Introduction
This article will show you how to make an advanced sticky navbar with side panel using the UIKit (http://getuikit.com/) framework. I'm hoping to introduce more people to this great new framework.
Click the image to see the code in action.
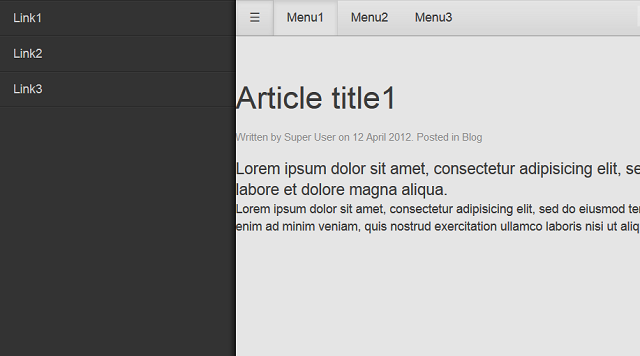
Background
Why UIKit? I was starting a new project with a specific design in mind. Since my site was going to have a lot of information, I didn't want to overwhelm the users with a super complex navigation menu. I wanted to make it as intuitive as I could by hiding options until needed and reducing sections of the site into a single page. To do this, I wanted a sticky navbar with scroll-spy, smooth scrolling and have a second off-canvas navigation (a.k.a side panel) to be revealed by using the Trigram of Heaven (a.k.a the hambuger). For those who don't know what a sticky navbar or scroll-spy looks like (or just don't know what they're called - this can be a pain since there is no standard naming convention), you can find a good example here: http://startbootstrap.com/templates/scrolling-nav/ As you scroll down the page, the navbar travels with you and knows when you've made it to a new section to highlight the appropriate menu item. Since I had done my last few projects with bootstrap this was a perfect template to start off with. It was great that Bootstrap 3 had these features baked in. The only thing I had to do was to add in the off-canvas menu. Easy huh? Unfortunately Bootstrap doesn't include a sidepanel widget, but there was plenty of plugins which could add this functionality:
- SidebarJS: http://makotot.github.io/sidebar/
- Slidebars: http://plugins.adchsm.me/slidebars/usage.php - it even has the sticky navbar on the example! Minus the scroll-spy and smooth scroll features.
- Sidr: http://www.berriart.com/sidr/
Even if the plug-ins didn't work I could always code it myself from scratch as I did in my last project. However, when I went to have all three of these items together, nothing seemed to work right. I tried multiple different plugins to try to add the off-canvas menu but each either had z-index issues or failed to load completely. If I got the off-canvas nav to work, the scrolling would shut down or not operate smoothly. Trying to debug the issues made me think Bootstrap might be getting a little too complex and no longer amenable to working with plug-ins. Time to see if there was anything else out there:
- JQuery UI: I had abandoned this for Bootstrap a few years ago since it didn't seem to be evolving quick enough. I figured since everyone uses JQuery that it would have lots of active development. I was hoping they would have finished version 2.0 by now. Nope, not even a recent mention of it on their blog.
- Foundation: It's now on version 5! Not bad. It has a sticky nav, off-canvas and tooltips. It's not the greatest looking UI, but not bad.
- UIKit (http://getuikit.com/): At first I thought this was a newer version of http://visionmedia.github.io/uikit/, but it doesn't appear to be related despite the name. It looked good and seemed to have everything I was looking for, plus much more.
Their panels weren't using div tags, instead it used HTML 5 article tags - a better way to organize. The articles also included different styles for titles, metas, leads and content. The styling was modern with drop shadows and gradients. According to github, it was still in active development. This was it!
Using the code
The first thing we need to do is include our files from the UIKit download. The style shown on the UIKit site actually isn't the default style, it is actually the gradient style. Took me a minute to figure out why things didn't look the same at first. :) You'll also notice they use ProximaNovaLight for a font which isn't included in the download. You can easily find something from Google's font library to replace this if you don't currently have the font. I'm going to use the browser's default font for this example. The sticky file is located in the addons folder and documentation is found in the addons section on their site.
<link rel="stylesheet" href="css/uikit.gradient.min.css" />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.js" type="text/javascript"></script>
<script src="js/uikit.min.js"></script>
<script src="js/sticky.min.js"></script>
We're going to start off with our navbar. We can use the HTML 5 'nav' tags to section off this component. UIKit uses style classes to define all the components similar to Bootstrap.
uk-navbar
lets us define our navbar uk-navbar-attached
styles the navbar to look nice when attached to the top but doesn't not attach it to the top of the site
Next we have some data properties - data is another HTML 5 trick used to define attributes which can be used by JavaScript. This is also similar to Bootstrap.
- To make the navbar actually stick to the top of the screen, we included the sticky js file and reference the
data-uk-sticky
attribute in the nav tag. - To make the navbar highlight when we scroll or navigate to each section of our site, we need to include the scrollspy nav and set it to highlight the closest item in our nav list. I'm not sure why sticky is considered an addon while scrollspy is a part of the basic package.
- To make the menu scroll smoothly from menu item to menu item, I've added the
data-uk-smooth-scroll
attribute to the anchor elements - The
uk-active
attribute makes sure the menu1 item is highlighted when we first go to the page - The ☰ entity is our hamburger symbol.
<nav class="uk-navbar uk-navbar-attached" data-uk-sticky data-uk-scrollspy-nav="{closest:'li'}">
<ul class="uk-navbar-nav">
<li><a href="#sidepanel">☰</a></li>
<li class="uk-active"><a href="#Menu1" data-uk-smooth-scroll>Menu1</a></li>
<li><a href="#Menu2" data-uk-smooth-scroll >Menu2</a></li>
<li><a href="#Menu3" data-uk-smooth-scroll>Menu3</a></li>
</ul>
Now that we have our navbar, let's make some content. I'm going to use the article tags to define three different sections - one for each of our menu items. In pure web style, it's filled with the usual Lorem Ipsum jargon grabbed straight from their site. For each we're going to put an anchor tag and set it's id equal to each of our menus. Each one I've added a section-gray or section-white class so we can add some of our own styling.:
<a id='Menu1'></a>
<article class="uk-article section-white">
<h1 class="uk-article-title">Article title1</h1>
<p class="uk-article-meta">Written by Super User on 12 April 2012. Posted in Blog</p>
<p class="uk-article-lead">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p><br /> Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
</article>
<a id='Menu2'></a>
<article class="uk-article section-gray">
<h1 class="uk-article-title">Article title2</h1>
<p class="uk-article-meta">Written by Super User on 12 April 2012. Posted in Blog</p>
<p class="uk-article-lead">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex </article>
<a id='Menu3'></a>
<article class="uk-article section-white">
<h1 class="uk-article-title">Article title3</h1>
<p class="uk-article-meta">Written by Super User on 12 April 2012. Posted in Blog</p>
<p class="uk-article-lead">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqea commodo consequat.
</article>
In order to make our articles take up more room, we're going to expand them to the height of the screen. I've also made every other one different colors so we can see the transition better. The padding at the top makes it so our sticky header doesn't run into our article titles. Here is our snippet of CSS to do this.
.section-gray{
height:100%;
width:100%;
background: #eee;
padding-top:50px;
min-height:800px;
}
.section-white{
height:100%;
width:100%;
background: #fff;
padding-top:50px;
min-height:800px;
}
The last thing we need to do is create the side-panel content. We do this by matching up the anchor tag from our hamburger with the id of our sidepanel which has a class of uk-offcanvas
. This will be used to overlay the content of the page so focus is set only to the side-panel. The uk-offcanvas-bar
is the panel itself and UIkit even has a special class to style the side-panel links.:
<div id="sidepanel" class="uk-offcanvas">
<div class="uk-offcanvas-bar">
<ul class='uk-nav uk-nav-offcanvas' data-uk-nav=''>
<li><a href=''>Link1</a><li>
<li><a href=''>Link2</a><li>
<li><a href=''>Link3</a><li>
</ul>
</div>
</div>
That's it! No additional plug-ins, very little custum coding and the site looks great.
If you like this, you might like some of my other articles: http://www.codeproject.com/Articles/693841/Making-Dashboards-with-Dc-js-Part-Using-Crossfil
I'm a Principal Performance Engineer who uses VB.Net, HTML, CSS, etc. to write automation tools and reports.