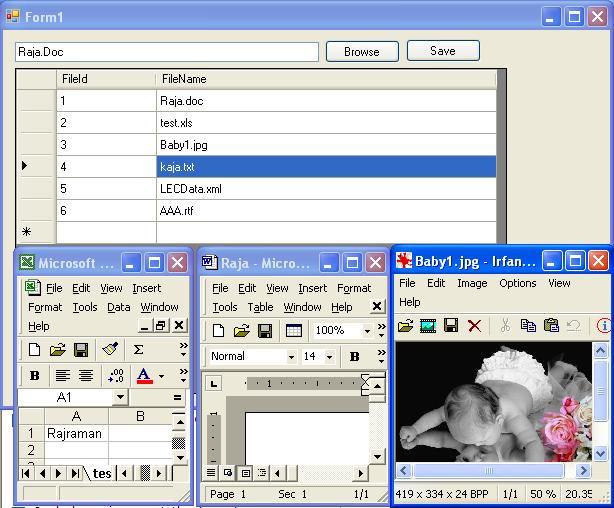
Introduction
In this code I used stream objects for converting the documents and images into byte objects. These byte objects will be stored in the database table in the image field.
How to convert images and documents into byte objects in C#
Here is the simple code to convert these files into byte objects.
FileStream fileStream = new FileStream(fileName, FileMode.OpenOrCreate,
FileAccess.Read);
long len;
len = fileStream.Length;
Byte[] fileAsByte = new Byte[len];
fileStream.Read(fileAsByte, 0, fileAsByte.Length);
MemoryStream memoryStream = new MemoryStream(fileAsByte);
return memoryStream.ToArray();
Here fileName
represents the name and location of the file. I used fileStream
objects to read the files and convert these files into byte objects using the fileStream.Read
command. I invoked memorystream
to convert the bytes into byteArray
.
How to convert the byte objects into words and images from C#
FileStream fs = new FileStream(di + "\\" + fileName, FileMode.Create);
fs.Write(content, 0, System.Convert.ToInt32(content.Length));
fs.Seek(0, SeekOrigin.Begin);
fs.Close();
The above code explains how the images/documents are converted to byte objects.
How to Store these files into a Database
SqlCommand insert = new SqlCommand("insert into FileStore
_([FileName],[FileStream]) values (@FileName,@FileStream)",
_sqlConnection);
SqlParameter imageParameter = insert.Parameters.Add("@FileStream",
_SqlDbType.Binary);
SqlParameter fileNameParameter = insert.Parameters.Add("@FileName",
_SqlDbType.VarChar);
imageParameter.Value = content;
imageParameter.Size = content.Length;
fileNameParameter.Value = fileName;
insert.ExecuteNonQuery();
The above code will explain how the content of the image/document is stored into the database.
Database Table Design:
- Table Name: FileStore
- Field:
FileId int - set its identity field.
fileName
Varchar(50)
FileStream
image
Summary:
The program was developed by C#.NET 2.0 and SQL Server 2005.