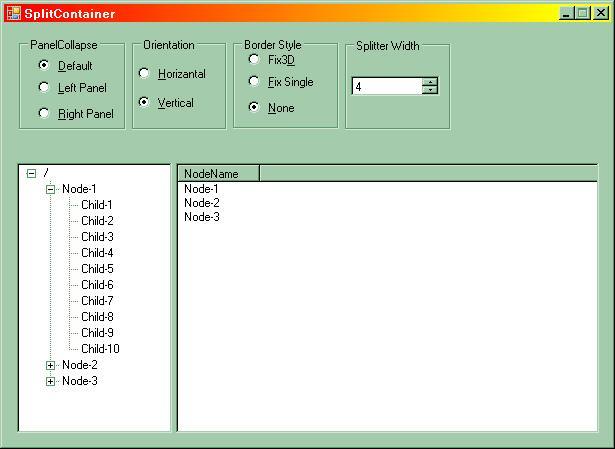
Assumption
This article assumed that you know litle about the TreView and ListView advance controll. Right now I have used Visual Studio 2005 Beta2, you can open it in Visual Studio 2003 by merging the partial classes and definately with .Net Framework 2.0.
Introduction
Windows based desktop interfaces can categorized into three types, 1) SDI-Single Document Interface like Microsoft Notepad,2) MDI-Multiple Document interface like Microsoft Word and 3)WE-Window Explorer Tree and ListView style interface. Designing a form like 'Window Explorer' is much easier now using the SplitContainer. I will demonstrate You through a simple example that how you can consume advance controll-'SplitContainer' into your application.
Description
SplitContainer
is an advance window's control or it is an extended version of old splitter control. You can place it at form horizantally or vertically by specifying Orientation property each represents left/top and right/bottom panels respectively.
Properties
1) Dock
Allows you to attach Splitcontainer's border to its container. When you drop the splitcontainer on a form a Default value of Dock property is 'FILL' that means splitcontainer gets the whole area of form. In this example, I have set it to 'None' for the sack of demonstrating splitcontainer features.
2) Panel1Collapsed / Panel2Collapsed
Used to set or get whether the panel1 or Panel2 is collapsed or expanded. If you assign true to Panel1Collapsed then left or top panel will be collapsed and if you assign false to Panel1Collapsed then left or top panel will be expanded same behaviour will be occured with Panel2Collapsed property.
Following are the lines of codes which toggles the collapse and expand behaviour
splitContainer1.Panel1Collapsed = true;
splitContainer1.Panel2Collapsed = false;
splitContainer1.Panel2Collapsed = true;
splitContainer1.Panel1Collapsed = false;
3) Orientation
Used to set or get the orientation of splitcontainer panel
splitContainer1.Orientation = Orientation.Horizontal;
splitContainer1.Orientation = Orientation.Vertical;
4) Border Style
Used to set or get the border style splitcontainer.
splitContainer1.BorderStyle = BorderStyle.Fixed3D;
splitContainer1.BorderStyle = BorderStyle.FixedSingle;
splitContainer1.BorderStyle = BorderStyle.None;
5) Splitter Width
Splitter is a horinzantal or vertical bar which use to set panel size, default size of splitter is 4 pixel but you can set or get the splitter width value programatically by manipulating splitterwidth property.
private void numericUpDown1_ValueChanged(object sender, EventArgs e)
{
NumericUpDown nm = (NumericUpDown)sender;
splitContainer1.SplitterWidth =(int) nm.Value ;
}
Events
1) SplitterMoving
This event occurs while the split control in moving state.
private void splitContainer1_SplitterMoving(object sender, SplitterCancelEventArgs e)
{
if(splitContainer1.Orientation == Orientation.Vertical )
Cursor.Current = Cursors.NoMoveVert;
else
Cursor.Current = Cursors.NoMoveHoriz;
}
2) SplitterMoved
This event occurs when the split control is moved.
private void splitContainer1_SplitterMoved(object sender, SplitterEventArgs e)
{
Cursor.Current = Cursors.Default;
}
Usage
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
namespace SplitContainerDemo
{
public partial class MySplitContainer : Form
{
public MySplitContainer()
{
InitializeComponent();
this.loadValuesInto();
}
private void loadValuesInto()
{
TreeNode tnRoot = treeView1.Nodes.Add("root", "/");
treeView1.Nodes[treeView1.Nodes.IndexOfKey("root")].Nodes.Add("Node-1", "Node-1");
TreeNode[] nodes = treeView1.Nodes.Find("Node-1", true);
addChildTo(nodes[0]);
treeView1.Nodes[treeView1.Nodes.IndexOfKey("root")].Nodes.Add("Node-2", "Node-2");
nodes = treeView1.Nodes.Find("Node-2", true);
addChildTo(nodes[0]);
treeView1.Nodes[treeView1.Nodes.IndexOfKey("root")].Nodes.Add("Node-3", "Node-3");
nodes = treeView1.Nodes.Find("Node-3", true);
addChildTo(nodes[0]);
}
private void addChildTo(TreeNode tn)
{
for (int i = 1; i <= 10; i++)
tn.Nodes.Add(tn.Name + ".Child-" + i, "Child-" + i);
}
private void rdoLeft_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.Panel1Collapsed = true;
splitContainer1.Panel2Collapsed = false;
}
private void rdoRight_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.Panel2Collapsed = true;
splitContainer1.Panel1Collapsed = false;
}
private void rdoDefault_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.Panel1Collapsed = false;
splitContainer1.Panel2Collapsed = false;
}
private void rdoHorizantal_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.Orientation = Orientation.Horizontal;
}
private void rdoVertical_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.Orientation = Orientation.Vertical;
}
private void rdoFix3d_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.BorderStyle = BorderStyle.Fixed3D;
}
private void rdoFixSingle_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.BorderStyle = BorderStyle.FixedSingle;
}
private void rdoNone_CheckedChanged(object sender, EventArgs e)
{
splitContainer1.BorderStyle = BorderStyle.None;
}
private void treeView1_AfterSelect(object sender, TreeViewEventArgs e)
{
listView1.Items.Clear();
for (int i = 0; i < e.Node.Nodes.Count; i++)
listView1.Items.Add(e.Node.Nodes[i].Name);
}
private void numericUpDown1_ValueChanged(object sender, EventArgs e)
{
NumericUpDown nm = (NumericUpDown)sender;
splitContainer1.SplitterWidth = (int)nm.Value;
}
private void splitContainer1_SplitterMoving(object sender, SplitterCancelEventArgs e)
{
if (splitContainer1.Orientation == Orientation.Vertical)
Cursor.Current = Cursors.NoMoveVert;
else
Cursor.Current = Cursors.NoMoveHoriz;
}
private void splitContainer1_SplitterMoved(object sender, SplitterEventArgs e)
{
Cursor.Current = Cursors.Default;
}
}
}
Conclusion
I hope this will give you overview about splitcontainer, I really appreciate any feedback, comments and suggestion.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.