Introduction
In this article, I will provide code sample on how to make a simple Master-Slave DataGrid
. First, add a DataGrid
on the page and make a "Select" column using property builder.
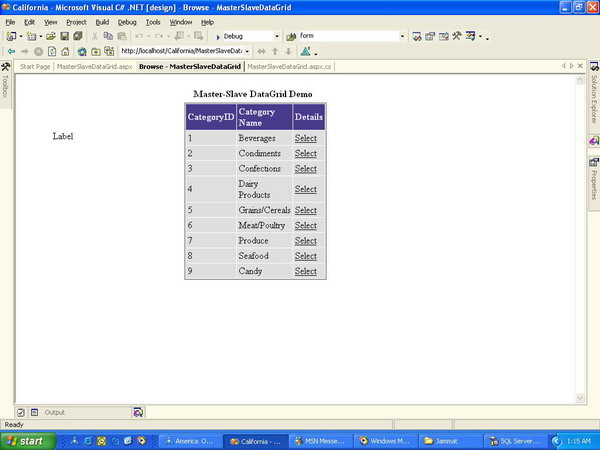

Master-Slave DataGrid in C# Code
private void Page_Load(object sender, System.EventArgs e)
{
if(!Page.IsPostBack)
{
BindGrid();
}
}
public void BindGrid()
{
SqlCommand myCommand =
new SqlCommand("SELECT * FROM Categories",myConnection);
myCommand.CommandType = CommandType.Text;
try
{
myConnection.Open();
SqlDataReader dr =
myCommand.ExecuteReader(CommandBehavior.CloseConnection);
MasterGrid.DataSource = dr;
MasterGrid.DataBind();
}
catch ( SqlException SqlEx )
{
}
catch ( Exception ex )
{
}
finally { myConnection.Close(); }
}
private void ViewSelectionDetails(object sender, System.EventArgs e)
{
Int32 categoryID =
Convert.ToInt32(MasterGrid.SelectedItem.Cells[0].Text);
Label2.Text = categoryID.ToString();
PopulateSlaveGrid( categoryID );
}
public void PopulateSlaveGrid(Int32 cID)
{
string commandString =
"SELECT CategoryName,Description FROM Categories WHERE CategoryID="+cID;
SqlDataAdapter ad = new SqlDataAdapter(commandString,myConnection);
DataSet ds = new DataSet();
ad.Fill(ds,"Categories");
try
{
myConnection.Open();
SlaveGrid.DataSource = ds;
SlaveGrid.DataBind();
}
catch ( SqlException SqlEx )
{
}
catch ( Exception ex )
{
}
finally { myConnection.Close(); }
}
}
My name is Mohammad Azam and I have been developing iOS applications since 2010. I have worked as a lead mobile developer for VALIC, AIG, Schlumberger, Baker Hughes, Blinds.com and The Home Depot. I have also published tons of my own apps to the App Store and even got featured by Apple for my app, Vegetable Tree. I highly recommend that you check out my portfolio. At present I am working as a lead instructor at DigitalCrafts.
I also have a lot of Udemy courses which you can check out at the following link:
Mohammad Azam Udemy Courses