Introduction
This tip provides a simple explanation of how to use NLog for log messages inside biztalk orchestration.
The tip is divided into 4 parts:
- Install NLog for C# application
- Update Biztalk Configuration
- Development
- Configure biztalk administration console [ run sample - get output ]
Getting Started
Part 1: Install NLog for C# Application
- Create new C# application "
HelperLibrary
" - Open package manager console:

- Write command:
Install-Package NLog.Config

- This will install NLog for the current application.

- Edit on
NLog.Config
.
="1.0"="utf-8"
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<targets>
<target name="TraceFile" xsi:type="File"
fileName="C:\logs\BiztalkTraceLogs.log"
layout="
-------------- ${level} (${longdate}) --------------${newline}
${newline}
Additional Info: ${message}${newline}" />
<target name="ErrorFile" xsi:type="File"
fileName="C:\logs\BiztalkErrorLogs.log"
layout="
-------------- ${level} (${longdate}) --------------${newline}
${newline}
Exception Type: ${exception:format=Type}${newline}
Exception Message: ${exception:format=Message}${newline}
Stack Trace: ${exception:format=StackTrace}${newline}
Additional Info: ${message}${newline}" />
</targets>
<rules>
<logger name="*" level="Trace" writeTo="TraceFile" />
<logger name="*" levels="Debug,Error" writeTo="ErrorFile" />
</rules>
</nlog>
Part 2: Update Biztalk Server Configuration
- Navigate to biztalk folder location in program files
Program Files (x86)Microsoft BizTalk Server 2013 R2
- Open BTSNTSvc64.exe or BTSNTSvc.exe according to version of biztalk and update file
="1.0"
<configuration>
<startup useLegacyV2RuntimeActivationPolicy="true">
<supportedRuntime version="v4.0" />
</startup>
<configSections>
<section name="nlog" type="NLog.Config.ConfigSectionHandler, NLog"/>
</configSections>
<runtime>
<assemblyBinding xmlns="urn:schemas-microsoft-com:asm.v1">
<probing privatePath="BizTalk Assemblies;Developer Tools;Tracking;Tracking\interop" />
</assemblyBinding>
</runtime>
<system.runtime.remoting>
<channelSinkProviders>
<serverProviders>
<provider id="sspi"
type="Microsoft.BizTalk.XLANGs.BTXEngine.
SecurityServerChannelSinkProvider,Microsoft.XLANGs.BizTalk.Engine"
securityPackage="ntlm" authenticationLevel="packetPrivacy" />
</serverProviders>
</channelSinkProviders>
<application>
<channels>
<channel ref="tcp" port="0" name="">
<serverProviders>
<provider ref="sspi" />
<formatter ref="binary" typeFilterLevel="Full"/>
</serverProviders>
</channel>
</channels>
</application>
</system.runtime.remoting>
<nlog file=" NLog.config" />
</configuration>
- Copy NLog.config file to biztalk server location
Program Files (x86)Microsoft BizTalk Server 2013 R2
Part 3: Development
- Add NLogManager.cs Class to
HelperLibrary
C# application:
using NLog;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HRManagement.HelperLibrary
{
[Serializable()]
public static class NLogManager
{
private static readonly Logger logman = LogManager.GetCurrentClassLogger();
public static void Log(LogType level, string message, Exception exception)
{
switch (level)
{s
case LogType.Error:
logman.Log(LogLevel.Error, exception , message , null);
break;
case LogType.Info:
logman.Log(LogLevel.Info, exception, message, null);
break;
case LogType.Warning:
logman.Log(LogLevel.Warn, exception, message, null);
break;
case LogType.Trace:
logman.Log(LogLevel.Trace, exception, message, null);
break;
}
if (exception.InnerException != null)
{
Log(level, message, exception.InnerException);
}
}
public static void Log(LogType level, string message)
{
switch (level)
{
case LogType.Error:
logman.Log(LogLevel.Error, message);
break;
case LogType.Info:
logman.Log(LogLevel.Info, message);
break;
case LogType.Warning:
logman.Log(LogLevel.Warn, message);
break;
case LogType.Trace:
logman.Log(LogLevel.Trace, message);
break;
} }
}
[Serializable()]
public enum LogType
{
Error, Info, Warning, Trace
}
}
-
Build HelperLibrary
and deploy to GAC, deploy helper to gac like Tip1-Add_Dll_To_Gac.

-
Add biztalk application + add reference for helperLibrary
.

-
Create new sample schema to route using orchestration [EmployeeSchema]

- Create your sample orcestration [simple for receive - write log - send]

- Call
HelperLibrary
to use NLogManager
Class to start log and specify LogType
[Sample Trace]
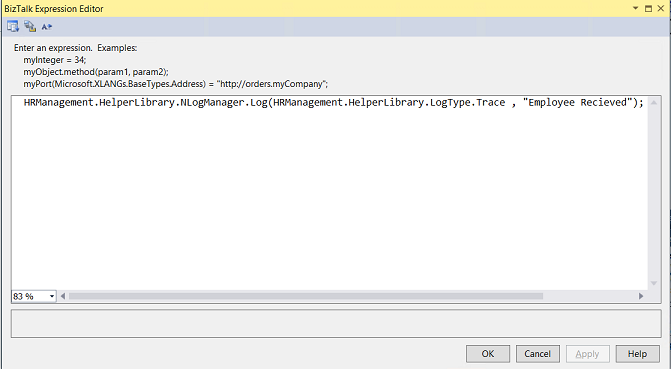
- Deploy Biztalk Application [add strong key + deploy]
Part 4: Configure biztalk Administration Console [Run Sample - Get Output]
- Bound orchestration, create physical receive, send ports
- Add resources of nlog, helper DLL
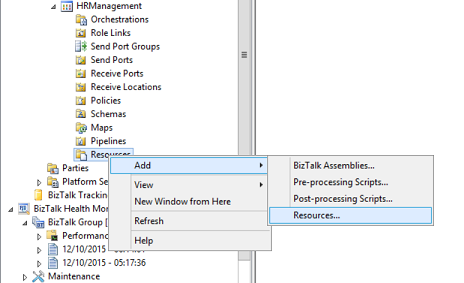

- Route messages [Employee Schema] from receive to send port
- Get Results in
sendPort
, you find the log [BiztalkTraceLogs.txt] in folder specified in NLog.Config
[c:\logs\]

- Finally, you can add more logs for any shape in orchestration, and enjoy logs types and collect all logs into one file or multiple according to configuration in
NLog.config
.