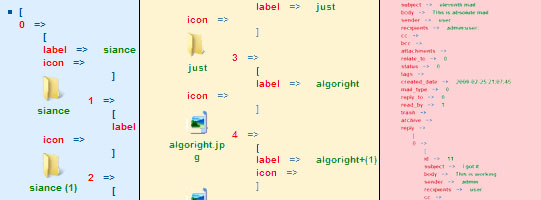
Introduction
I wrote this script for quick viewing of arrays and displaying HTML data in them. There are a lot of IDEs that support debugging but some times it is needed to scan arrays. The Print_r()
method of PHP is great but visually I think it is quite inconvenient for frequent viewing of arrays, particularly in recursive arrays. Here is a solution for this. This script returns string data that is basically the same as shown in the print_r()
method but it can help you via it visual separating, inner-array-tabbing style, and HTML source modes.
Basic Method
<?php
include("arrayViewer.php");
$array=array("first","second","third","fourth");
view_r($array);
?>
For return method, you may use the get_r()
method instead of the view_rr()
method.
echo get_r($array);
The output will be in this format:

1. Visual Separating Mode
There is a stylesheet file accompanying with the PHP script file which is needed to be imported in your web page to enable the visual separating mode in which keys are shown in red color and values are shown in green color.
<LINK REL = Stylesheet TYPE ="text/css" HREF ="arrayViewer.css" >
2. Inner Array Tabbing Style
This metThis
method can handle inner arrays. Inner values are shown one step tabbed than parent items.
<?php
include("arrayViewer.php");
$sub_sub_arr=array("sub_sub_item1",sub_sub_item2");
$sub_arr=array("sub_item1",sub_item2",$sub_sub_arr);
$arr=array("item1","item2",$sub_arr);
view_r($arr);
?>
The output will be like this:

HTML Source Mode
In conditions in which HTML content is stored in array items, itt will allow you to show HTML in default web view only, or both web view and HTML source code view at the same time.
<?php
include("arrayViewer.php");
$chArr=array("< div class='cls1'> </div>");
$array=array("html1"=>"< div class='clas1'> </div>",
"tow"=>"< div class='cls2'> </div>","sub"=>$chArr);
?>
Then to get normal HTML view:
view_r($array,"",false);
This feature is normally enabled, and option is available to hide HTML content and further attribute focusing. So to view source code only, you just need to call the default method:
view_r($array);
To get HTML source code view, use:
view_r($array,"",true);
The second argument is reserved for tab-space that is needed in the recursive method calls for arrays having inner arrays and it always needs to set a blank string.
Here are the related outputs (normal vs. HTML mode):

Focusing on HTML Attributes
It allows you to highlight an individual HTML attribute by specifying the attribute name as the fourth attribute in the method.
view_r($array,"",true,"class");
Here is the output:

And you can further specify highlighting option for the defined HTML attribute as:
view_r($array,"",true,"class","background: green;font-size: 20px;color: #fff;");
Then the output is:

Internal Architecture
function view_r($arr, $tab="", $encode=false, $focus_attr=null, $style=null) {
$return_debug = "<div style='width: 100%;'><pre>";
$return_debug.= "<pre>" . $tab . "[</pre>";
if (is_array($arr)) {
foreach ($arr as $key => $value) {
if (is_array($value)) {
$return_debug.= "<pre>" . $tab .
"<span class='array_key'>$key</span> => </pre>";
$return_debug.=view_r($value, $tab . " ",
$encode, $focus_attr, $style);
} else {
if ($encode) {
$return_debug.= "<pre>" . $tab . "<span class='array_key'>" .
$key . "</span> => <span class='array_value'>" .
$value . "</span></pre>";
$return_debug.= "<pre>" . $tab . "<span class='array_key'>" .
$key . "</span> => <span class='array_value'>" .
encode_html($value, $tab, $focus_attr, $style) . "</span></pre>";
}
else
$return_debug.= "<pre>" . $tab . "<span class='array_key'>" .
$key . "</span> => <span class='array_value'>" .
$value . "</span></pre>";
}
}
$return_debug.= "<pre>" . $tab . "]</pre><br />";
}
$return_debug.= "</pre></div>";
return $return_debug;
}
In the main foreach
loop of the method, it detects whether the value is an array or not, and if it is an array, it calls the view_r
method recursively, and if not, it calls encode_html()
that can also be used for other purposes. It also takes four arguments for HTML data: tab, focused attribute, and attribute style like the view_r()
method.
HTML encode method
This method will show HTML source of data in colorful style and with focusing feature for predefined attributes.
echo encode_html(array("<div class=’box’><h2 class=’heading’>text</h2></div>",
"",true,"class","color: red;");
Internal architecture for encode_html() method
function encode_html($str, $tab, $focus_attr=null, $style=null) {
$skip = 0;
$current_tag = null;
$html_close = false;
$html_open = false;
$return_str = "";
for ($i = 0; $i < strlen($str); $i++) {
if ($str{$i} == "<" && $skip == 0) {
$return_str.="<mark><</mark>";
$tag_arr = get_tag($str, $i, $focus_attr, $style);
$return_str.=$tag_arr["value"];
$skip = $tag_arr["skip"];
$html_open = true;
} elseif ($str{$i} == ">" && $skip == 0) {
$return_str.="<mark>></mark>\n$tab ";
$html_close = true;
} elseif ($str{$i} == "'" && $skip == 0 && $html_open) {
$quoted = get_quoted_value($str, $i, $focus_attr, $style, $current_tag);
$return_str.=$quoted['value'];
$skip = $quoted['skip'];
} elseif ($str{$i} == " " && $skip == 0 && $html_open) {
$current_tag = get_current_tag($str, $i);
$attr = get_attr($str, $i, $focus_attr, $style);
$return_str.=$attr['value'];
$skip = $attr['skip'];
} elseif ($skip > 0) {
$skip--;
}
else
$return_str.=$str{$i};
}
return $return_str;
}
function get_current_tag($str, $index) {
for ($new = $index + 1; $new < strlen($str); $new++) {
if ($str{$new} != "=" && $str{$new} != "/" &&
$str{$new} != ">" && $str{$new} != "<") {
$cur_tag.=$str{$new};
} else {
break;
}
}
return trim($cur_tag);
}
function get_attr($str, $index, $focus_attr=null, $style=null, $current_tag=null) {
$count = 0;
$return_quoted_arr = null;
if (isset($current_tag))
$quoted_text.="<attr" . check_focus($focus_attr, $current_tag, $style) . "> ";
else
$quoted_text.="<attr" . check_focus($focus_attr, "attr", $style) . "> ";
for ($s = $index + 1; $s < strlen($str); $s++) {
if ($str{$s} != "=" && $str{$s} != ">" && $str{$s} != "/")
$quoted_text.=$str{$s};
else {
$quoted_text.="</attr>";
$count = $s;
break;
}
}
$return_quoted_arr["skip"] = $count - $index - 1;
$return_quoted_arr["value"] = $quoted_text;
return $return_quoted_arr;
}
function get_tag($str, $index, $focus_attr=null, $style=null) {
$count = 0;
$return_quoted_arr = null;
$quoted_text.="<tag" . check_focus($focus_attr, "tag", $style) . ">";
for ($s = $index + 1; $s < strlen($str); $s++) {
if ($str{$s} != " " && $str{$s} != ">")
$quoted_text.=$str{$s};
else {
$quoted_text.="</tag>";
$count = $s;
break;
}
}
$return_quoted_arr["skip"] = $count - $index - 1;
$return_quoted_arr["value"] = $quoted_text;
return $return_quoted_arr;
}
function get_quoted_value($str, $index, $focus_attr=null,
$style=null, $current_tag=null) {
$count = 0;
$return_quoted_arr = null;
if (isset($current_tag))
$quoted_text.="<qoute>'</quote><attrvalue" .
check_focus($focus_attr, $current_tag, $style) .
check_focus($focus_attr, "attrvalue", $style) . ">";
else
$quoted_text.="<qoute>'</quote><attrvalue" .
check_focus($focus_attr, "attrvalue", $style) . ">";
for ($s = $index + 1; $s < strlen($str); $s++) {
if ($str{$s} != " " && $str{$s} != "'")
$quoted_text.=$str{$s};
else {
$quoted_text.="</attrvalue><qoute>'</quote> ";
$count = $s;
break;
}
}
$return_quoted_arr["skip"] = $count - $index;
$return_quoted_arr["value"] = $quoted_text;
return $return_quoted_arr;
}
function check_focus($focus_attr, $attr, $style) {
if ($focus_attr == null) {
return "";
} else {
if ($focus_attr == $attr) {
return " style='color: white; font-size: large;background: black;" .
$style . "' ";
}
else
return "";
}
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.