QR codes have been winning popularity since Covid. I also have one on my business card. But did you know it’s pretty easy to create a QR code generator in C#? You could do it in like 40 lines of C# code!
Introduction
Everywhere you go, you see QR codes. I even saw them when I was in an Australian restaurant on the table. The idea was pretty cool: scan the QR code with your phone and you are taken to the online menu. There you order, pay, and wait for the food.
Maybe you take QR codes for granted, just like I did. But have you ever learned what QR code stands for or what it actually is? Well… I have!
A QR code (Quick Response code) is a two-dimensional barcode that can be scanned with a smartphone camera or a QR code scanner. QR codes can store a large amount of information, including website URLs, contact information, product details, and much more.
QR codes consist of black and white squares arranged in a grid pattern on a white background. The information encoded in a QR code is stored in the pattern of the squares and can be decoded using a QR code reader app on a smartphone or a specialized scanner.
You can find QR codes in a lot of things, including advertising, marketing, ticketing, and inventory management, among others. They are popular because they are easy to create and use, and can be read quickly and accurately using a smartphone camera.
This article will be simple and basic since the subject is QR codes and not how to build an over complicated WinForms or WPF application that takes a lot of time and code to show you 15 rows of QR code example code.
In this tutorial, I will use a console application containing the code to generate and read the QR code. How you are going to use that is up to you. You can easily copy that code and implement it in your own application. It will work whether that is an API, WPF, MAUI, Blazor, or whatever type you want.
ZXing.NET
Although you could write a QR code generator from scratch, it would be a complete waste of time. Why reinvent the wheel? And that is what makes our lives as developers a lot easier: most things have been invented already.
There are numerous libraries you can use to generate QR codes and read them. I really had trouble finding one library that did both. They do exist, but are paid or too complicated.
ZXing.NET is an open-source package that allows you to generate and read QR codes. And that is the package that we will be using in this tutorial. The idea is pretty simple: Create an image that represents the QR code. In the code is some text. Then we want to read that QR code and write that text in the console.
Easy, right? Let’s start!
Get Started
Let’s start by making a simple console application. I will be using .NET 7, but you can also use .NET 8 or 6.
After you have created the console application, you can install the package ZXing.NET. Note that they have separated the different targets into different packages. If you want to use ZXing for Android, you need a different NuGet package than when you target a Windows machine.
I will be using a Windows machine, so I install the following packages:
Install-Package ZXing.Net
Install-Package ZXing.Net.Bindings.Windows.Compatibility
That’s it! Now we can create the code to generate the QR code.
Creating the QR Code
Before we can read a QR code, we need to create it (duh). Since we are using ZXing.NET, it’s pretty easy to generate a QR code that contains some text.
The first thing we need to do is to determine where the image of the QR code needs to be saved. Then we create some options that are needed to generate the QR code. After that, we define a writer that needs to know what kind of format we want to create (QR code). And last, we will insert the text into the writer and save the bitmap.
If you would translate the previous paragraph into code, it would look like this:
string imageFileName = "klc_qr_code.png";
QrCodeEncodingOptions options = new() {
DisableECI = true,
CharacterSet = "UTF-8",
Width = 500,
Height = 500
};
BarcodeWriter writer = new() {
Format = BarcodeFormat.QR_CODE,
Options = options
};
Bitmap qrCodeBitmap = writer.Write("This is my QR code!");
qrCodeBitmap.Save(imageFileName);
Let’s walk through it from the top. First, I define the image file. I didn’t add a path, so this file will be saved in the same directory as the debug application (path to your project/bin/Debug/net7.0).
Next up is the QrCodeEndodingOptions
, which is the settings for the QR code. I think most of the options are clear, except for the DisableECI
. ECI is a specification for QR codes, but some readers have trouble reading QR codes with this specification. So it’s best to disable it… Why not disable it by default? Just saying.
Anyway, next is the BarcodeWriter
. Now, the first time I tried this code I was “Barcode… Writer?” ZXing is not only compatible with creating QR codes but also barcodes and other types. Pretty cool, huh?
The property Format is where you set what kind of graphic you want to create. I have set it to QR_CODE
, but feel free to explore other options.
Then we write text to the writer and place it in a bitmap. In the example, I have put “This is my QR code”, but feel free to add whatever you want.
That’s it! You cannot execute this code and find the image. Scan it with your phone and see what happens!
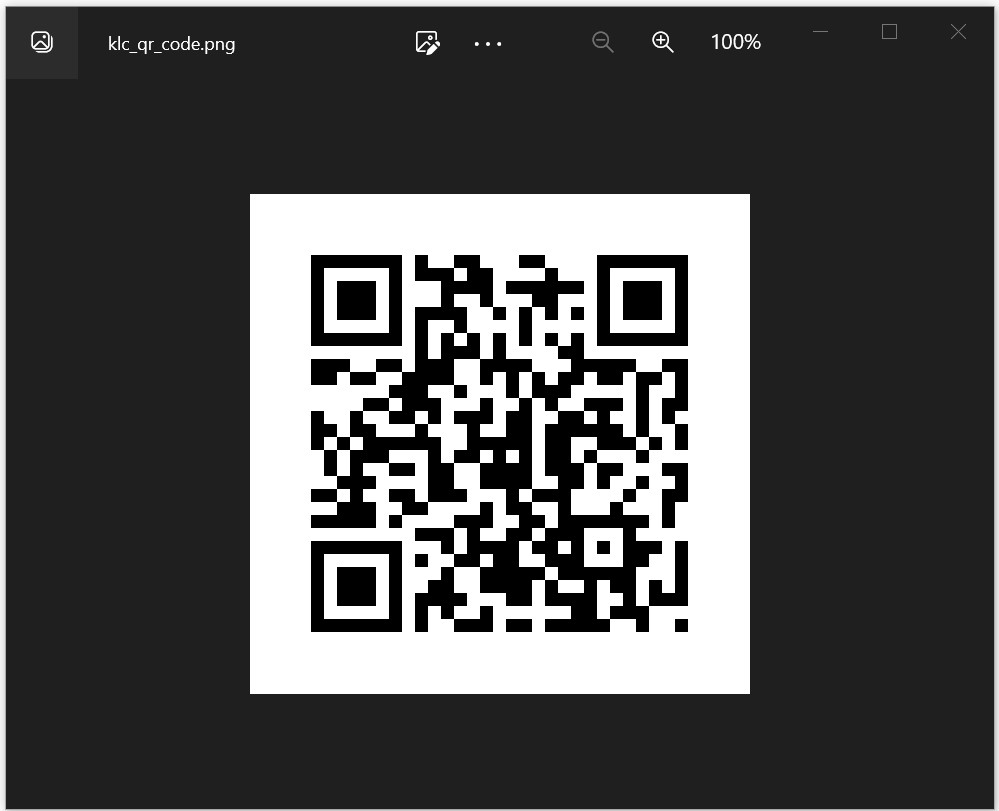
Reading the QR Code
Next up: Reading the QR code. You can use the QR code you just made, use my QR code from the image above, or some random QR code you find on the internet.
First, we need to set some options. Here, we define what kind of options we want to set up to read. Since ZXing can do more than just a QR code, we set it to a QR code. Then we will initialize a bitmap, which contains our QR code to read. Next is initializing a new reader, which can decode the bitmap to a result. The result contains the text. Simple!
Let’s look at the code:
DecodingOptions readOptions = new() {
PossibleFormats = new List<BarcodeFormat> { BarcodeFormat.QR_CODE },
TryHarder = true
};
Bitmap readQRCodeBitmap = new(imageFileName);
BarcodeReader reader = new() {
Options = readOptions
};
Result qrCodeResult = reader.Decode(readQRCodeBitmap);
if (qrCodeResult != null)
Console.WriteLine(qrCodeResult.Text);
As said, we start with the options, which is of the type DecodingOptions
. I have set the property PossibleFormats
to BarcodeFormat.QR_CODE
. If you want to fool around with other types, feel free to do so.
I must say I chuckled at the TryHarder
property. It basically means that when it’s set to try, it will look deeper into the bitmap.
Next up is the creation of the bitmap, which reads the QR code on the disk and transforms it into the bitmap.
After that, we initialize the BarcodeReader
and add the options to it. This reader will decode the bitmap in the next line, placing the result
in the variable qrCodeResult
.
Now we can get the text from the reader and show it in the console application.
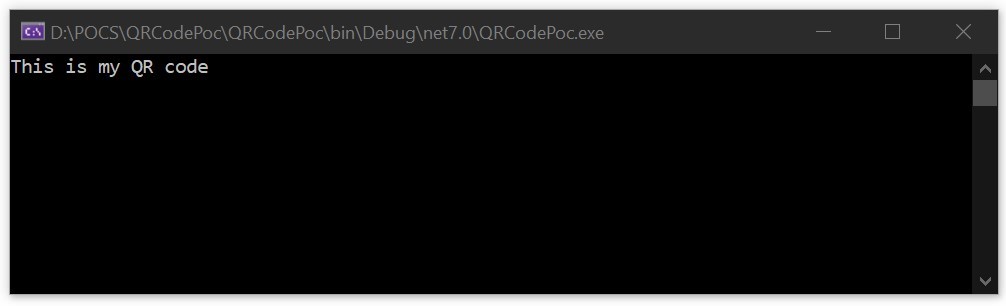
Other Options
Although text is cool and all, we can also add other types to the QR code. If you write an e-mail address instead of the text, you can scan that QR code with your phone, and your phone will suggest sending an e-mail to that email address.
If you write a phone number in the QR code, your phone allows you to directly call that number. Be careful with this though. One misplaced number and people are calling the wrong person.
But you can also write your whole contact information in a QR code. When someone scans it, he/she can save that contact information in their phone. This is done with a “MECARD
”. It’s the exact same code as before, but with a little bit more information to write to the QR code:
string imageFileName = "klc_qr_code.png";
QrCodeEncodingOptions options = new() {
DisableECI = true,
CharacterSet = "UTF-8",
Width = 500,
Height = 500
};
BarcodeWriter writer = new() {
Format = BarcodeFormat.QR_CODE,
Options = options
};
var contactInfo = new StringBuilder();
contactInfo.Append("MECARD:");
contactInfo.Append("N:Elzerman,Kenji;");
contactInfo.Append("TEL:123456789;");
contactInfo.Append("EMAIL:kenji.elzerman@kenslearningcurve.com;");
contactInfo.Append("ADR:123 Main St., Anytown, USA;");
contactInfo.Append("NOTE:Phonenumber and address are fake!");
Bitmap qrCodeBitmap = writer.Write(contactInfo.ToString());
qrCodeBitmap.Save(imageFileName);
There are way more ideas on how you can use QR codes. Whole scavenger hunts have been based on QR codes. You could even save small images in a QR code. No idea why you should, but you could!
Conclusion
QR codes are pretty easy to create and read. With just the right library, you can create a QR code and share it among friends, family, coworkers, or just random people. You can use it to send people to your website or share contact information without the chance of making a typo… If there was a type you made it.
There are a lot of libraries that could do the same trick as I have been showing you in this tutorial, but I find the ZXing library pretty easy. Plus, you cannot only generate QR codes, but you can also read them.
History
- 30th March, 2023: Initial version
I am a C# developer for over 20 years. I worked on many different projects, different companies, and different techniques. I was a C# teacher for people diagnosed with ADHD and/or autism. Here I have set up a complete training for them to learn programming with C#, basic cloud actions, and architecture. The goal was to help them to learn developing software with C#. But the mission was to help them find a job suitable to their needs.
Now I am enjoying the freedom of traveling the world. Learning new ways to teach and bring information to people through the internet.