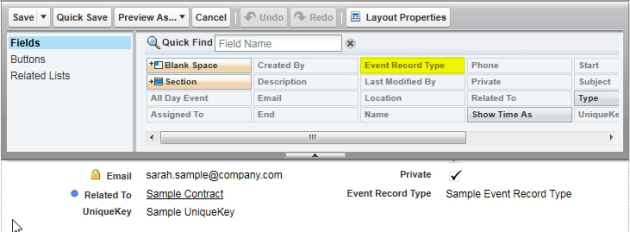
I have been twisting my head around in finding a solution for this simple task and I guess its worthwhile to share this information with everyone as I cannot find any solution related to my problem on Google or even in Developer Force. Eventually, it ended up calling their premier support for assistance, then after a week of emails sent back and forth, the answer was:
“you cannot get the default record type for user without logging to salesforce.com”
What that means is that you cannot get the default record type for a user if you are using a privileged account, you must use the users login credentials when performing the query, which defeats the purpose of having a super user account creating events for you programmatically.
Anyway, here is what I have initially done to get the default record type. At first, they suggested this solution in Java:
Schema.DescribeSObjectResult oSObjectResult = Event.SObjectType.getDescribe();
ListRecordTypeInfo> oRecTypeInfos = oSObjectResult.getRecordTypeInfos();
Schema.RecordTypeInfo has a method isDefaultRecordTypeMapping()
So I used that information to grab what I want in C#, so I created a method to extract that information:
public SforceService Authenticate()
{
try
{
SforceService oSalesForceService = new SforceService();
oSalesForceService.Timeout = 60000;
WebProxy oWebProxy = new WebProxy(WebRequest.DefaultWebProxy.GetProxy(
new Uri(oSalesForceService.Url.ToString())));
oWebProxy.Credentials = CredentialCache.DefaultCredentials;
oWebProxy.UseDefaultCredentials = true;
oSalesForceService.Proxy = oWebProxy;
LoginResult oLoginResult = oSalesForceService.login(sUserName,
string.Concat(sPassword, sToken));
oSalesForceService.Url = oLoginResult.serverUrl;
oSalesForceService.SessionHeaderValue = new SessionHeader();
oSalesForceService.SessionHeaderValue.sessionId = oLoginResult.sessionId;
GetUserInfoResult oUserInfo = oLoginResult.userInfo;
return oSalesForceService;
}
catch (Exception ex)
{
return null;
}
}
public string GetRecordType()
{
SforceService oSalesForceService = Authenticate();
string sRecordTypeId = "";
DescribeSObjectResult oObjectResult =
oSalesForceService.describeSObject("Event");
List<RecordTypeInfo> oRecTypeInfos =
oObjectResult.recordTypeInfos.ToList();
foreach (RecordTypeInfo oRecTypeInfo in oRecTypeInfos)
{
if (oRecTypeInfo.defaultRecordTypeMapping == true)
{
sRecordTypeId = oRecTypeInfo.recordTypeId;
}
}
return sRecordTypeId;
}
Using that will be an issue as it is not showing options on filtering it by Owner ID like what SalesForce
has in other tables, so the result shown after I executed the method is the default record type of the privileged Account. So I thought I can go directly to the related tables perform SQL queries like such:
oQueryResult = oSalesForceService.query(
"Select Id, Name from RecordType where SobjectType =
'Event' and IsActive = True and OwnerID = '" + sOwnerID +"'");
and might have some hope in there but after checking the RecordType
and RecordTypeInfo
, there is no field for Owner ID:


and in User
class, there are no fields for Default record type.

So the only real solution for this as of this post date is to keep a local copy of the default record type per user or as a Global setting if you wish. So when I create event, I assign the RecordTypeId
by getting it by the “Record Type Name” defaulted to a user which is stored locally.
oEvent.RecordTypeId = GetRecordTypeId(sUserDefaultRecordType);
public string GetRecordTypeId(string sRecordTypeName)
{
SforceService oSalesForceService = Authenticate();
QueryResult oQueryResult = null;
oSalesForceService.QueryOptionsValue = new QueryOptions();
oQueryResult = oSalesForceService.query(
"Select Id from RecordType where SobjectType = 'Event'
and IsActive = True and Name = '" + sRecordTypeName + "'");
if (oQueryResult.size != 0)
{
RecordType oRecordType = (RecordType)oQueryResult.records[0];
return oRecordType.Id;
}
return null;
}
I hoped I helped someone out there with a similar situation as I have.