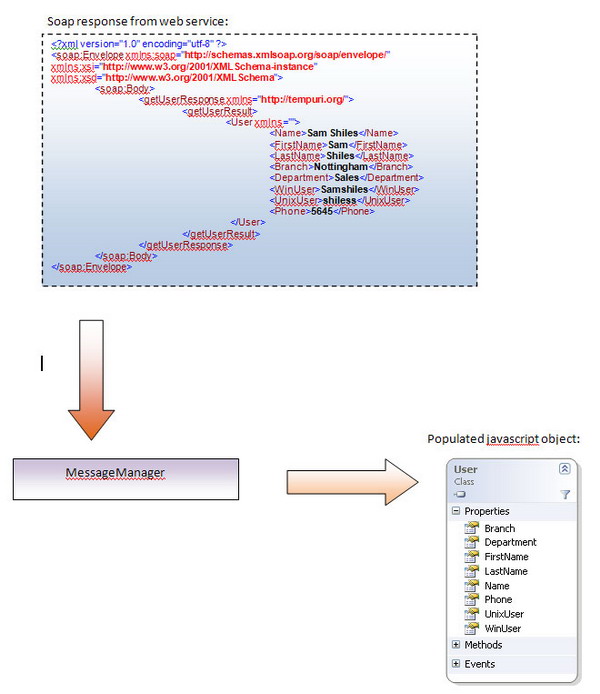
Introduction
The parsing and processing of XML response data from various sources (especially, SOAP messages returned from web services via the XMLHttpResponse
object) is becoming a more and more common task in the browser. The idea behind the solution presented in this article is to ease this parsing and processing by providing a simple and extensible solution to transform your responseXML
into more useful JavaScript objects. This article should also be useful to anyone wanting to start AJAX, and provides a good example of its use.
Background
The implementation presented in this article is done entirely in JavaScript. The principle, however, is applicable to many languages (e.g., .NET/Java) and shows a solution to the common problem of turning XML into more useful and more usable constructs. The best bit? Not one switch statement in sight.
Using the code
Using the messageManager is easy. Simply include the messageManager.js file in your page.
<script> type="text/javascript" </script>
Create an instance of the object:
var messageMan = new MessageManager();
Add in the maps; these link the XML node in the response data to the object that you wish to create and populate. For example, if your response data contained the following node:
<User>
<Name>Sam Shiles</Name>
<FirstName>Sam</FirstName>
<LastName>shiles</LastName>
</User>
...and you wanted to create a 'jsUser
' object from this noden you would create the map as follows:
messageMan.addMap("User","jsUser");
Next, you would create and send your message to the web service (in your preferred manner) and then pass the responseXML
into your messageManager
as follows (if oXmlHttpRequest
is the name of your XMLHttpRequest
instance):
var myJavascriptObjects=null;
myJavascriptObjects = messageMan.processMessage(oXmlHttpRequest.responseXML);
Note: the return type of MessageManager.processMessage(responseXML)
is a hashtable (as implemented in the HashTable.js file). The hashtable contains a collection of key/value pairs, with the key being the ID of the created object and the value being the object itself.
Points of interest
In order for the XML to object map to work successfully, a valid JavaScript object with a name corresponding to that which you entered into the map must be accessible to the 'MessageManager.js' file. In the source and demo attached, these objects reside in a file called 'bussinesObjects.js'. I would suggest that this is a sensible place to keep them, as long as the object is accessible to MessageManager.js (or the page which consumes this), it should work. Another key point in order to get a successful map is that the JavaScript/business object must implement a specific method and a specific field, as follows:
function fromXML(node)
{
this.id = node.selectSingleNode("Name").text;
return this;
}
For example:
function jsUser()
{
}
jsUser.prototype.fromXML = function(node)
{
this.name = XMLUtil.getSingleXpathValue(node, "Name");
this.id = this.name;
this.firstname = XMLUtil.getSingleXpathValue(node, "FirstName");
this.lastname = XMLUtil.getSingleXpathValue(node, "LastName");
this.branch = XMLUtil.getSingleXpathValue(node, "Branch");
this.department = XMLUtil.getSingleXpathValue(node, "Department");
this.winuser = XMLUtil.getSingleXpathValue(node, "WinUser");
this.unixuser = XMLUtil.getSingleXpathValue(node, "UnixUser");
this.phone = XMLUtil.getSingleXpathValue(node, "Phone");
return this;
}
Conclusion
Hopefully, the source code attached and demo provided should be fairly self-explanatory; the code is quite heavily commented. I would suggest downloading the demo first (it should work straight out of the box with the sample web service included).
Finally, many thanks to my friend Ian Harrigan on whose Java implementation the idea was based.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.