Introduction
From the NUnit website, we got the explanation for SetUpFixture
as:
Reference start------------------------------------------------------------------------------
This is the attribute that marks a class that contains the one-time setup or teardown methods for all the test fixtures under a given namespace.
The SetUp
method in a SetUpFixture
is executed once before any of the fixtures contained in its namespace. The TearDown
method is executed once after all the fixtures have completed execution.
Reference end--------------------------------------------------------------------------------------
But what is exactly the "under a given namespace" means? And how to make it work?
Background
Sometimes, we developed a library that can be reused in the application. Such as inject some logging statement when the function gets called. It works well in the function code. But during the unit test, we don't want to waste time on running those statements. So we can add the Flag in the library to bypass the code injection when the unit test is running.
Using the Code
The code for the library can be like this:
namespace MyLibrary
{
public class Program
{
public static bool Flag;
public void Function()
{
if (Flag) return;
DoJob();
}
private void DoJob()
{
}
}
}
So in unit test setup, we can set the Flag
to true
. The unit test will bypass it.
using MyLibrary;
using NUnit.Framework;
namespace MySetup.New
{
[TestFixture]
public class PrgramTest
{
[SetUp]
public void Init()
{
Program.Flag = true;
}
[Test]
public void Test()
{
Assert.IsTrue(Program.Flag);
}
}
}
In the case, we have many TestFixtures
, the SetUpFixture
can be useful. We can create a new class and mark in with SetUpFixture
. In this case, the Flag
will be set for all TestFixtures
.
using MyLibrary;
using NUnit.Framework;
namespace MySetup
{
[SetUpFixture]
public class MySetup
{
[SetUp]
public void Init()
{
Program.Flag = true;
}
}
}
In this case, we don't have to set the flag in each TestFixture
's SetUp
function.
using MyLibrary;
using NUnit.Framework;
namespace MySetup.New
{
[TestFixture]
public class PrgramTest
{
[Test]
public void Test()
{
Assert.IsTrue(Program.Flag);
}
}
}
So how does the namespace matter? We need to pay attention to the namespace. The sample I posted before is working since the class marked by SetUpFixture
and the class marked by TextFixture
are under the same namespace. If I change the class marked by TextFixture
to another namespace, it won't work.
using MyLibrary;
using NUnit.Framework;
namespace My.New
{
[TestFixture]
public class PrgramTest
{
[Test]
public void Test()
{
Assert.IsTrue(Program.Flag);
}
}
}
Now what we tried is that both the class marked by SetUpFixture
and the class marked by TextFixture
are in the same project. But how about the case that in our solution, we have many unit test projects and we want to apply this unit test Flag to all of them. Let's say we build the solution like this:
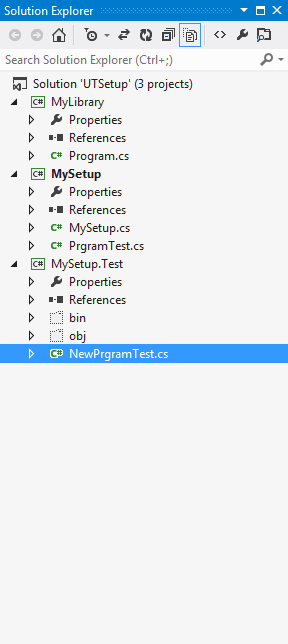
In the new test case, we put it under the same namespace with the class marked by SetUpFixture
.
using MyLibrary;
using NUnit.Framework;
namespace MySetup.Test
{
[TestFixture]
public class NewPrgramTest
{
[Test]
public void Test()
{
Assert.IsTrue(Program.Flag);
}
}
}
But this time, it does not work. The Flag
is still false
although they are under the same namespace.
There is a workaround for this issue. We can add the Setup file as a link file in the new project. It is working.
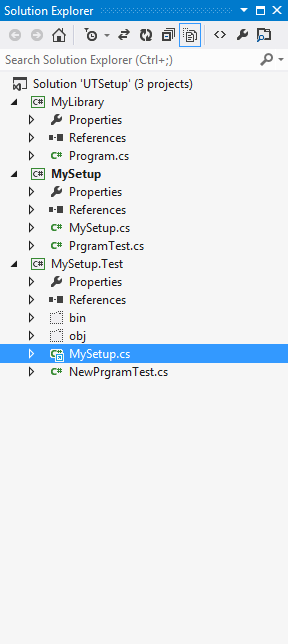
Points of Interest
In this tip, we explore how the SetUpFixture
can be used in Unit Testing and also provide a workaround when we want to apply it to solution-wise in the Visual Studio.
History
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.