Introduction
We can use the TI-83 or TI 84 calculators to do more than analyze graphs and simulate probabilistic events; we can program them to solve repetitive math problems. Programming a calculator helps us solve problems faster, decrease chances of typos, and more easily apply brute-force algorithms.
In this tutorial, we learn TI-BASIC, the language for programming the TI-83 or TI-84, and then programmatically solve six math problems.
Background
Programming-related activities are available in the “Programming Screen”, which appears by pressing [PRGM]
. At the top of the screen are three tabs:
- EXEC: execute a program. We will be executing the programs we make later in this tutorial
- EDIT: view or modify a program’s code. We would likely visit this tab when debugging programs
- NEW: Create a new program.
To delete a program, head to Memory -> Mem Mgmt/Del -> Prgm
, [2ND][+][2][7]
, and scroll to the desired program and press [DEL]
.
To type a letter, press [ALPHA]
followed by the key with the desired letter on top.
"Hello World"
Let’s start by creating a new program: [PRGM] -> NEW -> 1:Create New
, that displays the message “HELLO WORLD”. In this tutorial, we’ll name our program “HELLO”.
TI-BASIC has a set of pre-made commands, also known as tokens, which makes the calculator do specific tasks, such as displaying a message, comparing values, and even turning on and off individual pixels. If you programmed in other languages, you can think of tokens as keywords. We use tokens in our programs.
To view tokens, press [PRGM]. At the top of the new screen are three tabs, each containing many tokens:
- CTL (Control): These tokens control a program’s structure. Frequently used tokens include if/else and loops.
- I/O (Input/Output): These tokens get input from the user and display messages, graphs, and tables.
- EXEC: We can execute other programs in our program
To display a “HELLO WORLD” message, select the third token 3:Disp
in the I/O tab, then type “HELLO WORLD”.
To run the program, return to the home screen by pressing [2ND][MODE]
, then [PRGM]
and select HELLO, and press [ENTER]
.
Code, Execution,


Frequently Used Tokens
Here is a list of tokens used in most programs:
I/O Tokens
Disp [String or number]
| Displays a string or number onto the screen. Strings are left-aligned and numbers are right aligned
| Disp "HELLO WORLD" OR
Disp 5*9
|
Prompt [Variable]
| Asks user to input a number, which is stored into a variable
| Prompt A (stores the number user inputs into the variable A)
|
CTL Tokens
If [condition]
Then
[Statement(s)]
Else
[Statement(s)]
End
| Creates If/Else scenarios. There are three types.
If [condition]
[Statement]
we execute only the next statement if the condition is true
If [condition]
Then
[Statement(s)]
End
We execute all the statements between the Then and End tokens if the condition is true
If [condition]
Then
[Statement(s)]
Else
[Statement(s)]
End
We execute multiple statements and include a else clause.
| If A=42
Disp "ONE STATEMENT ONLY"
If A=42
Then
Disp "TWO"
Disp "STATEMENTS"
End
If A=42
Then
Disp "TRUE"
Else
Disp "FALSE"
End
|
For (X, start, end)
[Statement(s)]
End
| The value of X begins at start, and after the statements between the For and End tokens are run, the value of X increases by 1. This continues until the value of X is equal to end. This is a for loop.
| For (X,1,10)
Disp X
End
(this displays the numbers 1 to 10)
|
While [condition]
[Statement(s)]
End
| The statements execute as long as the condition is true or is 1. This is a while loop.
| While X=42
Disp "X IS STILL 42"
End
|
Repeat [condition]
[Statement(s)]
End
| This is similar to a while loop except the statements are run once and then the conditions are checked, or more succinctly, this is a while loop whose statements are guaranteed to run at least once. This is the equivalent of a do/while loop.
| 42->X (assigns X to the value of 42, press the [STO>] key to make the arrow symbole)
Repeat X=42
Disp "X IS 42"
End
|
Stop
| Exits a program
| While 1
Disp "WILL DISPLAY"
STOP
Disp "WILL NOT DISPLAY"
End
|
Note: To assign a number to a variable, we press [STO>]
, which creates an arrow symbol (->). Eg, 42->X
assigns X
to be 42
Solving Math Problems
Let’s programmatically solve a couple math problems to become more familiar coding TI-BASIC. We assume all user inputs are numbers.
Math Problem 1: Multiply input by 10
Eg, if user inputs 5, our program displays 50.
To ask the user to input a number, we use the Prompt
token. To display ten times a number, we use the Disp
token. Here’s our code:
Prompt X (this asks the user to supply a value and that value is stored in X)
Disp 10X (we display 10*X)
Alternatively, we could also have reassigned the value of X
to 10X
.
Prompt X
10X->X
Disp X
Math Problem 2: Brute-force the sum of the numbers between two inputs
Eg, if user inputs 1 and 5, our program displays 9 because 2+3+4=9.
Since we ask the user for two numbers, we use two Prompt
tokens. To iterate through the numbers between the user’s two numbers, we can use a for, while, or repeat (do/while) loop. Let’s assume that the user’s second number is larger than the first. To increase program clarity, we also let a variable, S
, to store the sum of the numbers.
Prompt X
Prompt Y
0->S
For (A,X+1,Y-1)
S+A->S
End
Disp S
Math Problem 3: Powers with For loop
Eg, if the user inputs 3 and 4, our program displays 81.
Although we can easily find the power of a number with the [^]
key, we try finding it the old fashioned way: multiple multiplications.
Similar to the previous program, we can use two Prompt
tokens to ask the user to input two numbers. However, a more space-saving technique is to combine the two Prompt
tokens into one: Prompt X,Y
. We use a for loop to continuously multiple the first number with itself until the number of times it has multiplied by itself is equal to the second number. Let’s also make a variable, P
, that stores the final result.
Prompt X,Y
1->P
For (A,1,Y)
PX->P
End
Disp P
Math Problem 4: Determine if an input is prime or composite
Eg, if the user inputs 79, our program displays “PRIME”. If the user inputs 80, our program displays “COMPOSITE”.
A number is prime if its only factors are 1 and itself. More descriptively, a number is prime if there are no numbers from 2 to the square root of that numbers that, when divided from the number, has a remainder of 0. Otherwise, a number is composite.
To check if a number is prime, we loop through every number from 2 to its square root and check if it divided by the looped number has a remainder of 0. To check the remainder, we can use the remainder function from [Math]->NUM -> 0:remainder(
. If the remainder is 0, our number is composite so we display “COMPOSITE” and exit the program. If all the numbers have been looped, then our number must be prime and we display “PRIME”.
Prompt X
For (A,2,sqrt(X))
If remainder(X,A)=0
Then
Disp "COMPOSITE"
Stop
End (marks the end of the if statement)
End (marks the end of the for loop)
Disp "PRIME"
Math Problem 5: Finding the GCD of two inputs with Euclid's Algorithms
Eg, if user enters 72 and 15, our program displays 3.
Note: there’s also a built-in GCD function, which is in [MATH]->NUM->9: gcd(
.
Euclid’s Algorithm relies on two theorems:
- If a = bt + r, then gcd(a, b) = gcd(b, r)
- If a = bt, then gcd(a, b) = b
…and determines the GCD of two numbers by repetitively applying the first theorem to create new equations with smaller numbers, until they eventually reduce to the second theorem (notice there’s no r term).
Eg, To find gcd(72,15):
72 = 15 * 4 + 12
By Theorem 1, gcd(72,15) = gcd(15,12). For the sake of this example, we assume we don’t know gcd(15, 12). Since gcd(…) can be expressed as an equation, and vice versa, and our goal is to eventually arrive at an equation of the form a = bt, let’s try to express gcd(15, 12) as the GCD of two smaller numbers, and in doing so, we get a smaller equation. In this equation, a = 72, b = 15, r = 12, the remainder of 72/15. Reducing:
15 = 12 * 1 + 3, since gcd(15,12) = gcd(12,3)
Notice that in this equation a = 15, which was the value of b in the previous equation; b = 12, which was the value or r in the previous equation; r = 3, which is the remainder of 15/12).
12 = 3* 4, since r is now 0, Euclid’s Algorithm finishes
Thus, by theorem 2, gcd(72, 15) = 3.
We note several observations when we reduce one equation to another:
- a in the new equation = b in previous equation
- b in new equation = r in previous equation
- r in new equation = remainder of new a and new b
- If r in the new equation is 0, then the GCD is the new b. If r in the new equation is not 0, then repeat.
We code Euclid’s Algorithm:
Prompt A,B
remainder(A,B)->R
Repeat R=0
B->A
R->B
remainder(A,B)->R
End
Disp "THE GCD IS",B (we can shorten two Disps into one by separating the messages with a comma)
Alternatively, we could also write a slightly longer version, which is equally valid:
Prompt A,B
remainder(A,B)->R
While 1
If R=0
Then
Disp "THE GCD IS",B
Stop
Else
B->A
R->B
remainder(A,B)->R
End
End
Math Problem 6: Finding the radius of an incircle
Eg, An incircle is a circle inscribed in a triangle. If the user inputs 3,4,5 as the side lengths of the triangle, our program displays 1.
Although this problem is more mathematical than algorithmic, it is a good example of using elegance and simplicity over brute power. The calculator is a tool, a helper, whose usefulness depends on the strategy its human user chooses. There are many ways to solve this problem but let’s choose one that minimizes code.
Let a, b, and c be the sides of a triangle. Let r be the radius of the incircle.
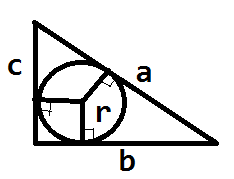
Since the incircle is tangeant to a, b, and c, it forms 90 degrees with each side. Therefore, the area of the triangle is ar/2 + br/2 + cr/2. By Heron’s formula, the area of the triangle is also \(\sqrt{(s(s-a)(s-b)(s-c))}\), where s is the semiperimeter of the triangle, (a+b+c)/2. Equating the two expressions for calculating the triangle’s area, we arrive with:
sqrt(s(s-a)(s-b)(s-c)) = ar/2 + br/2 + cr/2 (please use the square root symbol instead of typing sqrt)
= r(a+b+c) / 2
= sr
r = sqrt(s(s-a)(s-b)(s-c)) / s
Let’s write this in code:
Prompt A,B,C
(A+B+C)/2->S
sqrt((S(S-A)(S-B)(S-C)))/S->R (please use the square root symbol instead of typing sqrt)
Disp "THE INCIRCLE’S RADIUS IS",R
Concluding Thoughts
We programmatically solved six math problems! Air five! I hope this article provided you with a better understanding about some of the programming capabilities of the TI-83 / TI-84. Although we focused exclusively on algebra, you can also make programs to solve problems in calculus, probabilties, linear algebra, and so on.
Thank you for reading. If you have any feedback, please leave a comment below.
Further Reading
I'm a software developer with experience in infrastructure, back-end, and front-end development. I graduated with a BASc in Computer Engineering from the University of Waterloo in 2019. To learn how my skills may align with your needs, please feel free to send me a message or view my portfolio at: https://lennycheng.com