This article shows how to use the AngularJS event calendar component to build a scheduling application. The calendar supports drag and drop, event editing using a modal dialog, and date picker for changing the visible date.
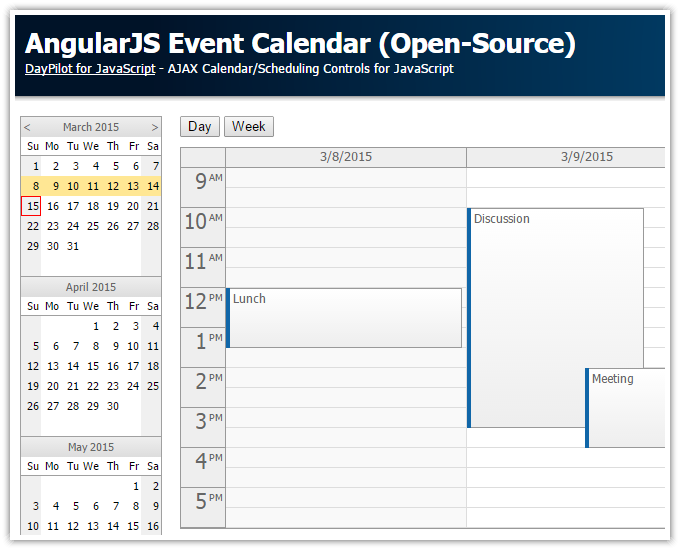
This article shows how to use the AngularJS event calendar (scheduler) from the open-source DayPilot Lite for JavaScript [javascript.daypilot.org
] library to build a calendar/scheduling web application.
- Drag and drop event creating
- Drag and drop event moving
- Drag and drop event resizing
- Event editing and deleting using a modal dialog
- Switching between day and week views
- Date navigator (date picker) for switching the visible day/week
- Automatic binding to a shared event data object using AngularJS
- AJAX notification calls to update the database (the server part is implemented in PHP and in ASP.NET MVC)
- Open-source (Apache License 2.0)
The server part is very light-weight and is based on simple JSON messages.
Angular Calendar/Scheduler

DayPilot calendar/scheduler component is now available for Angular as well. See the following tutorial to learn how to use it to build a Angular calendar with integrated day/week/month views:
AngularJS Event Calendar
DayPilot Lite for JavaScript lets you create the event calendar/scheduler UI using JavaScript. The latest release (version 1.2 [javascript.daypilot.org]) adds an AngularJS plugin.
The plugin provides element-based AngularJS directives that will let you create the calendar UI components easily:
<daypilot-calendar>
<daypilot-month>
<daypilot-navigator>
In this article, we will use the <daypilot-calendar>
and <daypilot-navigator>
directives to create a simple scheduling application with the following components:
- daily event calendar
- weekly event calendar
- date navigator
See also the AngularJS event calendar documentation [doc.daypilot.org
].
AngularJS Scheduler Day View

We will add the daily scheduler using <daypilot-calendar>
AngularJS element.
- The configuration is set using
dayConfig
object (daypilot-config="dayConfig"
) - The events are set using events object (
daypilot-events="events"
)
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script>
<script src="js/daypilot/daypilot-all.min.js" type="text/javascript"></script>
<div ng-app="main" ng-controller="DemoCtrl" >
<daypilot-calendar id="day"
daypilot-config="dayConfig" daypilot-events="events" ></daypilot-calendar>
</div>
<script type="text/javascript">
var app = angular.module('main',
['daypilot']).controller('DemoCtrl', function($scope, $timeout, $http) {
$scope.events = [];
$scope.dayConfig = {
viewType: "Day"
};
});
</script>
AngularJS Scheduler Week View

We will use another instance of the scheduler to create the week view. The configuration is very similar. The only difference is that we will keep this instance hidden (visible: false
).
<div ng-app="main" ng-controller="DemoCtrl" >
<daypilot-calendar id="day"
daypilot-config="dayConfig" daypilot-events="events" ></daypilot-calendar>
<daypilot-calendar id="week"
daypilot-config="weekConfig" daypilot-events="events" ></daypilot-calendar>
</div>
<script type="text/javascript">
var app = angular.module('main',
['daypilot']).controller('DemoCtrl', function($scope, $timeout, $http) {
$scope.events = [];
$scope.dayConfig = {
viewType: "Day"
};
$scope.weekConfig = {
visible: false,
viewType: "Week"
};
});
</script>
Loading Events

Since both calendar views point to the same event data source (daypilot-events="events"
), all we need to do to fill both instances with data is to load the events to $scope.events
:
function loadEvents() {
$timeout(function() {
var params = {
start: $scope.week.visibleStart().toString(),
end: $scope.week.visibleEnd().toString()
};
$http.post("backend_events.php", params).success(function(data) {
$scope.events = data;
});
});
}
PHP backend (backend_events.php)
<?php
require_once '_db.php';
$json = file_get_contents('php://input');
$params = json_decode($json);
$stmt = $db->prepare("SELECT * FROM events WHERE NOT ((end <= :start) OR (start >= :end))");
$stmt->bindParam(':start', $params->start);
$stmt->bindParam(':end', $params->end);
$stmt->execute();
$result = $stmt->fetchAll();
class Event {}
$events = array();
date_default_timezone_set("UTC");
$now = new DateTime("now");
$today = $now->setTime(0, 0, 0);
foreach($result as $row) {
$e = new Event();
$e->id = $row['id'];
$e->text = $row['text'];
$e->start = $row['start'];
$e->end = $row['end'];
$events[] = $e;
}
header('Content-Type: application/json');
echo json_encode($events);
?>
ASP.NET MVC backend (BackendController.cs)
public class BackendController : Controller
{
CalendarDataContext db = new CalendarDataContext();
public class JsonEvent
{
public string id { get; set; }
public string text { get; set; }
public string start { get; set; }
public string end { get; set; }
}
public ActionResult Events(DateTime? start, DateTime? end)
{
var events = from ev in db.Events.AsEnumerable()
where !(ev.End <= start || ev.Start >= end) select ev;
var result = events.Select(e => new JsonEvent()
{
start = e.Start.ToString("s"),
end = e.End.ToString("s"),
text = e.Text,
id = e.Id.ToString()
})
.ToList();
return new JsonResult { Data = result };
}
}
Sample JSON response:
[
{
"id":"12",
"text":"Test",
"start":"2015-03-16T11:30:00",
"end":"2015-03-16T16:30:00"
}
]
Switching Day/Week View

Now we will add two buttons ("Day
" and "Week
") that will let the user switch between the day and week calendar view:
<div ng-app="main" ng-controller="DemoCtrl" >
<div class="space">
<button ng-click="showDay()">Day</button>
<button ng-click="showWeek()">Week</button>
</div>
<daypilot-calendar id="day"
daypilot-config="dayConfig" daypilot-events="events" ></daypilot-calendar>
<daypilot-calendar id="week"
daypilot-config="weekConfig" daypilot-events="events" ></daypilot-calendar>
</div>
<script type="text/javascript">
var app = angular.module('main',
['daypilot']).controller('DemoCtrl', function($scope, $timeout, $http) {
$scope.showDay = function() {
$scope.dayConfig.visible = true;
$scope.weekConfig.visible = false;
};
$scope.showWeek = function() {
$scope.dayConfig.visible = false;
$scope.weekConfig.visible = true;
};
});
</script>
Date Navigator

The next step will be adding a date navigator control using <daypilot-navigator>
AngularJS element. It will let us switch the visible date:
<div ng-app="main" ng-controller="DemoCtrl" >
<div style="float:left; width: 160px">
<daypilot-navigator id="navi"
daypilot-config="navigatorConfig" ></daypilot-navigator>
</div>
<div style="margin-left: 160px">
<div class="space">
<button ng-click="showDay()">Day</button>
<button ng-click="showWeek()">Week</button>
</div>
<daypilot-calendar id="day"
daypilot-config="dayConfig" daypilot-events="events" ></daypilot-calendar>
<daypilot-calendar id="week"
daypilot-config="weekConfig" daypilot-events="events" ></daypilot-calendar>
</div>
</div>
<script type="text/javascript">
var app = angular.module('main',
['daypilot']).controller('DemoCtrl', function($scope, $timeout, $http) {
$scope.navigatorConfig = {
selectMode: "day",
showMonths: 3,
skipMonths: 3,
onTimeRangeSelected: function(args) {
$scope.weekConfig.startDate = args.day;
$scope.dayConfig.startDate = args.day;
loadEvents();
}
};
});
</script>
Calendar CSS Themes
You can build your own CSS theme for DayPilot AngularJS Event Calendar using the online CSS theme designer. It is a WYSIWYG editor that lets you create themes by specifying styles for the calendar elements (events, headers, time cells).

History
- December 30, 2021: DayPilot Lite version 2021.4.341 now includes a new Angular Calendar component.
- January 9, 2017: Sample projects updated with the latest DayPilot Lite version
- December 12, 2016: DayPilot Lite for JavaScript 1.3 SP5 released
- April 20, 2016: DayPilot Lite for JavaScript 1.3 SP2 released
- January 11, 2016: DayPilot Lite for JavaScript 1.3 SP1 released
- October 25, 2015: CSS Theme Designer added (event calendar, navigator controls supported), DayPilot Lite for JavaScript 1.2 SP2 available
- April 26, 2015: Minor improvements
- March 23, 2015: ASP.NET MVC 5 backend added
- March 16, 2015: Initial release