I believe that to write high-quality tests, we need to prevent the copy-paste development. To write UI tests, we usually utilize the Page Object Design Pattern. Most people to create a new page, they copy an existing one and delete the redundant code. I believe that this practice is error prone and tedious. Because of that in this publication, I am going to show you how to create item templates to solve this problem. You can find similar solutions in the other articles from the Automation Tools Series.
Quick Navigation
What is an Item Template?
Definition
The metadata file (*.vstemplate) that Visual Studio uses to define how to display the project item in the integrated development environment (IDE) and, if you have specified the appropriate properties, to customize how the project item is created.
How to Create Single Page Template for Standard Page Object?
In my article, Page Object Pattern in Automated Testing, I showed you how to use the default WebDriver
Page Objects. Below, you can find the default code for a regular page.
public class BingMainPage
{
private readonly IWebDriver driver;
private readonly string url = @"http://www.bing.com/";
public BingMainPage(IWebDriver browser)
{
this.driver = browser;
PageFactory.InitElements(browser, this);
}
[FindsBy(How = How.Id, Using = "sb_form_q")]
public IWebElement SearchBox { get; set; }
[FindsBy(How = How.Id, Using = "sb_form_go")]
public IWebElement GoButton { get; set; }
[FindsBy(How = How.Id, Using = "b_tween")]
public IWebElement ResultsCountDiv { get; set; }
public void Navigate()
{
this.driver.Navigate().GoToUrl(this.url);
}
public void Search(string textToType)
{
this.SearchBox.Clear();
this.SearchBox.SendKeys(textToType);
this.GoButton.Click();
}
public void ValidateResultsCount(string expectedCount)
{
Assert.IsTrue(this.ResultsCountDiv.Text.Contains(expectedCount),
"The results DIV doesn't contains the specified text.");
}
}
Usually, if you want to create a new page, you will copy one of the existing pages, remove the redundant code and start to add the new logic. However, for me, this process is tedious and error prone. Because of that, I believe that if we create a template for this boilerplate code, we will reduce the amount of the required work and errors.
Create Clean Default Template
- Copy the above page and start deleting the redundant code. Leave only the bare minimum required.
using Microsoft.VisualStudio.TestTools.UnitTesting;
using OpenQA.Selenium;
using OpenQA.Selenium.Support.PageObjects;
namespace PageObjectPattern.Selenium.Bing.Pages
{
public class PageTemplate
{
private readonly IWebDriver driver;
private readonly string url = @"http://www.bing.com/";
public PageTemplate(IWebDriver browser)
{
this.driver = browser;
PageFactory.InitElements(browser, this);
}
[FindsBy(How = How.Id, Using = "sb_form_q")]
public IWebElement SampleElement { get; set; }
public void Navigate()
{
this.driver.Navigate().GoToUrl(this.url);
}
public void SampleAction()
{
}
public void AssertIsTrue(bool expectedCondition = true)
{
Assert.IsTrue(expectedCondition);
}
}
}
- Now it is time to export the file as a template. On the File menu, click Export Template.
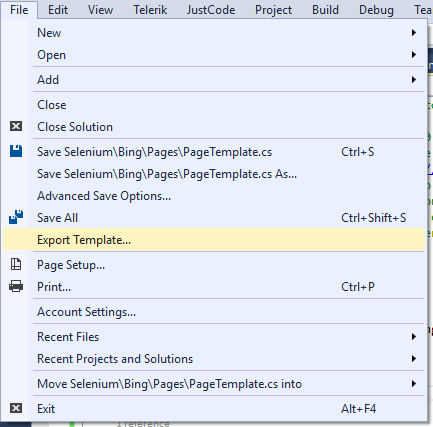
- Click Item Template, select the project that contains the item and click Next.

- Select the item for which you want to create a template, and click Next.

- You can select the assembly references to be included in the template, but we will skip this part.

?
- Next type the icon file name, preview image. You can find the most appropriate from some of the most popular sites such as FlatIcon.

- Type the template name and description, and click Finish.

The files for the template are added to a .zip file and copied to whatever directory you specify in the dialog. The default location is ..Users/<username>/Documents/Visual Studio <Version>/My Exported Templates/ folder.
Import Exported Templates in Visual Studio

By default, the template is imported automatically. However, if for some reason, you have deleted it, you need to copy the exported template to ..Users/<username>/Documents/Visual Studio <Version>/Templates/ItemTemplates/.
How to Use the Created Items Templates?
To use your new template, add a new item:

It is possible not to see your template at first when you open the new item window. To fix the problem, close all Visual Studio instances and open your solutions' folder. Delete the .vs folder.

After that, open your solution again and try to create a new item.

The following new file is created:
using Microsoft.VisualStudio.TestTools.UnitTesting;
using OpenQA.Selenium;
using OpenQA.Selenium.Support.PageObjects;
namespace PageObjectPattern
{
public class MyNewPage
{
private readonly IWebDriver driver;
private readonly string url = @"http://www.bing.com/";
public MyNewPage(IWebDriver browser)
{
this.driver = browser;
PageFactory.InitElements(browser, this);
}
[FindsBy(How = How.Id, Using = "sb_form_q")]
public IWebElement SampleElement { get; set; }
public void Navigate()
{
this.driver.Navigate().GoToUrl(this.url);
}
public void SampleAction()
{
}
public void AssertIsTrue(bool expectedCondition = true)
{
Assert.IsTrue(expectedCondition);
}
}
}
The class name and the filename are automatically changed to the value that you have specified in the new item window.
This is how the current version of the exported template looks like - MyTemplate.vstemplate file.
<VSTemplate Version="3.0.0"
xmlns="http://schemas.microsoft.com/developer/vstemplate/2005" Type="Item">
<TemplateData>
<DefaultName>WebDriverPageObjectItemTemplate.cs</DefaultName>
<Name>WebDriverPageObjecttemTemplate</Name>
<Description>Creates a WebDriver Page Object</Description>
<ProjectType>CSharp</ProjectType>
<SortOrder>10</SortOrder>
<Icon>__TemplateIcon.png</Icon>
<PreviewImage>__PreviewImage.png</PreviewImage>
</TemplateData>
<TemplateContent>
<References />
<ProjectItem SubType="" TargetFileName="$fileinputname$.cs"
ReplaceParameters="true">PageTemplate.cs</ProjectItem>
</TemplateContent>
</VSTemplate>
Download the Page Object Item Template
Automation Tools
References
The post Standardize Page Objects with Visual Studio Item Templates appeared first on Automate The Planet.
All images are purchased from DepositPhotos.com and cannot be downloaded and used for free.
License Agreement