Because Every New Framework Needs Another “Hello World!”
I’ve been forcing myself to try and learn this new .NET Core and in the past, I’ve written about writing C# code on Windows and running it in Linux using the Mono framework and recently, I wrote about using .NET Core with Yeoman to start an MVC application.
This, however, is a much simpler example to start understanding the .NET Core Framework.
Here are the dependencies for this example:
- Visual Studio Code (Download Link)
- Install .NET Core SDK
Now let’s open a terminal and run a few commands:
mkdir hwapp
cd hwapp
dotnet new
Ok, go ahead and run your new application:
dotnet restore
dotnet run
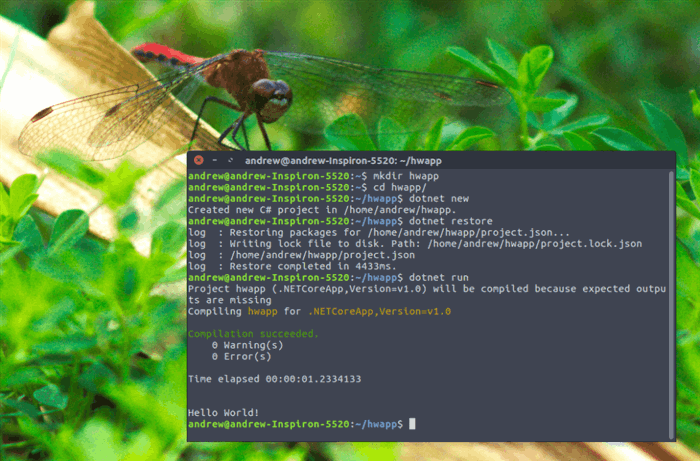
For those of you who read instructions, you may have seen these instructions already on Microsoft’s page for installing the .NET Core Framework, but what is happening when you run these commands?

First .NET Core is creating some files inside of the hwapp folder when you run “dotnet new”.
Let's look first at the contents of Project.json:
{
"version": "1.0.0-*",
"buildOptions": {
"debugType": "portable",
"emitEntryPoint": true
},
"dependencies": {},
"frameworks": {
"netcoreapp1.0": {
"dependencies": {
"Microsoft.NETCore.App": {
"type": "platform",
"version": "1.0.0"
}
},
"imports": "dnxcore50"
}
}
}
Above “version
” is the version of our application which starts at 1.0.0.
Under that, we have dependencies. This is where .NET Core gets interesting because it only provides the bare minimums by default (resulting in better performance) and then requires you to add dependencies for everything else you want in your application (MVC, Entity Framework, Identity, IIS Integration, etc.). These dependencies are then added to the project via Nuget when you run “dotnet restore” In this case, this is only dependent on .NET Core 1.0, but it’s worth noting that even this dependency can be replaced with net46 for .NET 4.6 on Windows or even the Mono Framework.
Now Let's Look at Program.cs
using System;
namespace ConsoleApplication
{
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
If you’ve ever seen a C# Hello World Console Application, this is nothing new. We can even make it slightly more interesting and add some variables:
using System;
namespace ConsoleApplication
{
public class Program
{
public static void Main(string[] args)
{
var hw = "Hello";
hw += " ";
hw += "World!";
var question = "What is 1 + 1?";
var math = 1+1;
var answer = "Answer: 1 + 1 is equal to ";
answer = String.Concat(answer,math);
Console.WriteLine(hw);
Console.WriteLine(question);
Console.WriteLine(answer);
}
}
}
Here’s what it looks like in Visual Studio Code with the C# extension installed:

and here is what that code looks like when it’s running:

Hopefully, this helps with a basic understanding of what this new cross platform framework can do and how it runs. I think it’s excellent that I was able to do this all in Linux on Ubuntu 16.04 which has now become my main OS on my home laptop and I’m excited to see more from Microsoft.
If you have any questions or comments, please drop them below and feel free to share this post!
CodeProject
The post .NET Core Hello World Console App (in Ubuntu 16.04) appeared first on Blank's Tech Blog.
I’m a dad, a husband, a tech enthusiast, a hobbyist photographer, and at work an associate software developer for VB6 and C# applications.