If you want to make a Web barcode reader app using node.js, you may find a node barcode module with npm or build it yourself. Node addons are the bridge between C/C++ libraries and JavaScript. In this tutorial, I will illustrate how to create node barcode addon on Ubuntu with DBR (Dynamsoft Barcode Reader for Linux).
Download & Installation
If you want to use the latest node version, do not install node and npm with the apt-get
utility, which only fetches the old version and will cause the error – ‘FunctionCallbackInfo’ does not name a type
:
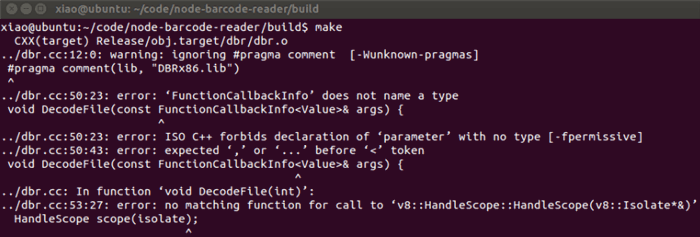
Install Node
Download the latest node package: node-v5.3.0-linux-x64.tar.gz.
Extract the node package as follows:
tar -xzf node-v5.3.0-linux-x64.tar.gz
Open .bashrc:
nano ~/.bashrc
Export node path and save .bashrc:
export PATH=$(YOUR_HOME)/Downloads/node-v5.3.0-linux-x64/bin:$PATH
Install node-gyp
:
npm install -g node-gyp
Install DBR
Download v4.0.0-pre-alpha.tar.gz.
Extract the dbr package:
tar -xzf v4.0.0-pre-alpha.tar.gz
To find the shared library when building the project with make
, create a symbolic link of barcode shared library:
sudo ln -s $(DynamsoftBarcodeReader)/Redist/libDynamsoftBarcodeReaderx64.so
/usr/lib/libDynamsoftBarcodeReaderx64.so
How to Make Node Barcode Addon
Create binding.gyp and specify the paths of DBR header files and shared libraries:
{
"targets": [
{
"target_name": "dbr",
"sources": [ "dbr.cc" ],
"include_dirs" : [
"$(DynamsoftBarcodeReader)/Include"
],
"libraries": [
"-lDynamsoftBarcodeReaderx64", "-L$(DynamsoftBarcodeReader)/Redist"
]
}
]
}
Create dbr.cc using the sample code provided by Dynamsoft Barcode Reader SDK:
#include <node.h>
#include "If_DBR.h"
#include "BarcodeFormat.h"
#include "BarcodeStructs.h"
#include "ErrorCode.h"
using namespace v8;
const char * GetFormatStr(__int64 format)
{
if (format == CODE_39)
return "CODE_39";
if (format == CODE_128)
return "CODE_128";
if (format == CODE_93)
return "CODE_93";
if (format == CODABAR)
return "CODABAR";
if (format == ITF)
return "ITF";
if (format == UPC_A)
return "UPC_A";
if (format == UPC_E)
return "UPC_E";
if (format == EAN_13)
return "EAN_13";
if (format == EAN_8)
return "EAN_8";
if (format == INDUSTRIAL_25)
return "INDUSTRIAL_25";
if (format == QR_CODE)
return "QR_CODE";
if (format == PDF417)
return "PDF417";
if (format == DATAMATRIX)
return "DATAMATRIX";
return "UNKNOWN";
}
void DecodeFile(const FunctionCallbackInfo<Value>& args) {
Isolate* isolate = Isolate::GetCurrent();
HandleScope scope(isolate);
String::Utf8Value fileName(args[0]->ToString());
String::Utf8Value license(args[1]->ToString());
char *pFileName = *fileName;
char *pszLicense = *license;
__int64 llFormat = (OneD | QR_CODE | PDF417 | DATAMATRIX);
int iMaxCount = 0x7FFFFFFF;
ReaderOptions ro = {0};
pBarcodeResultArray pResults = NULL;
if (pszLicense)
{
printf("license: %s\n", pszLicense);
DBR_InitLicense(pszLicense);
}
else
DBR_InitLicense("AC4561856D63EF392F46D7454052372D");
ro.llBarcodeFormat = llFormat;
ro.iMaxBarcodesNumPerPage = iMaxCount;
int ret = DBR_DecodeFile(pFileName, &ro, &pResults);
printf("ret: %d\n", ret);
if (ret == DBR_OK){
int count = pResults->iBarcodeCount;
pBarcodeResult* ppBarcodes = pResults->ppBarcodes;
pBarcodeResult tmp = NULL;
Local<Function> cb = Local<Function>::Cast(args[2]);
const unsigned argc = 1;
Local<Array> barcodeResults = Array::New(isolate);
for (int i = 0; i < count; i++)
{
tmp = ppBarcodes[i];
Local<Object> result = Object::New(isolate);
result->Set(String::NewFromUtf8
(isolate, "format"), Number::New(isolate, tmp->llFormat));
result->Set(String::NewFromUtf8
(isolate, "value"), String::NewFromUtf8(isolate, tmp->pBarcodeData));
barcodeResults->Set(Number::New(isolate, i), result);
}
DBR_FreeBarcodeResults(&pResults);
Local<Value> argv[argc] = { barcodeResults };
cb->Call(isolate->GetCurrentContext()->Global(), argc, argv);
}
}
void Init(Handle<Object> exports) {
NODE_SET_METHOD(exports, "decodeFile", DecodeFile);
}
NODE_MODULE(dbr, Init)
Automatically generate the project build files:
node-gyp configure
Build the project to produce dbr.node
:
node-gyp build
Write a test dbr.js which loads SDK license and invokes native reader methods:
var dbr = require('./build/Release/dbr');
var readline = require('readline');
var fs = require('fs');
fs.readFile('./license.txt', 'utf8', function (err, data) {
if (err) throw err;
var rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
var license = data.trim();
rl.question("Please input a barcode image path: ", function(answer) {
dbr.decodeFile(
answer, license,
function(msg){
var result = null;
for (index in msg) {
result = msg[index]
console.log(result['format']);
console.log(result['value']);
console.log("##################");
}
}
);
rl.close();
});
});
Run the script:
node dbr.js
