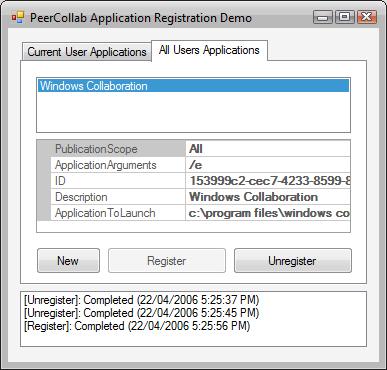
Background
This article shows how applications can be registered using Microsoft's new peer-to-peer Collaboration technology, in Windows Vista. Once registered, these applications can be discovered by other users signed into People Near Me. A future article will describe how registered applications can be used in an invitation to start the application and initiate the collaboration.
Applications are registered once when they are installed. Applications can either be registered for the Current User or All Users. Registration does not require that the application be signed in.
Introduction
Microsoft's entire Peer-to-Peer technology is exposed through the latest Platform SDK, as C/C++ API calls. However, the code in this article shows these APIs being used from .NET managed code using C#. The sample application includes a PeerCollab
class in which this article adds two properties that return a collection of registered applications and allows registering new applications. While the PeerCollab
class hides the details of using Microsoft's peer-to-peer collaboration APIs (in order to simplify the programming model), the flow of unmanaged calls is outlined below.
ApplicationsRegisteredForCurrentUser Property
This is a new property added to the PeerCollab
class which returns a collection of applications registered for the Current User.
public PeerApplicationRegistrationCollection
ApplicationsRegisteredForCurrentUser
{
get { return new PeerApplicationRegistrationCollection(
PeerApplicationRegistrationType.CurrentUser); }
}
The collection implements the IEnumerable
interface. The Reset
method of this interface calls the underlying PeerCollabEnumApplicationRegistrationInfo
API to retrieve the current list of registered applications.
ApplicationsRegisteredForAllUsers Property
This is a new property added to the PeerCollab
class which returns a collection of applications registered for All Users.
public PeerApplicationRegistrationCollection
ApplicationsRegisteredForAllUsers
{
get { return new PeerApplicationRegistrationCollection(
PeerApplicationRegistrationType.AllUsers); }
}
The same collection is used but with a parameter indicating All Users.
PeerApplicationRegisteration Class
The PeerApplicationRegisteration
class is either returned while enumerating a registered application collection, or can be created to register an application. This class wraps the PEER_APPLICATION_REGISTRATION_INFO
data structure. Before an application can be registered, the ID
, Description
, and ApplicationToLaunch
properties must contain values. An application must use the same GUID on all computers to identify itself.
public class PeerApplicationRegistration
{
private PEER_APPLICATION_REGISTRATION_INFO info;
public PeerApplicationRegistration(Guid ID, string Description,
string ApplicationToLaunch)
{
info = new PEER_APPLICATION_REGISTRATION_INFO();
this.ID = ID;
this.Description = Description;
this.ApplicationToLaunch = ApplicationToLaunch;
}
public Guid ID
{
get { return info.application.id; }
set { info.application.id = value; }
}
public string Description
{
get { return info.application.pwzDescription; }
set { info.application.pwzDescription = value; }
}
public string ApplicationToLaunch
{
get { return info.pwzApplicationToLaunch; }
set { info.pwzApplicationToLaunch = value; }
}
public string ApplicationArguments
{
get { return info.pwzApplicationArguments; }
set { info.pwzApplicationArguments = value; }
}
public PeerPublicationScope PublicationScope
{
get { return info.dwPublicationScope; }
set { info.dwPublicationScope = value; }
}
}
The ApplicationArguments
property is optional. The PublicationScope
property determines the visibility of the registered application to other People Near Me, and is a wrapper for the PEER_PUBLICATION_SCOPE
enumeration. It can have one of four values:
None
indicates the application is not visible to other People Near Me or Contacts.
NearMe
indicates the application is visible to other People Near Me.
Internet
indicates the application is visible to registered Contacts.
All
indicates the application is visible to People Near Me and registered Contacts.
I will discuss Contacts in a future article.
Register Method
Once the properties of the PeerApplicationRegisteration
class are populated, call the Register
method on the appropriate PeerApplicationRegistrationCollection
collection to register the application. This method uses the underlying PeerCollabRegisterApplication
API.
public void Register(PeerApplicationRegistration Application)
{
uint hr = PeerCollabNative.PeerCollabRegisterApplication(
ref Application.info, apps.registrationType);
if (hr != 0) throw new PeerCollabException(hr);
}
Unregister Method
Use the PeerCollab
ApplicationsRegisteredForCurrentUser
or ApplicationsRegisteredForAllUsers
properties to get a registered application. Then, use the Unregister
method on the collection to unregister the application. This method uses the underlying PeerCollabUnregisterApplication
API.
public void Unregister(PeerApplicationRegistration Application)
{
Guid id = Application.ID;
uint hr = PeerCollabNative.PeerCollabUnregisterApplication(
ref id, apps.registrationType);
if (hr != 0) throw new PeerCollabException(hr);
}
Using the Sample Application
The sample application only runs on Windows Vista. Two tabs are included to distinguish which applications are registered against the Current User and which are registered for All Users on the current computer. The list at the top shows the description of the currently registered applications.
foreach (PeerApplicationRegistration app
in collab.ApplicationsRegisteredForAllUsers)
{
listBox5.Items.Add(app);
}
The property grid shows the properties of the registered application. Click the Unregister button to un-register a selected application.
PeerApplicationRegistration app =
(PeerApplicationRegistration)propertyGrid3.SelectedObject;
try
{
collab.ApplicationsRegisteredForCurrentUser.Unregister(app);
listBox3.Items.Remove(app);
LogMessage("Unregister", "Completed");
}
catch (PeerCollabException ex)
{
LogMessage("Unregister", ex.Message);
}
Otherwise, click the New button to begin editing the properties for a new application.
propertyGrid3.SelectedObject = new
PeerApplicationRegistration(Guid.NewGuid(),
string.Empty, string.Empty);
Then, click the Register button to register it.
PeerApplicationRegistration app =
(PeerApplicationRegistration)propertyGrid3.SelectedObject;
if (app == null) return;
try
{
collab.ApplicationsRegisteredForCurrentUser.Register(app);
listBox3.Items.Add(app);
LogMessage("Unregister", "Completed");
}
catch (PeerCollabException ex)
{
LogMessage("Unregister", ex.Message);
}
Current User
To register applications for the Current User, start the sample application normally.
All Users
To register applications for All Users, right click on the sample application and Run As Administrator. Only Administrators can register applications for All Users.
Point of Interest
The February CTP of Windows Vista has a small bug were applications registered under All Users are not visible to all users. Here's the problem; under User1 Windows account, I register Notepad for All Users. When I switch users and login to a User2 account and enumerate what's registered for All Users, I would expect to see Notepad, however, I don't. If you are using a newer CTP, this problem will have been fixed. A special thanks to Tripp Parks and the folks at Microsoft, for looking into the problem and confirming it's been fixed.
The sample application is meant to show the mechanics of registering and un-registering an application. In reality, this would be done by your installer and not your application.
Links to Resources
I have found the following resources to be very useful in understanding peer-to-peer collaboration:
Conclusion
I promise the next article will be more exciting. It will show how you can detect other users signed in to People Near Me that have the sample application installed. It will then show how you can invite that person and, on acceptance, invoke the sample application to collaborate.
I'll be writing more articles to describe the other features of Peer-to-Peer Collaboration in the coming weeks and months, so stay tuned. If you have suggestions for other topics, please leave a comment. Don't forget to vote.
History
Initial revision.
Adrian Moore is the Development Manager for the SCADA Vision system developed by ABB Inc in Calgary, Alberta.
He has been interested in compilers, parsers, real-time database systems and peer-to-peer solutions since the early 90's. In his spare time, he is currently working on a SQL parser for querying .NET DataSets (http://www.queryadataset.com).
Adrian is a Microsoft MVP for Windows Networking.