Introduction
Before we get into how URL Routing works in MVC, let's have a quick look at the MVC architecture.
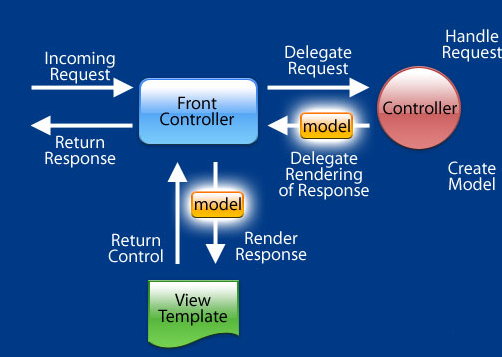
By seeing this high level architecture diagram, we can say that the Controller will handle the user requests. The complete flow is as follows:
- User’s request is first passed to the controller
- Controller parses the request and gets the required data from Model
- After getting required data, it is passed to view
- And finally, controller sends it to User browser as a Response
Now where comes the routing in the above model that should be the next question and the answer is the below image which shows routing is the first important thing in MVC.

Step by Step Routing Implementation
In ASP.NET traditional web forms, what happens is user is directly redirected to the resources requested but in MVC, it matches the incoming requests that would otherwise match a resource on file system and maps the request to a controller action. Basically, I would say it constructs the outgoing URLs that correspond to controller actions.
Some of the developers compare ASP.NET routing with URL rewriting and the key difference between the two is Routing generates URLs using the same mapping that it used to match incoming URLs. On the other hand, URL rewriting applies to incoming requests and does not help in generating same URLs.
How to Define a Route
Defining Route starts with a URL Pattern which specifies the pattern which the route will match. Along with the route, URL route can also specify default values and constraints for the various parts of the URL providing tight control over how and when the route matches incoming request URLs.
One Routing URL pattern can serve for a group of similar URLs request by a user and the pattern helps the routing engine to correctly serve the URL with correct controller and actions.
In ASP.NET MVC, Routing is supported by default. We can register route patterns in RegisterRoutes (RouteCollection routes)
method from the Application_Start
eventhandler of Global.asax file. Where in, RouteCollection
is a collection of routes registered with the application.
The default route pattern is given as {controller}/{action}/{id}. Below is the sample of code where the routes are registered.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
namespace MVCWithEF
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home",
action = "Index", id = UrlParameter.Optional },
namespaces: new string[] { "MVCWithEF.Controllers" }
);
}
}
}
As we can observe in RegisterRoutes
method, there is a Default Route pattern registered and even we can add our custom route patterns into RouteCollection
.
In the above code, the first line under RegisterRoutes
method tells that request having .asxd extention will not be handled by routing of ASP.NET routing as this has to be handled by HTTPHandler
. We can also ensure that routing ignores such request. We can use StopRoutingHandler
and the above modified code would be as below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
namespace MVCWithEF
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.Add(new Route
(
"{resource}.axd/{*pathInfo}",
new StopRoutingHandler()
));
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home",
action = "Index", id = UrlParameter.Optional },
namespaces: new string[] { "MVCWithEF.Controllers" }
);
}
}
}
and the defaults for this pattern are given as:
controller
- Homeaction
- IndexId
- it's an optional parameter as defined by UrlParameter.Optional
Now suppose we have a Controller called Home and inside controller, there is an action called Index
as defined below, then request will be sent to /Home/Index.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MVCWithEF.Common;
using System.Data.Entity;
using System.Web.Caching;
namespace MVCWithEF.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
}
}
Points of Interest
There are some points which need to be discussed such as:
StopRouteHandler
Constraints
- Custom Router
But to learn the basics of router, the above information would be useful.
Working as .Net Technical Lead in a reputed MNC and having more than 8 years of experience in developing .Net Applications