwrd'];
$host = $_GET['host'];
I want to store the value of variable in a medium. So I selected
session cookies to store the value of variables for later use. Now the
code will like this,
$user = $_COOKIE['ftpuser'];
$passwd = !$_COOKIE['ftppassword']?"":$_COOKIE['ftppassword'];
$host = $_COOKIE['host'];
So when the user fills the form and submit it, I will store the values of forms like this,
if(isset($_GET['host']) && isset($_GET['usrname']) && isset($_GET['passwrd']))
{
global $user, $passwd, $host;
setcookie("ftpuser", $_GET['usrname']);
setcookie("ftppassword", $_GET['passwrd']);
setcookie("host", $_GET['host']);
}
Now let us check the FTP function of PHP which will help to connect FTP Server. To connect a FTP Server use, $connect = ftp_connect($host);
It will try to connect to FTP Server. The function ftp_connect()
will return a boolean value to $connect< br/>
Now if it is success next step is to login to FTP Server. To login to FTP Server use function ftp_login()
So the code will become, $login = ftp_login($connect, $user, $passwd);
The function ftp_login()
will return a boolean value to $login
Later now next step is to read the files in FTP Server. The are two function to do a listing of files in FTP Server. ftp_nlist()
and ftp_rawlist()
So here, ftp_rawlist()
is advanced type of directory listing because It will return almost every details of files in FTP Server.
function dirDetails($directory=".")
{
global $connect;
if(is_array($directs=ftp_rawlist($connect, $directory)))
{
$prop = array();
$nl=count($directs);
foreach($directs as $dirx)
{
$chunks = preg_split("/[\s]+/", $dirx, 9);
list($prop['perm'], $prop['num'], $prop['user'], $prop['group'], $prop['size'], $prop['mon'], $prop['day'], $prop['time']) = $chunks;
$prop['type']=$chunks[0]{0}==='d'?'Directory':'File';
$prop['name']=$chunks[8];
array_splice($chunks, 0, 8);
$props[implode(" ", $chunks)]=$prop;
}
return $props;
}
}
The function dirDetails()
will read files in directory when we call the function with path as parameter(current worling directory '.' as default).
The inner fuction will split the output from ftp_rawlist()
according to
permission, user, group, size(in bits), last modified date(month, day, time), type of file and name of file.
To show the details of files and directories in the FTP Server, the bellow function is used
$property = dirDetails($fpath);
foreach($property as $prop)
{
global $fpath;
$modified = date("F d Y",ftp_mdtm($connect, $prop['name']));
if($prop['type']=='Directory')
{
print "<a>[ ".$prop["name"]." ]</a>".$prop['type']."".filesizex($prop['size'])."$modified<a>".$prop['perm']."</a>".$prop['user']."".$prop['group']."<a>Rename</a> | <a>Delete</a>";
}
else
{
print "<a>".$prop['name']."</a>".$prop['type']."".filesizex($prop['size'])."$modified<a>".$prop['perm']."</a>".$prop['user']."".$prop['group']."<a>Download</a> | <a>Rename</a> | <a>Delete</a>";
}
}
To download a file from FTP Server $down = ftp_get($resource, $fname, $fname, FTP_ASCII);
here $resource is connection stream, fisrt $fname represents in what name the file download, second $fname represents the remote file and the third parameter FTP_ASCII represents resumepos of download.
function download($resource, $fname)
{
$down = ftp_get($resource, $fname, $fname, FTP_ASCII);
if($down)
{
success("The file ".$fname." downloaded sucessfully");
}
else
{
failed("The file ".$fname." failed to download");
}
}
The above snippet is exact function I used. To delete a file from FTP Server, you can use function ftp_delete($connect, $file);
function deleteFiled($file, $dire)
{
global $connect;
ftp_chdir($connect, $dire);
$del = ftp_delete($connect, $file);
if($del)
{
success("The file $file deleted sucessfully");
}
else {
failed("The file $file cannot delete");
}
}
The above snppet is used by me to delete the file.
To delete a directory you can simply call the function ftp_rmdir($connect, $file);
But it will fail to execute if the directory is not empty. So before
delete directory you should delete all files and directories from
desired directory. You can use a recursive function to do it,
function deleteDir($file, $cdir)
{
global $connect, $fpath;
$arraay = dirDetails("$fpath/$file");
ftp_rmdir($connect, $file);
foreach ($arraay as $key)
{
if($key['type']!='Directory')
{
deleteFiledd($fpath."/".$file."/".$key['name']);
}
else
{
deleteDir($fpath."/".$file."/".$key['name']);
}
}
if(ftp_rmdir($connect, $file))
{
success("The directory $file has deleted succssfully");
}
else
{
failed("The directory $file cannot delete");
}
}
The function deleteDir() will delete a directory successfully if deletion of directory is set to user.
To upload a file from Server to FTP Server the PHP function ftp_put($connect, $file, $file, FTP_ASCII);
will help. The first $file represents name of remote file you like to give and second $file represents the current name of file in server.
The exact function I used in application is,
function uploadf()
{
$file=$_GET['upload'];
global $connect, $host;
$uploadd = ftp_put($connect, $file, $file, FTP_ASCII);
if($uploadd)
{
success("Successfully uploaded file $file to FTP Server $host");
}
else
{
failed("Failed to upload file $file to FTP Server $host");
}
}
Now we have uploaded, downloaded and deleted from server. Now we want
to maintain files in server. I would like to show how to rename a file
in server, the PHP function to rename a file is ftp_rename($resource, $fname, $nfname);
The $fname represent the current name of file and $nfname represents new name for file. Exact function I used is,
function renamef($resource, $fname, $nfname)
{
$ren = ftp_rename($resource, $fname, $nfname);
if($ren)
{
success("The file ".$fname." renamed sucessfully");
}
else
{
failed("The file ".$fname." failed to rename");
}
}
Next is to change permission of file in FTP Server. The PHP function to change permission is ftp_chmod($connect, $mode, $file);
You can use function like this ftp_chmod($connect, 0777, "ajithkp.jpg");
to change permission of file "ajithkp.jpg" to mode 777.
The exact code I used is,
function changeperm()
{
global $connect, $fpath;
ftp_chdir($connect, $fpath);
$mode = $_GET['new_perm'];
$file = $_GET['fname'];
$mode = octdec(str_pad($mode, 4, '0', STR_PAD_LEFT));
if(ftp_chmod($connect, $mode, $file) != FALSE)
{
success("The file $file permission changed successfully");
}
else
{
failed("The file $file permission cannot changed");
}
}
The other functions I used in this application are bellow. To convert bit size to KB, MB and GB, I used the bellow function,
function filesizex($size)
{
if ($size>=1073741824)$size = round(($size/1073741824) ,2)." GB";
elseif ($size>=1048576)$size = round(($size/1048576),2)." MB";
elseif ($size>=1024)$size = round(($size/1024),2)." KB";
else $size .= " B";
return $size;
}
To logout from current session, you need to delete the session
cookies stored in your browser. To delete cookies I used PHP function setcookie("ftpuser", "");
The exact function I used is,
function logout()
{
setcookie("ftpuser", "");
setcookie("ftppassword", "");
setcookie("host", "");
}
To change the current directory: ftp_chdir($connect, $fpath);
To get FTP System Type: ftp_systype($connect);
To get current timeout: ftp_get_option($connect, FTP_TIMEOUT_SEC);
I hope you have enjoyed this article and the application
Name Ajith Kp. Currently working at Tata Consultancy Service Ltd. I completed MCA from School of Information Science and Techonolgy, Kannur University Campus, Mangattuparamba. I like programming as well as computer/network security analyzing. I'm concentrating programming in Android, PHP, Python, Ajax, JQuery, C# and JAVA.
Blog: http://www.terminalcoders.blogspot.com
Knowledge in Java, Python, PHP, and Android.