Introduction
I will demonstrate how to easily get the available screen
size using PageMethods and ASP.NET
Sometimes it may be useful to get the screen
resolution or available height/width of the screen from server code, especially if
you need to resize controls from server side.
I decided to write this because I have
seen terrific things to retrieve screen resolution, such as a page which
redirects to another page.
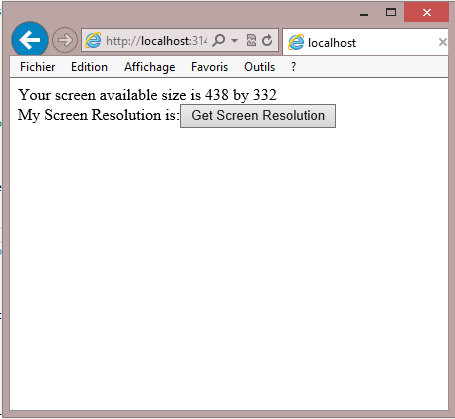
Background
This post simply uses PageMethods to
retrieve user window available size.
Using the Code
The first thing to do is to add a ScriptManager
to our
page, and tell it to accept page Methods.
<asp:ScriptManager ID="ScriptManager1" runat="server" EnablePageMethods="True">
</asp:ScriptManager>
After that I will have my server control which
fires the JavaScript on the OnClientClick
.
As the OnClientClick
gets fired before the
server event, we can easily get our screen resolution.
<asp:Button ID="btnGetScreenResolution" runat="server"
OnClick="btnGetScreenResolution_Click" Text="Get Screen Resolution"
OnClientClick="getScreenResolution();" />
So what is the JavaScript like? In fact,
quite simple:
<script type="text/javascript">
function getScreenResolution() {
PageMethods.setResolution(window.innerWidth, window.innerHeight);
}
</script>
And last but not least, to use PageMethods I
need three things :
- A
ScriptManager
with EnablePageMethods
to true
- The method must be static
- The method must have the
[WebMethod]
attribute
In this case I have created a base page (so my
default.aspx pages inherits from the base page), with the following C#
code:
Code for basepage.cs
...
using System.Drawing;
using System.Web.Services;
..omited for briefty
public static Size ScreenResolution
{
get
{
return (Size)HttpContext.Current.Session["ScreenResolution"];
}
set
{
HttpContext.Current.Session["ScreenResolution"]=value;
}
}
[WebMethod()]
public static void setResolution(int width, int height)
{
ScreenResolution = new Size(width, height);
}
Note that in the above code I’ve used the Size
element from System.Drawing
namespace.
And finally we display the result. You’ll notice
that I call the ScreenResolution
variable from the base page with
inheritance:
public partial class _Default : basepage
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnGetScreenResolution_Click(object sender, EventArgs e)
{
Response.Write(string.Format("Your screen available size is {0} by {1}", ScreenResolution.Width, ScreenResolution.Height));
}
}
So, this wasn’t difficult, was it? And if
you want to test and resize the window, it will work!
Conclusion
So we’ve seen a very basic example of how
PageMethods, plus some other tricks can help us to get JavaScript code from
server side.
I am Web Developer at Messages, a printing company in Toulouse, France. I am particularly interested about Blazor, but my primary development platform at work is ASP.NET MVC with C#. I have 15 years experience in developing software, always using Microsoft Technologies.