Introduction
C# interfaces have always been a confusing concept to understand and implement in code for beginner programmers. I will be explaining 'interface
' with an example that shows what, why and when to use them. In our next article, we be discussing some advanced feature of C# interface.
What is Interface
Book Definition: An interface contains only the signatures of methods, properties, events or indexers. A class or struct that implements the interface must implement the members of the interface that are specified in the interface definition.
Definition: Interfaces provide you with the ability to define methods, properties, events or indexers, which can be implemented in a class or struct as per user requirement. It is mandatory to implement all the members of an interface.
To demystify, interfaces are a cure for multiple inheritance in C#. You can implement multiple interface in a single class. Every class in your program can have 'n' number of Interfaces with different implementation as per your requirement.
Example:
public interface IDetail
{
string FirstName { get; set; }
string LastName { get; set; }
string Role { get; set; }
string GetDetail();
string MyWork();
}
public interface IEmployementContract
{
dateTime ContractDate {get;set;}
string ContractTerms {get;set;}
string GetContractDetails();
}
public class Employee : IDetail, IEmploymentContract
{
}
Key Syntax Points
- Access modifiers are not required by interface as by default all the member are
public
. Even if you add a modifier, you will get a compile time error. - The properties in the interface have the same syntax as the implementation in the class or structure.
Why To Use Interface
Before we dig into 'WHY', always remember this sentence "Program to an Abstraction rather to concrete class".
Interfaces provide you with better code maintainability and Unit testing.
Scenario
A television shows different channels to you based on your selection. Basically, it is providing you with a single interface to multiple channels. You don't have to buy separate televisions for each channel. In addition, different televisions can have the same channels.
Let's have an diagram to understand the Scenario.
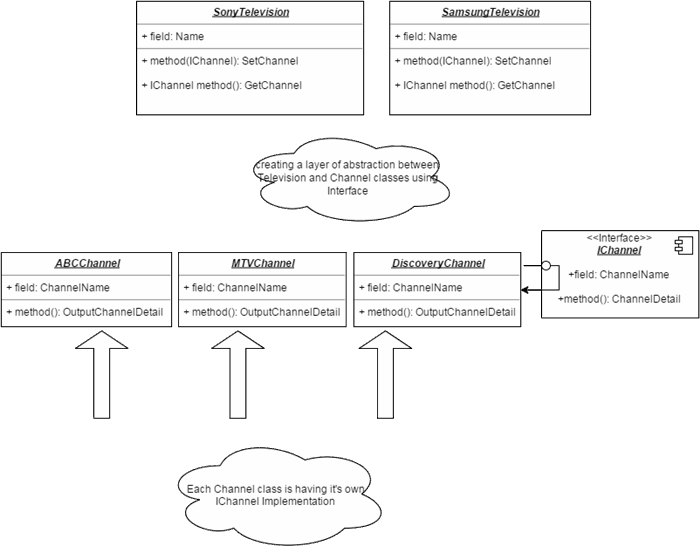
Let us convert our UML diagram to C# code.
Using the Code
public interface IChannel
{
string ChannelName { get;}
void OutputChannelDetails();
}
public class ABCChannel : IChannel
{
private readonly string _channeName;
public ABCChannel(string channelName)
{
this._channelName = channelName;
}
public string ChannelName { get { return _channel_Name; } }
public void OutChannelDetails()
{
Console.WriteLine(string.Format("This is {0} channel", ChannelName));
}
}
public class MTVChannel : IChannel
{
private readonly string _channelName;
public MTVChannel(string channelName)
{
this._channelName = channelName;
}
public string ChannelName { get { return _channelName; } }
public void OutputChannelDetails()
{
Console.WriteLine(string.Format("This is {0} channel", ChannelName));
}
}
public class DiscoveryChannel : IChannel
{
private readonly string _channelName;
public DiscoveryChannel(string channelName)
{
this._channelName = channelName;
}
public string ChannelName { get { return _channelName; } }
public void OutputChannelDetails()
{
Console.WriteLine(string.Format("This is {0} channel", ChannelName));
}
}
public class SonyTelevision
{
public IChannel ChannelType { get; set; }
public IChannel GetChannel()
{
return ChannelType;
}
public void SetChannel(IChannel channel)
{
ChannelType = channel;
}
}
public class SamsungTelevision
{
public IChannel ChannelType { get; set; }
public IChannel GetChannel()
{
return ChannelType;
}
public void SetChannel(IChannel channel)
{
ChannelType = channel;
}
}
Understanding the Code
The below code shows our Main
method where the program starts to execute.
static void Main(string[] args)
{
ABCChannel abcChannel = new ABCChannel("ABC Channel");
MTVChannel mtvChannel = new MTVChannel("MTV Channel");
DiscoveryChannel discoveryChannel = new DiscoveryChannel("Discovery Channel");
SamsungTelevision samsungTelevision = new SamsungTelevision();
samsungTelevision.SetChannel(abcChannel);
samsungTelevision.GetChannel().OutputChannelDetails();
SonyTelevision sonyTelevision = new SonyTelevision();
sonyTelevision.SetChannel(abcChannel);
sonyTelevision.GetChannel().OutputChannelDetails();
}
We have created instances of all channel classes (abcChannel
, mtvChannel
and discoveryChannel
) and have also created instances of all Television
classes (i.e., samsungTelevision
and sonyTelevision
). We are passing the abcChannel
object in the SetChannel
method of SamsungTelevison
class and SonyTelevision
, which can basically take any instance of a class that implements IChannel
Interface.
If we would had hard code ABCChannel
class object as the parameter for SetChannel
method. It would not be possible to pass any other Channel
object (e.g., MTVChannel
and DiscoveryChannel
). But because of the beauty of Interface, we can pass any object that implements IChannel
Interface. That's where code maintainability comes into the picture. In the future, we can easily add more Channel
classes that implement the IChannel
interface and pass it to SetChannel
method as no class object is hard coded in the SetChannel
parameter.
There is much more to Interface which will be discussed in detail in my next article where I will be discussing Interfaces crucial role in dependency Injection.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.