Introduction
This article is about a neat way I found to filter data in a DataGridView
. This works with all grid view I've ever made with very little code change.
Background
This stems from a similar solution I found. It was too complicated for a beginner like me, so I developed a solution that I could understand.
Using the Code
Step 1: add the following code to the form containing the DataGridView
. In this case, the form is called "DataGridViewForm
".
Private Sub PartsDataGridView_ColumnHeaderMouseClick(ByVal sender As Object, _
ByVal e As System.Windows.Forms.DataGridViewCellMouseEventArgs) _
Handles PartsDataGridView.ColumnHeaderMouseClick
Search.Show()
End Sub
Step 2: Add a form to your project called Search.vb and set the properties the way you would like. For instance, I have set the form properties FormBorderStyle=None
, TopMost=True
, BackgroundColor
, etc.
I added a Label
, TextBox
, and two Button
s.
This is the code-behind of the form.
Public Class Search
Dim columnIndex As Integer
Dim columnName As String
Private Sub Search_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
Dim loc As Point
loc = Control.MousePosition
Me.Location = loc
Me.TextBox1.Focus()
columnIndex = DataGridViewForm.PartsDataGridView.SortedColumn.Index
columnName = DataGridViewForm.PartsDataGridView.Columns(_
columnIndex).HeaderText.ToString
Label1.Text = columnName
End Sub
Private Sub btnSearch_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnSearch.Click
Try
DataGridViewForm.PartsBindingSource.Filter = _
String.Format("{0} = '{1}'", _
DataGridViewForm.ShopPartsDataSet.Parts.Columns(_
columnIndex).ColumnName.ToString, TextBox1.Text)
Catch
MessageBox.Show("Database error")
End Try
Me.Close()
End Sub
Private Sub btnExit_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnExit.Click
Me.Close()
End Sub
End Class
As you can see in the picture, when you click on the header, a window pops up. You enter some text, a part number in this case, and hit the binoculars. Voila!! Your data is filtered.
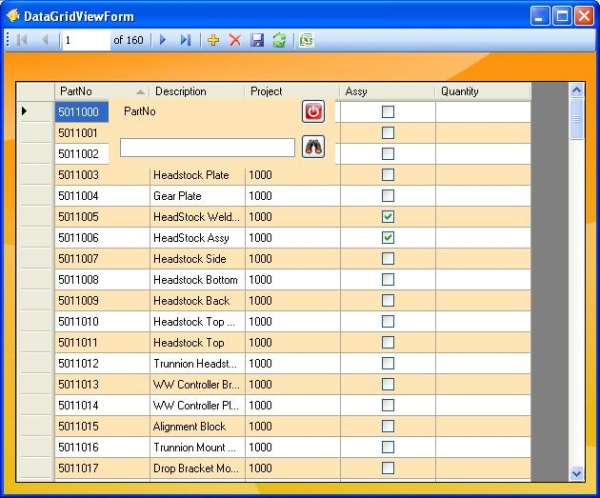
It's super easy!! This should work with any existing form you have out there!!
Points of Interest
I'm not an expert. One thing that I wasn't able to figure out was how to get the selected column index. So that's why I don't have the Search Form popup when I right click. I need to left click, let the DataGrid
sort, and get the index of the sorted column.
Also, I added a button to my ToolStrip
to clear the filter and re-fill the DataGrid
.
Obviously, you will want to change the code to apply this to your project.
"DataGridViewForm" = "Yourform".
"PartsBindingSource" = "YourBindingSource"
"ShopPartsDataSet" = "YourDataSet"
"PartsDataGridView" = "YourDataGridView"
History
No history. Brand new!!