Introduction
XML is a very important base on which Web Services work. XML can be used in conjunction with a lot of client side and server side languages to put it to good effect. Let us see how we can use XML and client side JavaScript to work. We will see how we can display the contents of an XML file using JavaScript, accessing child elements, manipulating elements etc.
Browser issues
When it comes to client side languages, browser incompatibilities are a major issue. But here where we want to use XML and JavaScript, XML is the issue. Not all browsers have support for parsing XML documents. I will use IE6 to explain the codes. Browsers that do not support XML, cannot read them. When you view an XML file in such a browser, it will just ignore all the tags.
Sample XML file
Let us consider a sample XML file >>
="1.0"
<company>
<employee id="001" sex="M" age="19">Premshree Pillai</employee>
<employee id="002" sex="M" age="24">Kumar Singh</employee>
<employee id="003" sex="F" age="21">Suhasini Pandita</employee>
<turnover>
<year id="2000">100,000</year>
<year id="2001">140,000</year>
<year id="2002">200,000</year>
</turnover>
</company>
The above XML file shows employee data and turnover of the company (just an example).
Manipulating XML file data using JavaScript
- Loading XML file: You can load an XML fie from JavaScript like this >>
var xmlDoc = new ActiveXObject("Microsoft.XMLDOM");
function loadXML(xmlFile)
{
xmlDoc.async="false";
xmlDoc.onreadystatechange=verify;
xmlDoc.load(xmlFile);
xmlObj=xmlDoc.documentElement;
}
Actually, just the last two lines of the function are enough to load the XML file. The previous two lines are written to ensure that the JavaScript functions that we may use later to manipulate the XML file data, does not perform any function on an uninitialized object. Thus the function verify()
is called.
function verify()
{
if (xmlDoc.readyState != 4)
{
return false;
}
}
Now the XML file can be loaded.
loadXML('xml_file.xml');
- Displaying contents of the XML file: View the entire contents of the XML file using:
alert(xmlObj.xml);
The entire XML file will be displayed in an alert box as it is with proper indentation.
- Children and nodes: In the above XML file
<company>
is the top level tag under which all other tags come. These tags are called children. The above XML file can be represented graphically like a folder-tree. A folder-tree is shown below.
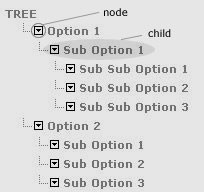
Thus in the above XML file the top level tag <company>
has 4 children. The numbering of children (as is usual in all languages) starts from 0 (zero). The <turnover>
tag has 3 children under it.
We can find the number of children a tag has by using the childNodes.length
property. Thus the number of children of <company>
tag (here, 4) can be found by using xmlObj.childNodes.length
.
The number of children of <turnover>
tag (here, 3) can be found by using xmlObj.childNodes(3).childNodes.length
.
Here we use childNodes(3)
because <turnover>
is the 3rd child of <company>
- Testing for children: You can test whether a particular node child has any children using
childNodes(i).hasChildNodes
. Thus xmlObj.childNodes(3).hasChildNodes()
will return true
. xmlObj.childNodes(2).hasChildNodes()
will return false
, since the <employee>
tag does not have any children.
- Getting tag Name: You can get the tag name of a child using
childNodes(i).tagName
. Thus xmlObj.tagName
will return "company
". xmlObj.childNodes(0).tagName
will return "employee
". xmlObj.childNodes(3).childNodes(0).tagName
will return "year
".
- Displaying content of a tag: In the XML file, the content of the 1st
<employee>
tag is "Premshree Pillai
". You can get this value using xmlObj.childNodes(0).firstChild.text
. xmlObj.childNodes(2).firstChild.text
will return “Suhasini Pandita
”. Similarly xmlObj.childNodes(3).childNodes(1).firstChild.text
will return "140,000
".
- Attributes: In the XML file, the
<employee>
tag has 3 attributes. An attribute can be accessed using childNodes(i).getAttribute(“AttributeName”)
. Thus xmlObj.childNodes(0).getAttribute("id")
will return "001
". xmlObj.childNodes(1).getAttribute("age")
will return "24
". xmlObj.childNodes(2).getAttribute("sex")
will return "F
".
XML – JavaScript example
There are many more properties and methods available. Using these properties you can create lots of client side applications. The main advantage of using XML along with JavaScript is that editing of data becomes very easy. XML being structured, it becomes very easy to manage content. One example is a folder-tree menu. Another one is a JavaScript Ticker. You can find an XML based JavaScript Ticker here.
XML based JavaScript Ticker
We will create an XML based JavaScript Ticker that can tick any number of messages. The ticker reads its contents, i.e. the ticker style, text to be displayed, the link for that particular message from an XML file. Let the XML file be ticker_items.xml
The structure of the XML document is as follows >>

XML Ticker Script
<script language="JavaScript1.2">
var xmlDoc = new ActiveXObject("Microsoft.XMLDOM");
function loadXML(xmlFile)
{
xmlDoc.async="false";
xmlDoc.onreadystatechange=verify;
xmlDoc.load(xmlFile);
ticker=xmlDoc.documentElement;
}
function verify()
{
if (xmlDoc.readyState != 4)
{
return false;
}
}
loadXML('ticker_items.xml');
document.write('<style type="text\/css">');
document.write('.ticker_style{font-family:' +
ticker.childNodes(1).childNodes(0).getAttribute('font') + '; font-size:' +
ticker.childNodes(1).childNodes(0).getAttribute('size') + '; color:' +
ticker.childNodes(1).childNodes(0).getAttribute('color')
+ '; font-weight:' +
ticker.childNodes(1).childNodes(0).getAttribute('weight') +
'; text-decoration:' +
ticker.childNodes(1).childNodes(0).getAttribute('decoration') + '}');
document.write('.ticker_style:hover{font-family:' +
ticker.childNodes(1).childNodes(1).getAttribute('font') + '; font-size:' +
ticker.childNodes(1).childNodes(1).getAttribute('size') + '; color:' +
ticker.childNodes(1).childNodes(1).getAttribute('color')
+ '; font-weight:' +
ticker.childNodes(1).childNodes(1).getAttribute('weight') +
'; text-decoration:' +
ticker.childNodes(1).childNodes(1).getAttribute('decoration') + '}<br>');
document.write('</style>');
document.write('<table style="border:' +
ticker.childNodes(0).getAttribute('border') +
' solid ' + ticker.childNodes(0).getAttribute('bordercolor') +
'; background:' +
ticker.childNodes(0).getAttribute('background') + '; width:' +
ticker.childNodes(0).getAttribute('width') + '; height:' +
ticker.childNodes(0).getAttribute('height') + '"><tr><td><div
id="ticker_space"></div></td></tr></table>');
var item_count=2;
var timeOutVal=(ticker.childNodes(0).getAttribute('timeout'))*1000;
var original_timeOutVal=timeOutVal;
var isPauseContent;
if(ticker.childNodes(0).getAttribute('pause')=="true")
{
isPauseContent=' onmouseover="setDelay();" onmouseout="reset();"';
}
else
{
isPauseContent='';
}
function setTicker()
{
document.all.ticker_space.innerHTML='<center><a href="' +
ticker.childNodes(item_count).getAttribute('URL') + '" target="' +
ticker.childNodes(item_count).getAttribute('target') +
'" class="ticker_style"' + isPauseContent + '>' +
ticker.childNodes(item_count).firstChild.text + '</a></center>';
if(item_count==ticker.childNodes.length-1)
{
item_count=2;
}
else
{
item_count++;
}
setTimeout("setTicker()",timeOutVal);
}
function setDelay()
{
timeOutVal=10000000000000;
item_count--;
}
function reset()
{
timeOutVal=original_timeOutVal;
setTicker();
}
setTicker();
</script>
As you can see in the source code, the ticker reads all the contents/messages to be displayed, the links for each message, the target for each URL, the ticker static style, roll-over style, border width, color, background, the delay between messages etc. from the XML file. So if you want to change any parameter of the Ticker, all you have to do is make necessary changes in the XML file.
The ticker shown here is a basic ticker that rotates messages at an interval that is specified in the XML file. There are many effects you could add to the ticker like ‘Fading message’, ‘Teletypewriter’ etc. You could add features to change the ticker speed or to list all messages at an instant.
You can find some Ticker effects here.
I hope this article has helped you in some way.
The author is an engineering student in Mumbai, India and is a programming enthusiast. You can visit his site at http://www.qiksearch.com