Introduction
This post describes how to create controls in an array.
Background
You can now create the defined number of controls in an array according to the user input or as desired.
Using the Code
Here, you can find sample code to create the number of controls in an array.
As the user inputs the value and clicks the button to create controls, the controls are created as defined.
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private System.Windows.Forms.TextBox[] TextBoxArray;
private System.Windows.Forms.Label[] LabelArray;
private int Draw_count = 0;
private void AddControls(string AnyCtrl, int CtrlNum)
{
switch (AnyCtrl)
{
case "LabelCtrl":
{
LabelArray = new System.Windows.Forms.Label[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
LabelArray[Draw_count] =
new System.Windows.Forms.Label();
}
break;
}
case "TextBoxCtrl":
{
TextBoxArray =
new System.Windows.Forms.TextBox[CtrlNum];
for (Draw_count = 0; Draw_count < CtrlNum; Draw_count++)
{
TextBoxArray[Draw_count] =
new System.Windows.Forms.TextBox();
}
break;
}
}
private void button1_Click(object sender, EventArgs e)
{
if (maskedTextBox1.Text == string.Empty)
{
MessageBox.Show("Please, enter the value.",
"Error message", MessageBoxButtons.OK,
MessageBoxIcon.Error);
maskedTextBox1.Focus();
}
else
{
this.panel1.Controls.Clear();
AddControls("TextBoxCtrl", Convert.ToInt32(maskedTextBox1.Text));
Draw_count = Convert.ToInt32(maskedTextBox1.Text);
{
for (int i = 0; i < Draw_count; )
{
TextBoxArray[i].Location =
new Point(5, (5 + (22 + 5) * i));
TextBoxArray[i].Size = new Size(150, 22);
TextBoxArray[i].Text = "Textbox " + (i + 1).ToString();
TextBoxArray[i].ForeColor = Color.Navy;
this.panel1.Controls.Add
(TextBoxArray[i]);
i++;
}
}
}
}
private void button2_Click(object sender, EventArgs e)
{
if (maskedTextBox2.Text == string.Empty)
{
MessageBox.Show("Please, enter the value.",
"Error message", MessageBoxButtons.OK,
MessageBoxIcon.Error);
maskedTextBox2.Focus();
}
else
{
this.panel2.Controls.Clear();
AddControls("LabelCtrl", Convert.ToInt32(maskedTextBox2.Text));
Draw_count = Convert.ToInt32(maskedTextBox2.Text);
for (int j = 0; j < Draw_count; )
{
LabelArray[j].Location =
new Point(5, (5 + (22 + 5) * j));
LabelArray[j].Size = new Size(150, 22);
LabelArray[j].Text = "Label " + (j + 1).ToString();
LabelArray[j].ForeColor = Color.Navy;
this.panel2.Controls.Add(LabelArray[j]);
j++;
}
}
}
}
}
- Enter the number to create
textbox
or label
.
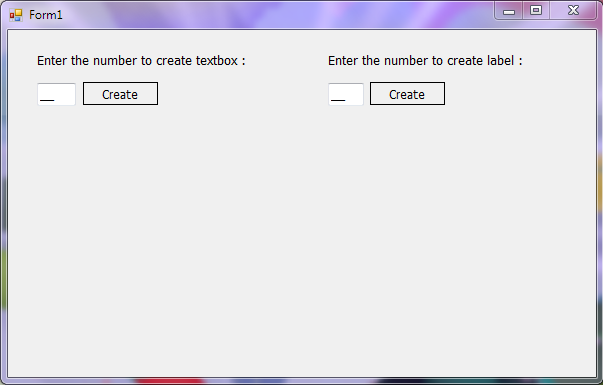
- Click the create button to create
textbox
or label
.
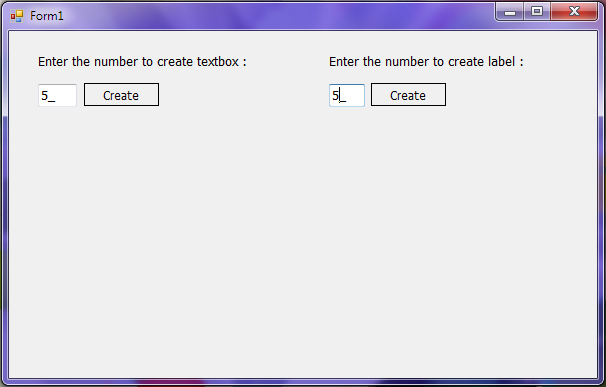
- You can see the list of array controls created.
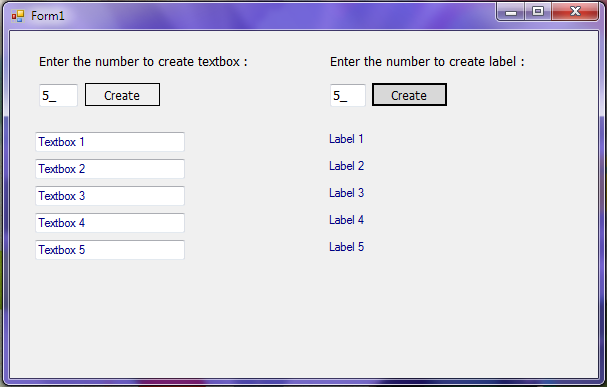
Thank you.
Santosh Shrestha
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.