Introduction
I’m writing now to share my experience in Quartz.net. With this library, you will be able to build background task in your web application.
Background
This tip is intended for developers who are familiar with the .NET Framework and develop their web applications using ASP.NET.
Imagine that you want to:
- Send newsletter for members every day, week, or fortnight
- Send some reminders by email at specific time, so what should you do?
- Clear some temporary folder in regular period
Indeed, you should use one of the two strategies to implement that:
- Host Windows service in your IIS in a traditional way.
- Build your service programmatically and let it start when your application started.
In my opinion, the second strategy is not more efficient than the first only, but more portable as well.
Keep in your mind, the better you implement it, the more quality you will get, below you can find the major steps to do that in the simplest way.
Using the Code
Firstly, you should install Quartz library via NuGet manager.
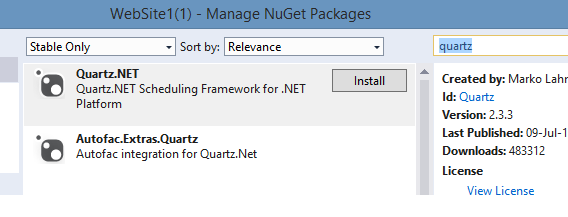
Secondly, you should implement IJob
interface which has one method to be overridden and do your stuff inside.
public class YourJob : IJob
{
public void Execute (IJobExecutionContext context)
{
}
}
Then, you should build trigger to run the previous code in each regular period as follows:
public class SomeScheduler
{
public static void Start()
{
IScheduler scheduler = StdSchedulerFactory.GetDefaultScheduler();
scheduler.Start();
IJobDetail job = JobBuilder.Create<YourJob>().Build();
ITrigger trigger = TriggerBuilder.Create()
.WithDailyTimeIntervalSchedule
(s =>
s.WithIntervalInHours(24)
.OnEveryDay()
.StartingDailyAt(TimeOfDay.HourAndMinuteOfDay(0, 0))
)
.Build();
scheduler.ScheduleJob(job, trigger);
}
}
s.WithIntervalInHours(24)
means that YourJob.Execute();
will trigger every hour.
Finally, you can start your background task at the start of application.
Suppose we develop an ASP.NET web application, so we can call start in Application_Start
method in Global.asax.
protected void Application_Start()
{
SomeScheduler.Start();
}
So, we can summarize the process by these steps:
- Install Quartz from NuGet manager.
- Build
YourJob
class. - Build your scheduler.
- Start your task at the start of your application.
I hope that I present a useful way to implement your background task in the simplest way.
Please don’t hesitate to ask me for any further assistance.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.