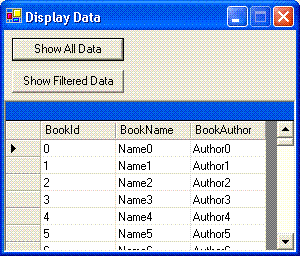
Introduction
This article shows us how to convert a Collection Base to a DataSet
. It uses the System.Reflection
and System.Collection
namespaces.
Background
Sometimes we need to convert a Collection Base to a DataSet
and we need to use the DataSet
's functionality such as filtering, sorting and others which are not available in a Collection Base. That's why I created this code. I hope it will help other developers.
Using the code
First, you need to create a class that inherits from CollectionBaseCustom
. CollectionBaseCustom
is inherited from System.Collection.CollectionBase
. You will find it in the source code available above.
using System;
[Serializable]
public class Book
{
public Book()
{
}
private string _BookId;
public string BookId
{
get
{
return _BookId;
}
set
{
_BookId = value;
}
}
private string _BookName;
public string BookName
{
get
{
return _BookName;
}
set
{
_BookName = value;
}
}
private string _BookAuthor;
public string BookAuthor
{
get
{
return _BookAuthor;
}
set
{
_BookAuthor = value;
}
}
}
public class BookCollection:CollectionBaseCustom
{
public void Add(Book oBook)
{
this.List.Add(oBook);
}
public Book this[Int32 Index]
{
get
{
return (Book)this.List[Index];
}
set
{
if (!(value.GetType().Equals(typeof(Book))))
{
throw new Exception("Type can't be converted");
}
this.List[Index] = value;
}
}
}
Now you fill the collection with data:
private BookCollection FillData()
{
BookCollection oBookCollection = new BookCollection();
for(int i = 0; i < 100; i++)
{
Book oBook = new Book();
oBook.BookId = i.ToString();
oBook.BookName = "Name" + i.ToString();
oBook.BookAuthor = "Author" + i.ToString();
oBookCollection.Add(oBook);
}
return oBookCollection;
}
When you need to convert a Collection Base to a DataSet
and bind it to a DataGrid
, you just write this code:
this.grdForm.DataSource = FillData().ToDataSet().Tables[0].DefaultView;
After that, you can use all of the DataSet
's functionality, such as filtering, just like this! Easy, huh?
if (this.grdForm.DataSource != null)
{
DataView oDataView = (DataView)this.grdForm.DataSource;
oDataView.RowFilter = "BookId > 22 AND BookId < 88 ";
this.grdForm.DataSource = oDataView.Table.DefaultView;
}
History
- 3 May, 2005 -- Original version posted
- 10 May, 2007 -- Updated
- 27 May, 2005 -- Article moved
- 15 June, 2007 -- Demo project download updated
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.