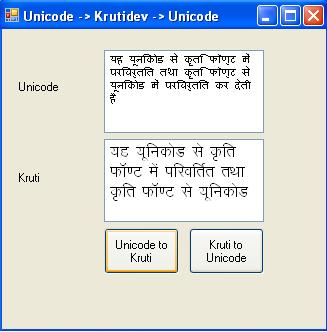
Introduction
This application is created in C# for solving major problem of Text Conversion from Unicode to Krutidev and vice-versa.
Background
When I was trying to convert Unicode to Krutidev, I could not found any offline / desktop solution for it with the source code, then I created this for
the solution by using HTML page with JavaScript, which was available by Rajbhasha.net, using JavaScript for it, and using Browser control for local execution
of JavaScript and Firing Event of HTML control.



Using the code
private string UnicodetoKruti(string strInput)
{
string strOutPut = "";
HtmlElementCollection theElementCollection;
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if ((curElement.GetAttribute("id").ToString() == "unicode_text"))
{
curElement.SetAttribute("Value", strInput);
}
}
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if (curElement.GetAttribute("id").Equals("converter"))
{
curElement.InvokeMember("click");
}
}
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if ((curElement.GetAttribute("id").ToString() == "legacy_text"))
{
strOutPut = curElement.GetAttribute("Value");
}
}
return strOutPut;
}
private string KrutitoUnicode(string strInput)
{
string strOutPut = "";
HtmlElementCollection theElementCollection;
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if ((curElement.GetAttribute("id").ToString() == "legacy_text"))
{
curElement.SetAttribute("Value", strInput);
}
}
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if (curElement.GetAttribute("id").Equals("converttounicode"))
{
curElement.InvokeMember("click");
}
}
theElementCollection = webBrowser1.Document.GetElementsByTagName("input");
foreach (HtmlElement curElement in theElementCollection)
{
if ((curElement.GetAttribute("id").ToString() == "unicode_text"))
{
strOutPut = curElement.GetAttribute("Value");
}
}
return strOutPut;
}