Reflection is one of the very nice features of .NET, with Reflection one can take out lotsa information about the type. When I say type that means it could be a .NET type that can be a class, enum, structure etc. with reflection you can get the methods of a class, parameters it takes and can even invoke the methods of that type. I’ll tell you the basics of reflection that will give you a strong basic knowledge to study it further and use in your code. This article could be short in length but my motive behind to write is to give basic information about reflection. So if I want to conclude the reflection definition in a sentence, “Reflection is a mechanism that provides you to drill the metadata of an assembly, module or types”.
So let’s start with the code, so that it’ll give you a nice idea about reflection, for a brief I’m going to create a normal class with some method and a constructor, some void methods, and a method that will take 2 int parameters to show the addition of them plus our main class that will retrieve the information of those methods in runtime irrespective they are public or private methods. Because reflection provides you that much flexibility so that you can invoke the private methods of a class, which you basically cannot do using that type with add reference in your code, interesting?? Yes it is.
I’ve created a class AeroPlane with some public method, private methods, some takes parameters, some are just to invoke, for better understanding refer the code below:
class AeroPlane
{
public AeroPlane()
{
Console.WriteLine("AeroPlane Constructor invoked");
}
private void Model()
{
Console.WriteLine("Airbus A380");
}
private void Company()
{
Console.WriteLine("Airbus");
}
private void CompanyLoacation()
{
Console.WriteLine("United States");
}
public void PublicMethod()
{
Console.WriteLine("This is public Method");
}
private void DoSum(int a, int b)
{
Console.WriteLine("Addition of {0} and {1} is {2}", a, b, a + b);
}
private void Age(string name, int age)
{
Console.WriteLine("Hi {0} your age is : {1}", name, age);
}
}
As you can see most of the methods I’ve created as private, there is no other reason apart; I wanted to invoke private method just for my fantasy. I’ve some void method plus two methods that takes parameters, so we are going to retrieve all the details of this class in any other class with the help of .NET Reflection.
Let’s see the structure of my class that will call the above class methods and retrieve the details of them, first we’ll see the code then we will see the output of my class below
namespace NamespaceReflectionInner
{
class TestReflection
{
Type _type = null;
public TestReflection()
{
_type = Type.GetType("AeroPlane");
}
public void Proceed()
{
_type = typeof(AeroPlane);
if (_type.IsClass)
{
ConstructorInfo[] _cInfo = _type.GetConstructors();
foreach (ConstructorInfo _ci in _cInfo)
{
Console.WriteLine(_ci.Name);
}
object obj = Activator.CreateInstance(_type);
MethodInfo _minfo = _type.GetMethod("Model", BindingFlags.Instance | BindingFlags.NonPublic);
if (_minfo != null)
{
_minfo.Invoke(obj, null);
}
MethodInfo[] _mInfoCollection = _type.GetMethods(BindingFlags.Instance | BindingFlags.NonPublic);
foreach (MethodInfo _mm in _mInfoCollection)
{
ParameterInfo[] _pInfo = _mm.GetParameters();
if (_pInfo.Length != 0)
{
object[] _param;
if (_mm.Name.Equals("DoSum"))
{
_param = new object[] { 2, 4 };
}
else
{
_param = new object[] { "Ashutosh", 23 };
}
_mm.Invoke(obj, _param);
}
else
{
_mm.Invoke(obj, null);
}
}
}
}
}
}
In above class as you can see I’ve retrieved the aeroplane class type. Type is a class that contains what kind of type it is, an array, class, interface, value type etc. Then in Proceed method I’m passing the type of my AeroPlane class inside this variable with typeof() keyword, that will bring the type inside my _type variable. Now what all things I’ll do, they all will come from this _type variable. Now in the next line I’m checking whether this _type is a class or not. If this is a class type then I’m checking the constructor inside this class with the help of constructorinfo. Constructorinfo provides the details of the constructor in the type specified. At foreach loop I’m trying to retrieve the name of constructor that will give me .ctor as output.
Okay now the main thing begins, at the next line I’ve created an object called “obj” inside that I’m calling something called Activator.CreateInstance(_type). This line will actually create an instance of my _type Type that is actually pointing to AeroPlane class. So now in my object I’ve an instance of AeroPlane class. This thing I required because of invoking my methods at runtime. For better understanding refer the image below, that will show you the instance that is coming inside obj at runtime.

Now let’s move to the next line where I’m using something called “MethodInfo”. This MethodInfo used to retrieve the method from that Type so that we can use them further in our code, in my code you can see I’m retrieving MethodInfo with the help of _type.GetMethod(“Model”) with some bindingflag parameter. There are two ways to get the method details, one to get all in an array using _type.GetMethods() and the second one I’ve used above _type.GetMethod(“MethodName”). With _type.GetMethod(“”) you can get a specific method in MethodInfo object. The bindingflag is a enumeration that help you to extract information of Type, bindingflag.instance will bring you instance, bindingflag.NonPublic will brings NonPublic method like private so that you can use them ahead in your code.so the code below will bring the Model, that is private as in nature and with MethodInfo.Invoke method I’m going to invoke them, so that I can see the output of that method.as you can see invoke method takes object obj as first parameter, this is the reason we’ve created this object in our code earlier to invoke methods. For better understanding please refer to the image below.
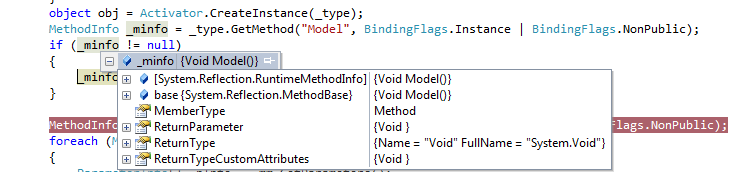
In the above image you can see, I’ve retrieved the Model method inside my MethodInfo object, now I can do lotsa things with this object.
Now let’s move ahead when you don’t wanna be specific while calling methods, in simple language when you want to retrieve all the method details and wants to invoke them one by one. As I said earlier there are two methods to retrieve method details; first one we saw already to get the details of a specific method, now we are going to use the next method that will bring out all the method inside MethodInfo array object, that is _type.GetMethods(). For better understanding please see the image below.
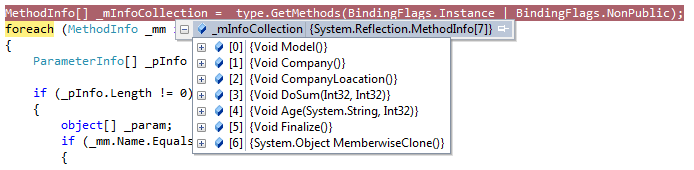
In above code you can see I’ve retrieved all the NonPublic method details inside _mInfoCollection object. Now we will invoke them one by one; as you can see most of the methods are void and taking no parameters; apart DoSum() that is taking two Int32 parameters and Age() that is taking String and Int32 as parameters, so with MethodInfo.Invoke() method we will invoke our parameterless methods, and those methods that are taking parameters we will invoke them with the help of ParameterInfo.
Before we start understanding the code first go through the image below
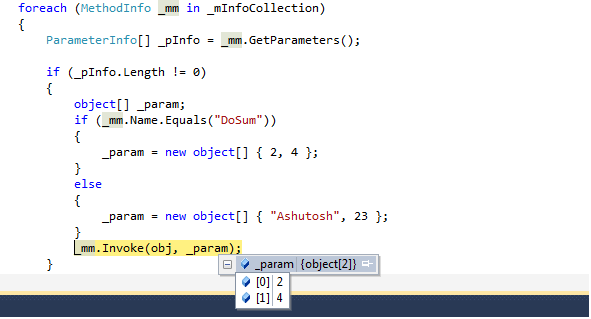
As you can see in the above code I’m iterating through the _mInfoCollection that is the collection of my methods as of now. Now with the help of ParameterInfo[] I’m trying to access their parameters and checking whether they take any parameter with the help of checking their length property. If they contain any parameter based on the method name, I’m creating the object array and passing my parameters and while invoking taking the second parameter as obj, remember earlier we were passing it NULL. So it will call parameterized methods with parameter as we passed, and for those methods that doesn’t contain any parameter you can pass it as NULL.
So at the end if you execute the code you will found the output below
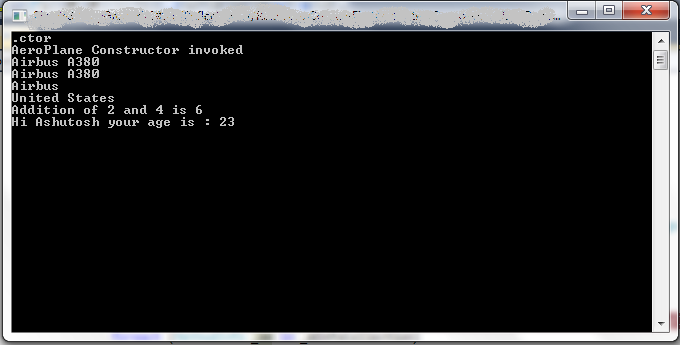
I hope it gave you some idea about Reflection in .NET
The ASP.NET Wiki was started by Scott Hanselman in February of 2008. The idea is that folks spend a lot of time trolling the blogs, googlinglive-searching for answers to common "How To" questions. There's piles of fantastic community-created and MSFT-created content out there, but if it's not found by a search engine and the right combination of keywords, it's often lost.
The ASP.NET Wiki articles moved to CodeProject in October 2013 and will live on, loved, protected and updated by the community.