I recently discovered the following warning from ReSharper:

So what exactly does Virtual member call in constructor actually mean, and why am I being warned about this?
Virtual members
In C# a virtual member is a method or property which is prepended with the modifier virtual, meaning that it may be overridden in a child class but unlike the abstract modifier it doesn't have to be. Since a virtual member may be overridden many times, only the most derived type will be executed.
Constructors
Since only the most derived virtual member is executed this can lead to a unique problem in the scenario where the virtual member is contained within a constructor. To understand this consider the following two classes:
class ClassA
{
public ClassA()
{
SplitTheWords();
}
public virtual void SplitTheWords()
{
}
}
class ClassB : ClassA
{
private readonly String _output;
public ClassB()
{
_output = "Constructor has occured";
}
public override void SplitTheWords()
{
String[] something = _output.Split(new[]{' '});
}
}
If I create an instance of ClassB
, then the constructor of ClassA
will be called and SplitTheWords()
will be executed. Now because SplitTheWords()
is virtual, then only SplitTheWords()
in ClassB will actually be executed (the most derived class) before the constructor of ClassB
has been. Which means we could be using an object in an unsuitable state.
Resharper recommends to make the virtual member in ClassA sealed, which means that it cannot be overridden and must be the most derived type, which entirely solves this problem.
I believe this is exactly the kind of thing which would get overlooked in your codebase, and just goes to show what a useful tool ReSharper actually is. A more concise explanation of this situation is available in the ReSharper docs.
Note about comments below:
Due to a typo I made, an earlier version of the post didn't exhibit the behaviour described, and I very much thank those who took the time to point this out to me. This have now been fixed, although I believe a deeper explanation as to the reasons behind this article is warranted:
[10.11] of the C# Specification tells us that object constructors run in order from the base class first, to the most inherited class last. Whereas [10.6.3] of the specification tells us that it is the most derived implementation of a virtual member (in this case ClassB) which is executed at run-time.
Therefore when you call a virtual member in a constructor the following can happen because the most derived implementation of a virtual member was contained within an object whose constructor hadn't been executed yet:
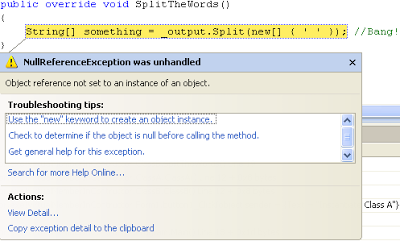