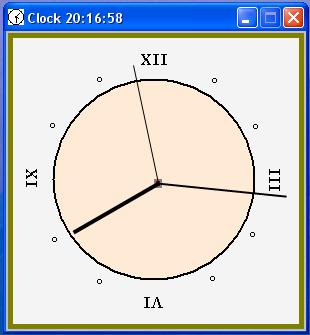
Introduction
This program shows how to make a clock using C#. The program is basically very easy, there is only a little math involved which I think shouldn't be that difficult for any of you programmers out there. The program uses a timer that is fired every .5 seconds; myTimer_Tick()
takes the system's current time (hour, minute, second) and saves them to the appropriate variables. The titlebar is then updated showing the time in numbers, and at last a call to updateClock()
is made, which in turn draws the clock's hands.
updateClock()
makes a new bitmap and copies the bmp image of the clock.bmp (which is saved in the variable 'bitmap
' in the constructor and taken from the program's execution directory) to another Bitmap
variable. Then, it figures out the angle of every hand and draws them on that variable. Finally, our bitmap variable is loaded to the PictureBox
on our form.
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Clock
{
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.PictureBox pictureBox1;
private System.Windows.Forms.Timer myTimer;
private System.ComponentModel.IContainer components;
private int hour, minute, second;
private int xCordinate=150, yCordinate=150;
private int radius;
private Bitmap bitmap;
public Form1()
{
InitializeComponent();
this.Text += " Getting time...";
radius = this.pictureBox1.Width/2;
bitmap = new Bitmap(Application.StartupPath + "\\clock.bmp");
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
This section is all the code generated by the VS.NET Forms Designer.
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources =
new System.Resources.ResourceManager(typeof(Form1));
this.pictureBox1 = new System.Windows.Forms.PictureBox();
this.myTimer = new System.Windows.Forms.Timer(this.components);
this.SuspendLayout();
this.pictureBox1.Name = "pictureBox1";
this.pictureBox1.Size = new System.Drawing.Size(304, 304);
this.pictureBox1.TabIndex = 0;
this.pictureBox1.TabStop = false;
this.myTimer.Enabled = true;
this.myTimer.Interval = 500;
this.myTimer.Tick += new System.EventHandler(this.myTimer_Tick);
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(300, 300);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.pictureBox1});
this.FormBorderStyle =
System.Windows.Forms.FormBorderStyle.FixedSingle;
this.Icon =
((System.Drawing.Icon)(resources.GetObject("$this.Icon")));
this.MaximizeBox = false;
this.Name = "Form1";
this.ShowInTaskbar = false;
this.Text = "Clock";
this.ResumeLayout(false);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void myTimer_Tick(object sender, System.EventArgs e)
{
TimeZone myTime = TimeZone.CurrentTimeZone;
TimeSpan myTimeSpan =
myTime.GetUtcOffset(new DateTime(DateTime.Today.Year,
DateTime.Today.Month, DateTime.Today.Day));
DateTime today = DateTime.UtcNow;
today = today.Add(myTimeSpan);
hour = today.Hour;
minute = today.Minute;
second = today.Second;
this.Text = "Clock " + hour + ":" + minute + ":" + second;
updateClock();
}
private void updateClock()
{
double angle=0;
double hourSize=.65, minuteSize=.85, secondSize=.8;
System.Drawing.Pen pen = new System.Drawing.Pen(Color.Black, 4);
Bitmap bitmaptemp = (Bitmap) this.bitmap.Clone();
Graphics gr = Graphics.FromImage(bitmaptemp);
gr.SmoothingMode =
System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
Point point1 = new Point(radius, radius), point2;
if (hour <= 12)
angle = ((Math.PI / 2) - ((double)hour/12)* 2 * Math.PI);
else if (hour > 12)
angle = ((Math.PI / 2) - ((double)(hour-12)/12)* 2 * Math.PI);
xCordinate = radius + (int)(radius * Math.Cos(angle) * hourSize);
yCordinate = radius - (int)(radius * Math.Sin(angle) * hourSize);
point2 = new Point(xCordinate, yCordinate);
gr.DrawLine(pen, point1, point2);
angle = ((Math.PI / 2) - ((double)minute/60) * 2 * Math.PI);
pen.Width = 2;
xCordinate = radius + (int)(radius * Math.Cos(angle) * minuteSize);
yCordinate = radius - (int)(radius * Math.Sin(angle) * minuteSize);
point2 = new Point(xCordinate, yCordinate);
gr.DrawLine(pen, point1, point2);
angle = ((Math.PI / 2) - (((double)second/60) * 2 * Math.PI));
pen.Width = 1;
xCordinate = radius + (int)(radius * Math.Cos(angle) * secondSize);
yCordinate = radius - (int)(radius * Math.Sin(angle) * secondSize);
point2 = new Point(xCordinate, yCordinate);
gr.DrawLine(pen, point1, point2);
this.pictureBox1.Image = bitmaptemp;
pen.Dispose();
gr.Dispose();
}
}
}
Above, I have a chunk of comments trying to explain what happens with the Math part. I hope I made sense!
Started programming when I was 8 using BASIC under DOS. Later I switched to C and then C++. Recently I have been doing PHP and just started with C#.