Table of Contents
Thanks to everyone who has knowingly / unknowingly helped me to become a Microsoft MVP, hope I can live up to it.
In this section, we will understand the round trip issues of LINQ and how we can overcome the same using ‘DataLoadOptions
’. One of the biggest issues with LINQ to SQL is that it fires SQL query for every object which has a huge impact on performance. In this article, we will see how we can get all data in one SQL query.
This article assumes that you have basic knowledge of how entity objects can be flourished using LINQ. In case you are not aware of basics of LINQ to SQL mapping, you can read my article to understand the basic LINQ concepts.
First let’s try to understand how LINQ queries actually work and then we will see how round trips happen. Let’s consider the below database design where we have 3 tables -- customer
, addresses
and phone
. There is one-many relationship between customer
and addresses
, while there is one-one relationship between address
table and phones
.
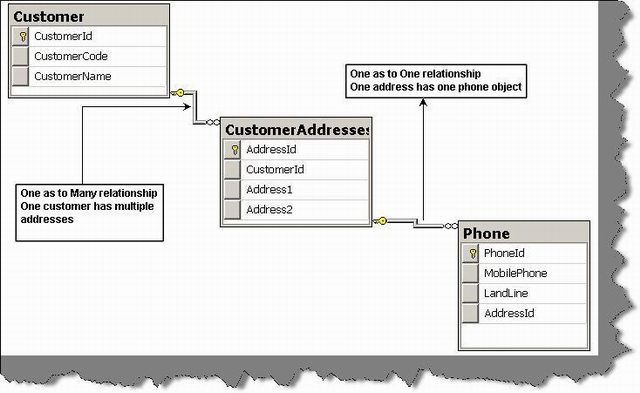
We have created three entities as per the table design:
ClsCustomerWithAddresses
ClsAddresses
ClsPhone
We have defined the relationships between them using ‘EntitySet
’ and ‘EntityRef
’.

To fill the entity objects with data from table is a 5 step process. As a first step, the datacontext
connection is created using the connection string, LINQ query is created and then we start browsing through customer
, address
and phones
.

Ok, now that we have analyzed that it takes 5 steps to execute a LINQ query, let’s try to figure out on which step the LINQ query actually fires SQL to the database. So what we will do is run the above LINQ code and analyze the same using SQL profiler.
Just so that we do not catch a lot of SQL Server noise, we have only enabled RPC and SQL batch events.

Now when you run the query, you will find the below things:
- The execution of actual SQL takes place when the
foreach
statement is iterated on the LINQ objects. - The second very stunning thing you will notice is that for every entity, a separate query is fired to SQL Server. For instance, for
customer
one query is fired and then separate queries for address
and phones
are fired to flourish the entity object. In other words, there are a lot of round trips.

We can instruct LINQ engine to load all the objects using ‘DataLoadOptions
’. Below are the steps involved to enable ‘DataLoadOptions
’.
The first step is to create the data context class:
DataContext objContext = new DataContext(strConnectionString);
The second step is to create the ‘DataLoadOption
’ object:
DataLoadOptions objDataLoadOption = new DataLoadOptions();
Using the LoadWith
method, we need to define that we want to load customer
with address
in one SQL.
objDataLoadOption.LoadWith<clsCustomerWithAddresses>
(clsCustomerWithAddresses => clsCustomerWithAddresses.Addresses);
Every address
object has phone
object, so we have also defined saying that the phone
objects should be loaded for every address
object in one SQL.
objDataLoadOption.LoadWith<clsAddresses>(clsAddresses => clsAddresses.Phone);
Whatever load option you have defined, you need to set the same to the data context object using ‘LoadOptions
’ property.
objContext.LoadOptions = objDataLoadOption;
Finally prepare your query:
var MyQuery = from objCustomer in objContext.GetTable<clsCustomerWithAddresses>()
select objCustomer;
Start looping through the objects:
foreach (clsCustomerWithAddresses objCustomer in MyQuery)
{
Response.Write(objCustomer.CustomerName + "<br>");
foreach (clsAddresses objAddress in objCustomer.Addresses)
{
Response.Write("===Address:- " + objAddress.Address1 + "<br>");
Response.Write("========Mobile:- " + objAddress.Phone.MobilePhone + "<br>");
Response.Write("========LandLine:- " + objAddress.Phone.LandLine + "<br>");
}
}
Below is the complete source code for the same:
DataContext objContext = new DataContext(strConnectionString);
DataLoadOptions objDataLoadOption = new DataLoadOptions();
objDataLoadOption.LoadWith<clsCustomerWithAddresses>
(clsCustomerWithAddresses => clsCustomerWithAddresses.Addresses);
objDataLoadOption.LoadWith<clsAddresses>(clsAddresses => clsAddresses.Phone);
objContext.LoadOptions = objDataLoadOption;
var MyQuery = from objCustomer in objContext.GetTable<clsCustomerWithAddresses>()
select objCustomer;
foreach (clsCustomerWithAddresses objCustomer in MyQuery)
{
Response.Write(objCustomer.CustomerName + "<br>");
foreach (clsAddresses objAddress in objCustomer.Addresses)
{
Response.Write("===Address:- " + objAddress.Address1 + "<br>");
Response.Write("========Mobile:- " + objAddress.Phone.MobilePhone + "<br>");
Response.Write("========LandLine:- " + objAddress.Phone.LandLine + "<br>");
}
}
Abracadabra…. Now if you run the code LINQ has executed only one SQL with proper joins as compared to 3 SQL for every object shown previously.

We have also attached a source code. Run the project and see how the profiler shows different SQL execution. You can first run the ‘EntitySet
’ example and see how SQL profiler reacts for the same and then run the example with ‘DataLoadOptions
’. The SQL script is attached in a different file.
))==:- You can the download the source code from here.

For further reading do watch the below interview preparation videos and step by step video series.