Introduction
This article will outline the method for creating Web Service methods that will return strongly-typed data to the consumer. I’ve seen some C# examples of this, but this one is presented using VB, and done in Visual Studio 2008 (it works the same in VS 2010). This example will also use a WebForms application to consume the Web Service.
This process involves using the xsd.exe tool from Visual Studio to generate a .xsd file from a .xml file, then further using the .xsd file to generate a class. Once the class has been created, it can be incorporated into the project and used to create strongly-typed data to return to the consumer.
Background
There have been examples presented that take you up to the part where you’re expected to know how to use this generated class. This is like saying “we are going to the movies” and then getting dropped off in front of the theater. I’d like to provide just a little more practical direction, and follow through. I’m going to take you inside, buy you a ticket and some popcorn, and enjoy the movie with you.
Using XSD.EXE to create a class
The procedure for creating the base class is straightforward. The input to the procedure is an XML file sample that represents the data being passed back to the consumer.
- Begin with the XML. Here’s a sample:
<YourCompany>
<Customer>
<CustomerID>10101</CustomerID>
<FirstName>Jim</FirstName>
<LastName>Roth</LastName>
<Phone>972-555-1212</Phone>
</Customer>
</YourCompany>
The XML will need to be saved in a file. Using this XML file, use xsd.exe (installed with Visual Studio) to create a schema definition (.xsd) output file. No parameters are required for this step.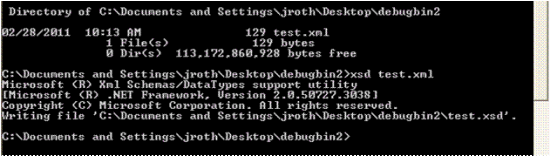
The next step is to use the .xsd file as an input to the xsd.exe command again and produce a class. The “/c” parameter specifies a class (the other option is “/d” for dataset). The “/l:vb” parameter indicates that a VB class will be generated. The default is C#, so choose carefully.
Make sure you take a close look at the generated class. If you’re going to make changes to the data types, this is the place to do it. Keep in mind that if you regenerate the class from xsd.exe, you’ll lose any changes.
Working with the generated class
Once the class has been generated, it will need to be added to the project. This can be accomplished by simply adding the generated source code file to the existing Web Service project. Once compiled, it’s available for use by the Web Service. From this point forward, the class properties can be referenced by name, and will provide Intellisense support to ensure proper use.
Here’s what a very simple solution in Visual Studio would look like:

This solution consists of a Web Service project and a web application project. The Web Service contains the class generated by xsd.exe (test.vb), which is referenced by Service1.asmx. The web application project has a web reference to the Service1 Web Service.
How to use the new class:
- Instantiate a reference to the class.
- Reference the properties using the familiar “dot” reference.
- Set the properties to the values desired.
- Return the strong type to the consumer.
This is a grossly oversimplified template of what a Web Service method might look like:
Imports System.Web.Services
Imports System.Web.Services.Protocols
Imports System.ComponentModel
Imports System.Xml.Serialization
<System.Web.Services.WebService(Namespace:="http://yourmom.com/webservices")> _
<System.Web.Services.WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)> _
<ToolboxItem(False)> _
Public Class Service1
Inherits System.Web.Services.WebService
<WebMethod()> _
Public Function StrongTypeCustomer() As YourCompanyCustomer
Dim eC As New YourCompanyCustomer()
eC.FirstName = "Jim"
eC.LastName = "Roth"
eC.CustomerID = 10101
eC.Phone = "972-555-1212"
Return eC
End Function
End Class
This Web Service method can now be referenced for its WSDL, the consumer can create a reference in their project, and the methods and properties are exposed according to the data types defined in the Web Service. Again, here’s another grossly oversimplified example of the consumer instantiating the Web Service and taking advantage of the strong data types. This example is from a web form that has a text box that will display the data returned from the Web Service. The project for the web application will need to create a web reference to the Web Service. Once that’s done, it can be instantiated and used.
Partial Public Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
Dim EC As New localhost.Service1()
TextBox1.Text = EC.StrongTypeCustomer.CustomerID().ToString + vbCrLf
TextBox1.Text += EC.StrongTypeCustomer.FirstName() + vbCrLf
TextBox1.Text += EC.StrongTypeCustomer.LastName() + vbCrLf
TextBox1.Text += EC.StrongTypeCustomer.Phone()
End Sub
End Class
It’s pretty easy once you’ve done it a couple of times. Hope it helps.
History
Sr. Director at Epsilon, a leader in marketing technology.