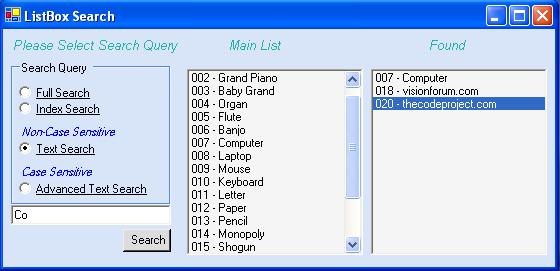
Introduction
This code shows you how to search through a ListBox (ListBox1) and add all Items that match the search query (from TextBox1) to a second ListBox (ListBox2).
Using The Source Code
I have included two sets of the source code, one with comments, and one without. I did this because the begginers have a better chance of needed comments to walk them through the steps, but they might just be more in the way than anything else for the more advanced programmers.
The Source Code
<FONT size=2><P></FONT><FONT color=#0000ff size=2>Private</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Sub</FONT><FONT size=2> btnSearch_Click(</FONT><FONT color=#0000ff size=2>ByVal</FONT><FONT size=2> sender </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> System.Object, </FONT><FONT color=#0000ff size=2>ByVal</FONT><FONT size=2> e </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> System.EventArgs) _</FONT></P><P><FONT color=#0000ff size=2> Handles</FONT><FONT size=2> btnSearch.Click</FONT><FONT size=2></P><P></FONT><FONT color=#008000 size=2> '//removes all items from the second List Box</P></FONT><FONT size=2><P> ListBox2.Items.Clear()</P><P></FONT><FONT color=#008000 size=2> '
Now without the comments.
<FONT size=2><P></FONT><FONT color=#0000ff size=2>Private</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Sub</FONT><FONT size=2> btnSearch_Click(</FONT><FONT color=#0000ff size=2>ByVal</FONT><FONT size=2> sender </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> System.Object, </FONT><FONT color=#0000ff size=2>ByVal</FONT><FONT size=2> e </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> System.EventArgs) _</FONT></P><P> <FONT color=#0000ff size=2>Handles</FONT><FONT size=2> btnSearch.Click</P><P> ListBox2.Items.Clear()</P><P></FONT><FONT color=#0000ff size=2> Dim</FONT><FONT size=2> listLength </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Integer</FONT><FONT size=2> = (ListBox1.Items.Count - 1)</P><P></FONT><FONT color=#0000ff size=2> Dim</FONT><FONT size=2> i, j </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Integer</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Dim</FONT><FONT size=2> listString, newString </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>String</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> radioFull.Checked = </FONT><FONT color=#0000ff size=2>True</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> i = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listLength</P><P> listString = ListBox1.Items.Item(i)</P><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> InStr(listString.ToLower, TextBox1.Text.ToLower) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> ListBox2.Items.Add(listString)</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> ElseIf</FONT><FONT size=2> radioIndex.Checked = </FONT><FONT color=#0000ff size=2>True</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> i = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listLength</P><P> listString = ListBox1.Items.Item(i)</P><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> j = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listString.Length - 1</P><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> listString.Substring(j, 1) <> Chr(32) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> newString += listString.Substring(j, 1)</P><P></FONT><FONT color=#0000ff size=2> Else</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> InStr(newString, TextBox1.Text) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> ListBox2.Items.Add(ListBox1.Items.Item(i))</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Exit</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>For</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P> newString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> ElseIf</FONT><FONT size=2> radioText.Checked = </FONT><FONT color=#0000ff size=2>True</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Dim</FONT><FONT size=2> spaceCharCounter </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Integer</FONT><FONT size=2> = 0</P><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> i = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listLength</P><P> listString = ListBox1.Items.Item(i)</P><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> j = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listString.Length - 1</P><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> spaceCharCounter >= 2 </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> newString += listString.Substring(j, 1)</P><P></FONT><FONT color=#0000ff size=2> ElseIf</FONT><FONT size=2> listString.Substring(j, 1) = Chr(32) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> spaceCharCounter += 1</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> InStr(newString.ToLower, TextBox1.Text.ToLower) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> ListBox2.Items.Add(ListBox1.Items.Item(i))</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P> listString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P> spaceCharCounter = 0</P><P> newString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> ElseIf</FONT><FONT size=2> radioAdvText.Checked = </FONT><FONT color=#0000ff size=2>True</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Dim</FONT><FONT size=2> spaceCharCounter </FONT><FONT color=#0000ff size=2>As</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Integer</FONT><FONT size=2> = 0</P><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> i = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listLength</P><P> listString = ListBox1.Items.Item(i)</P><P></FONT><FONT color=#0000ff size=2> For</FONT><FONT size=2> j = 0 </FONT><FONT color=#0000ff size=2>To</FONT><FONT size=2> listString.Length - 1</P><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> spaceCharCounter >= 2 </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> newString += listString.Substring(j, 1)</P><P></FONT><FONT color=#0000ff size=2> ElseIf</FONT><FONT size=2> listString.Substring(j, 1) = Chr(32) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> spaceCharCounter += 1</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> If</FONT><FONT size=2> InStr(newString, TextBox1.Text) </FONT><FONT color=#0000ff size=2>Then</P></FONT><FONT size=2><P> ListBox2.Items.Add(ListBox1.Items.Item(i))</P><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P> listString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P> spaceCharCounter = 0</P><P> newString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> Next</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2> End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>If</P></FONT><FONT size=2><P> listString = </FONT><FONT color=#0000ff size=2>Nothing</P></FONT><FONT size=2><P></FONT><FONT color=#0000ff size=2>End</FONT><FONT size=2> </FONT><FONT color=#0000ff size=2>Sub</FONT></P><FONT color=#0000ff></FONT>
The End
I would have seperated the different RadioButton.Checked statements for easier reading, but I'm having some problems with the editor.
Have Fun!
3+ years Visual Basic.NET programming experience. A little ASP.NET and HTML as well.