Introduction
In this article, I will share the code for most common operations of a DataGrid
control. These operations include editing, updating, selecting, deleting, sorting and paging. Before you write any code, you need to add the columns into your DataGrid
. Please see the images below to see how to do that.
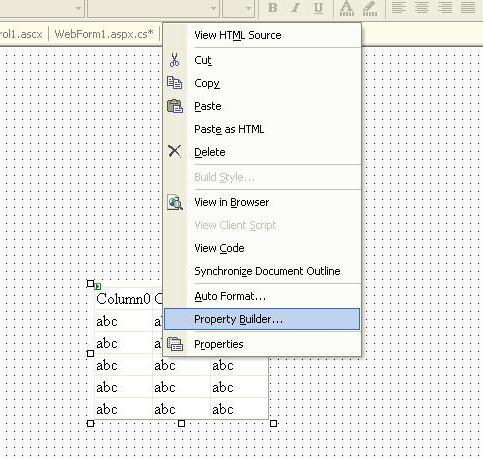

Now we are ready. So let's start with the Page_Load
event.
Page_Load Event Code
if(!Page.IsPostBack)
{
Bind_DataGrid();
}
Bind_DataGrid Method Code
public void Bind_DataGrid()
{
SqlCommand myCommand =
new SqlCommand("SELECT * FROM Categories",myConnection);
myCommand.CommandType = CommandType.Text;
SqlDataAdapter myAdapter = new SqlDataAdapter(myCommand);
DataSet ds = new DataSet();
myAdapter.Fill(ds,"Categories");
myConnection.Open();
myCommand.ExecuteReader();
myDataGrid.DataSource = ds;
myConnection.Close();
try
{
myDataGrid.DataBind();
}
catch
{
myDataGrid.CurrentPageIndex = 0;
Bind_DataGrid();
}
}
EditCommand Event Code
private void Edit_DataGrid(object source,
System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
myDataGrid.EditItemIndex = e.Item.ItemIndex;
Bind_DataGrid();
}
CancelCommand Event Code
private void Cancel_DataGrid(object source,
System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
myDataGrid.EditItemIndex = -1;
Bind_DataGrid();
}
UpdateCommand Event Code
private void Update_DataGrid(object source,
System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
System.Web.UI.WebControls.TextBox cName =
new System.Web.UI.WebControls.TextBox();
System.Web.UI.WebControls.TextBox cID =
new System.Web.UI.WebControls.TextBox();
cID = (System.Web.UI.WebControls.TextBox) e.Item.Cells[0].Controls[0];
cName = (System.Web.UI.WebControls.TextBox) e.Item.Cells[1].Controls[0];
Label3.Text = cID.Text;
SqlCommand myCommand = new SqlCommand("UpdateCat",myConnection);
myCommand.CommandType = CommandType.StoredProcedure;
myCommand.Parameters.Add(new SqlParameter("@CategoryID",
SqlDbType.NVarChar,4));
myCommand.Parameters["@CategoryID"].Value = Convert.ToInt32( cID.Text );
myCommand.Parameters.Add(new SqlParameter("@CategoryName",
SqlDbType.NVarChar,15));
myCommand.Parameters["@CategoryName"].Value = cName.Text;
myConnection.Open();
myCommand.ExecuteNonQuery();
myConnection.Close();
myDataGrid.EditItemIndex = -1;
Bind_DataGrid();
}
SelectedIndexChanged Event Code
private void Select_DataGrid(object sender, System.EventArgs e)
{
Label2.Text += myDataGrid.SelectedItem.Cells[0].Text;
}
PageIndexChanged Event Code
private void Paging_DataGrid(object source,
System.Web.UI.WebControls.DataGridPageChangedEventArgs e)
{
myDataGrid.CurrentPageIndex = e.NewPageIndex;
Bind_DataGrid();
}
SortCommand Event Code
The sorting is done by clicking on the column header. Clicking first time will sort the DataGrid
in the ascending order and clicking twice will sort the DataGrid
in descending order.
private void Sort_DataGrid(object source,
System.Web.UI.WebControls.DataGridSortCommandEventArgs e)
{
SqlCommand myCommand =
new SqlCommand("SELECT * FROM Categories",myConnection);
myCommand.CommandType = CommandType.Text;
SqlDataAdapter myAdapter = new SqlDataAdapter(myCommand);
DataSet ds = new DataSet();
myAdapter.Fill(ds,"Categories");
DataView dv = new DataView(ds.Tables["Categories"]);
if( (numberDiv%2) == 0 )
dv.Sort = e.SortExpression + " " + "ASC";
else
dv.Sort = e.SortExpression + " " + "DESC";
numberDiv++;
myDataGrid.DataSource = dv;
myDataGrid.DataBind();
}
DeleteCommand Event Code
private void Delete_DataGrid(object source,
System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
string s = e.Item.Cells[0].Text;
SqlCommand myCommand =
new SqlCommand("DELETE FROM Categories WHERE CategoryID="+s,myConnection);
myCommand.CommandType = CommandType.Text;
myConnection.Open();
myCommand.ExecuteNonQuery();
myConnection.Close();
Bind_DataGrid();
}
Enjoy the code! I hope you all will use it one way or the other.