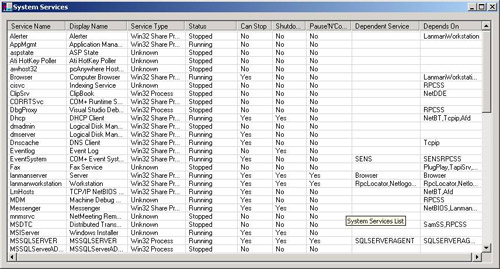
Introduction
Continuing in the series of writing some diagnostics tools and exploring the
System.Diagnostics
namespace; this article shows how do you find out what all system
services are running on the system and most importantly finding their dependencies. It's the later
part that I found very interesting. Every time I open the Services dialog box or Task Manager on my
system to look what all services are running, I used to think what will happen if I shut down one of
them to recover some resources. But then you have to think, what if this service is part of some
other service or application running on the system. This utility application enumerates and displays
all the system services running on a system. And it also lists all the services a particular service
is dependent upon and the services that depend on this service.
How do you do it?
The .NET framework provides the System.ServiceProcess
namespace. This namespace
contains classes for installing and running system services. In this namespace, you will find a
class named SystemController
. A SystemController
is nothing but a System
Service in NT lingo. This class has a static method GetServices()
that returns an
array of SystemController
objects. This array contains all the services running on a
system.
ServiceController [] controllers =
ServiceController.GetServices();
After you have obtained this array, enumerate through each element to get details about each
service. The SystemController
class has a bunch of properties and their names are
self-explanatory. I will not go into details of each property. I would suggest you to look in
framework documentation for more details.
DisplayName
- The descriptive name shown for this service in the Service
applet. Status
- Status of the service e.g., Running, Stopped, etc. ServiceType
- Type of service that object references. ServiceName
Short name of the service. CanStop
- Indicates if the service can be stopped at any time. CanShutDown
- Indicates if the service can be shut down any time. CanPauseAndContinue
- If the service can be stopped and continued. DependentServices
- List of services that depend on this service. ServicesDependOn
- List of services that this service depends on.
The ServiceController
class has a couple of methods that can be used to start and
stop a service. I have not shown the use of these methods in this utility project. But they are
pretty simple to use.
Look at the InitList
method in the source code attached with this article. This
function shows how to use all the properties of the SystemController
class.
bool bCanStop = controllers[i].CanStop;
item.SetSubItem(3, bCanStop ? "Yes" : "No");
bool bCanShutDown = controllers[i].CanShutdown;
item.SetSubItem(4, bCanShutDown ? "Yes" : "No");
bool bPauseNContinue = controllers[i].CanPauseAndContinue;
item.SetSubItem(5, bPauseNContinue ? "Yes" : "No");
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.