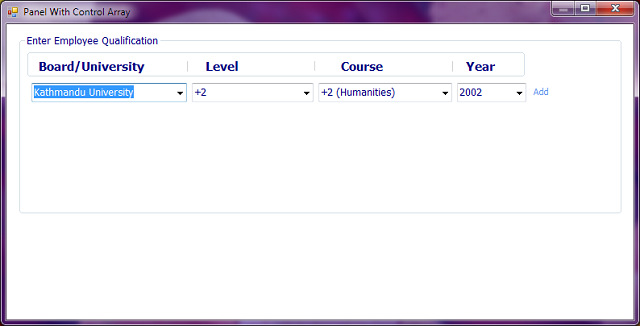
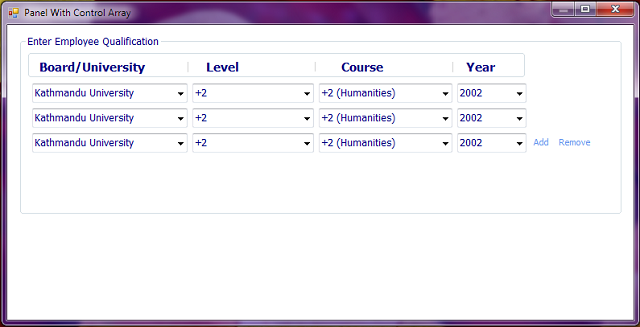
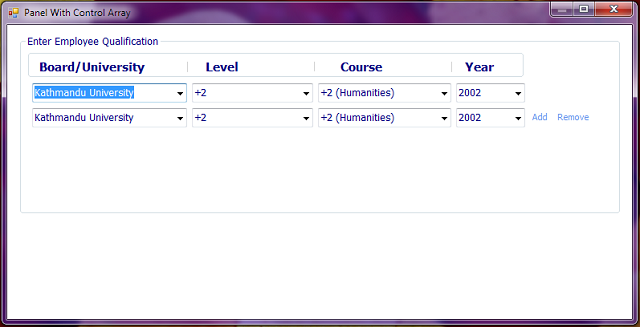
Introduction
This article is about adding and removing the array controls from one array control.
Background
The previous article was about creating the array control. This article includes adding and removing the array controls.
This also helps to change the appearance of one array control from another array control.
Using the Code
This code is done under the C# 4.0 framework.
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
Data_Connection DC = new Data_Connection();
private System.Windows.Forms.ComboBox[] Cbo_board_Array;
private System.Windows.Forms.ComboBox[] Cbo_board_level_Array;
private System.Windows.Forms.ComboBox[] Cbo_board_course_Array;
private System.Windows.Forms.ComboBox[] Cbo_board_year_Array;
private System.Windows.Forms.Label[] Lbl_add_array;
private System.Windows.Forms.Label[] Lbl_remove_array;
private int Draw_count = 0;
private void AddControls(string AnyCtrl, int CtrlNum)
{
case "ComboBoxBoardCtrl":
{
Cbo_board_Array = new System.Windows.Forms.ComboBox[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Cbo_board_Array[Draw_count] = new System.Windows.Forms.ComboBox();
}
break;
}
case "ComboBoxBoardLevelCtrl":
{
Cbo_board_level_Array =
new System.Windows.Forms.ComboBox[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Cbo_board_level_Array[Draw_count] =
new System.Windows.Forms.ComboBox();
}
break;
}
case "ComboBoxBoardCourseCtrl":
{
Cbo_board_course_Array =
new System.Windows.Forms.ComboBox[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Cbo_board_course_Array[Draw_count] =
new System.Windows.Forms.ComboBox();
}
break;
}
case "ComboBoxBoardYearCtrl":
{
Cbo_board_year_Array = new System.Windows.Forms.ComboBox[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Cbo_board_year_Array[Draw_count] =
new System.Windows.Forms.ComboBox();
}
break;
}
case "LabelAdd":
{
Lbl_add_array = new System.Windows.Forms.Label[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Lbl_add_array[Draw_count] = new System.Windows.Forms.Label();
}
break;
}
case "LabelRemove":
{
Lbl_remove_array = new System.Windows.Forms.Label[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
Lbl_remove_array[Draw_count] =
new System.Windows.Forms.Label();
}
break;
}
}
private void Form1_Load(object sender, EventArgs e)
{
int num = 1;
Draw_Combobox_Board(num);
}
private void Draw_Combobox_Board(int num)
{
AddControls("ComboBoxBoardCtrl", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Cbo_board_Array[i].Location =
new Point(5, (5 + (26 + 5) * i));
Cbo_board_Array[i].Size = new Size(193, 26);
Cbo_board_Array[i].Tag = i;
DataTable DT = DC.Get_Board();
Cbo_board_Array[i].DataSource = DT;
Cbo_board_Array[i].DisplayMember = "BOARD";
Cbo_board_Array[i].ForeColor = Color.Navy;
panel1.Controls.Add(Cbo_board_Array[i]);
i++;
}
Draw_Combobox_Board_Level(num);
}
private void Draw_Combobox_Board_Level(int num)
{
AddControls("ComboBoxBoardLevelCtrl", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Cbo_board_level_Array[i].Location =
new Point(204, (5 + (26 + 5) * i));
Cbo_board_level_Array[i].Size = new Size(151, 26);
Cbo_board_level_Array[i].Tag = i;
DataTable DT = DC.Get_Board_Level();
Cbo_board_level_Array[i].DataSource = DT;
Cbo_board_level_Array[i].DisplayMember = "BOARDLEVEL";
Cbo_board_level_Array[i].ForeColor = Color.Navy;
panel1.Controls.Add(Cbo_board_level_Array[i]);
i++;
}
Draw_Combox_Board_Course(num);
}
private void Draw_Combox_Board_Course(int num)
{
AddControls("ComboBoxBoardCourseCtrl", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Cbo_board_course_Array[i].Location =
new Point(361, (5 + (26 + 5) * i));
Cbo_board_course_Array[i].Size = new Size(166, 26);
Cbo_board_course_Array[i].Tag = i;
DataTable DT = DC.Get_Board_Course();
Cbo_board_course_Array[i].DataSource = DT;
Cbo_board_course_Array[i].DisplayMember = "BOARDCOURSE";
Cbo_board_course_Array[i].ForeColor = Color.Navy;
panel1.Controls.Add(Cbo_board_course_Array[i]);
i++;
}
Draw_Combox_Board_Year(num);
}
private void Draw_Combox_Board_Year(int num)
{
AddControls("ComboBoxBoardYearCtrl", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Cbo_board_year_Array[i].Location =
new Point(533, (5 + (26 + 5) * i));
Cbo_board_year_Array[i].Size = new Size(86, 26);
Cbo_board_year_Array[i].Tag = i;
DataTable DT = DC.Get_Board_Year();
Cbo_board_year_Array[i].DataSource = DT;
Cbo_board_year_Array[i].DisplayMember = "YEAR";
Cbo_board_year_Array[i].ForeColor = Color.Navy;
panel1.Controls.Add(Cbo_board_year_Array[i]);
i++;
}
Draw_Label_Add(num);
}
private void Draw_Label_Add(int num)
{
AddControls("LabelAdd", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Lbl_add_array[i].Location =
new Point(625, (9 + (26 + 5) * i));
Lbl_add_array[i].AutoSize = true;
Lbl_add_array[i].Tag = i;
Lbl_add_array[i].Font = new System.Drawing.Font
("Tahoma", 8.25F, System.Drawing.FontStyle.Regular,
System.Drawing.GraphicsUnit.Point, ((byte)(0)));
Lbl_add_array[i].Cursor = System.Windows.Forms.Cursors.Hand;
Lbl_add_array[i].Text = "Add";
Lbl_add_array[i].ForeColor = Color.CornflowerBlue;
if (num - i == 1)
{
Lbl_add_array[i].Visible = true;
}
else
{
Lbl_add_array[i].Visible = false;
}
Lbl_add_array[i].Click +=
new EventHandler(Lbl_Add_Array_Click);
panel1.Controls.Add(Lbl_add_array[i]);
i++;
}
Draw_Label_Remove(num);
}
private void Draw_Label_Remove(int num)
{
AddControls("LabelRemove", num);
Draw_count = num - 1;
for (int i = 0; i < num; )
{
Lbl_remove_array[i].Location =
new Point(657, (9 + (26 + 5) * i));
Lbl_remove_array[i].AutoSize = true;
Lbl_remove_array[i].Tag = i;
Lbl_remove_array[i].Font=new System.Drawing.Font
("Tahoma", 8.25F, System.Drawing.FontStyle.Regular,
System.Drawing.GraphicsUnit.Point, ((byte)(0)));
Lbl_remove_array[i].Cursor =
System.Windows.Forms.Cursors.Hand;
Lbl_remove_array[i].Text = "Remove";
Lbl_remove_array[i].ForeColor = Color.CornflowerBlue;
if (num - i == 1)
{
if (i == 0)
{
Lbl_remove_array[i].Visible = false;
}
else
{
Lbl_remove_array[i].Visible = true;
}
}
else
{
Lbl_remove_array[i].Visible = false;
}
Lbl_remove_array[i].Click +=
new EventHandler(Lbl_Remove_Array_Click);
panel1.Controls.Add(Lbl_remove_array[i]);
i++;
}
}
int count = 1;
private void Lbl_Add_Array_Click(object sender, System.EventArgs e)
{
count++;
int Array_Tag = (int)((System.Windows.Forms.Label)sender).Tag;
panel1.Controls.Clear();
Draw_Combobox_Board(count);
}
private void Lbl_Remove_Array_Click(object sender, System.EventArgs e)
{
int Array_Tag = (int)((System.Windows.Forms.Label)sender).Tag;
count = Array_Tag;
panel1.Controls.Remove(Cbo_board_Array[Array_Tag]);
panel1.Controls.Remove(Cbo_board_course_Array[Array_Tag]);
panel1.Controls.Remove(Cbo_board_level_Array[Array_Tag]);
panel1.Controls.Remove(Cbo_board_year_Array[Array_Tag]);
panel1.Controls.Remove(Lbl_add_array[Array_Tag]);
panel1.Controls.Remove(Lbl_remove_array[Array_Tag]);
if (Array_Tag - 1 > 0)
{
Lbl_add_array[Array_Tag - 1].Visible = true;
Lbl_remove_array[Array_Tag - 1].Visible = true;
}
else
{
Lbl_add_array[Array_Tag - 1].Visible = true;
Lbl_remove_array[Array_Tag - 1].Visible = false;
}
}
}
The above code is the complete code of the array controls.
You can add and remove the controls from one array control.
Points of Interest
You can edit the code for different purposes just like keeping the reference of the array controls from different array controls.
Giving the tag number is very important in this section. Without the tag of the control, you cannot define the type of control selected.
Thank you,
Santosh Shrestha
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.