Introduction
So often when learning about a specific API or a new technology, I create many small solutions, and by time the list of solutions grows outside what Visual Studio can display in its start page, soon the list of most recently used solutions becomes not very useful since it displays lots of solutions that I do not really use any more.
Since editing the list form Visual Studio is not possible [at least I do not know about any option or dialog from within VS that lets us edit this list or clear it altogether], I have created this little application to clear things out.
Background
I was looking in CodeProject for an application that does what I was looking to, and I found this article: Visual Studio Project MRU List Editor that explains where Visual Studio saves the MRU list. But because I had more than a version of Visual Studio on my machine, I have created this app to handle different versions.
Here is the application at runtime:
Choose your Visual Studio version:
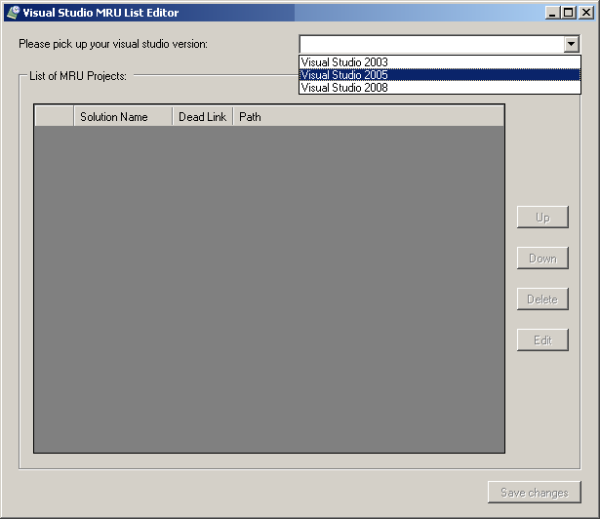
Visual Studio 2005 MRU displayed:

Navigating the MRU list:

Edit an item:

Item updated:

Important Note
Visual Studio instances do rewrite the list when they are closed; to effectively clear the MRU list, all instances of the target Visual Studio should be closed.
Using the Code
All it takes to build this application is to read the Registry entries and rewrite them.
Visual Studio stores the MRU list in the following registry location: HKEY_CURRENT_USER\Software\Microsoft\VisualStudio\XX\ProjectMRUList, where XX is the version number of Visual Studio.
- Visual Studio 2002 - 7.0
- Visual Studio 2003 - 7.1
- Visual Studio 2005 - 8.0
- Visual Studio 2008 - 9.0
- Visual Studio 2010 - 10.0
There is a class called Util
that stores these values in a Hashtable
:
internal static class Utils
{
private static Hashtable visualStudioVersions = new Hashtable();
private static string commonPath = @"Software\Microsoft\VisualStudio";
private static string projectMRUList = "ProjectMRUList";
public static Hashtable VisualStudioVersions
{
get { return visualStudioVersions; }
}
static Utils()
{
visualStudioVersions.Add("7.0", "Visual Studio 2002");
visualStudioVersions.Add("7.1", "Visual Studio 2003");
visualStudioVersions.Add("8.0", "Visual Studio 2005");
visualStudioVersions.Add("9.0", "Visual Studio 2008");
visualStudioVersions.Add("10.0", "Visual Studio 2010");
}
public static List<VisualStudioVersion> GetInstalledVersions()
{
List<VisualStudioVersion> installedVersions = null;
RegistryKey visualStudio = null;
try
{
visualStudio = Registry.CurrentUser.OpenSubKey(commonPath);
if (visualStudio != null)
{
installedVersions = new List<VisualStudioVersion>();
foreach (string key in visualStudio.GetSubKeyNames())
{
if (visualStudioVersions.ContainsKey(key))
{
installedVersions.Add(new VisualStudioVersion(key,
(string)visualStudioVersions[key]));
}
}
}
}
catch
{
}
finally
{
if (visualStudio != null)
visualStudio.Close();
}
return installedVersions;
}
public static List<string> GetMRUList(VisualStudioVersion version)
{
List<string> mruList = null;
RegistryKey mruRegistryKey = null;
try
{
if (version != null)
{
mruRegistryKey = Registry.CurrentUser.OpenSubKey(
String.Format(@"{0}\{1}\{2}",
commonPath, version.Version, projectMRUList));
if(mruRegistryKey != null)
{
mruList = new List<string>();
string[] fileKeys = mruRegistryKey.GetValueNames();
if (fileKeys != null && fileKeys.Length > 0)
{
foreach (string key in fileKeys)
{
mruList.Add((string)mruRegistryKey.GetValue(key));
}
}
}
}
}
catch
{
}
finally
{
if (mruRegistryKey != null)
mruRegistryKey.Close();
}
return mruList;
}
public static void SaveMruList(VisualStudioVersion version,
List<VisualStudioSolution> solutions)
{
RegistryKey mruRegistryKey = null;
try
{
if (solutions != null && version != null)
{
mruRegistryKey =
Registry.CurrentUser.OpenSubKey(String.Format(@"{0}\{1}\{2}",
commonPath, version.Version, projectMRUList), true);
if (mruRegistryKey != null)
{
foreach (string key in mruRegistryKey.GetValueNames())
{
mruRegistryKey.DeleteValue(key);
}
for (int i = 0; i < solutions.Count; i++)
{
mruRegistryKey.SetValue("File" +
(i + 1).ToString(), solutions[i].SolutionPath);
}
}
}
}
catch
{
}
finally
{
if (mruRegistryKey != null)
mruRegistryKey.Close();
}
}
internal class VisualStudioVersion
{
public VisualStudioVersion(string version, string description)
{
this.version = version;
this.description = description;
}
private string version;
public string Version
{
get { return version; }
set { version = value; }
}
private string description;
public string Description
{
get { return description; }
set { description = value; }
}
}