Followed this official tutorial, my own version on GitHub, and you can see it in action here (click the cube to see the effect).
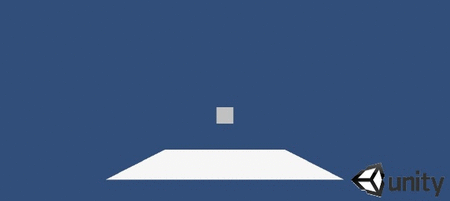
Steps on how to make this:
- Create a new 3D project
- Save the scene as
Level0
- Create -> 3D object ->
Cube
- Create -> Light -> Directional light
- Save this scene and create a new one
Level2
and repeat the process just here create two cubes - Create an empty object called
FaderObj
and attach a new script to it called Fading
:
#pragma strict
public var fadeOutTexture : Texture2D;
public var fadeSpeed : float = 0.8f;
private var drawDepth : int = -1000;
private var alpha : float = 1.0f;
private var fadeDir : int = -1;
function OnGUI()
{
alpha += fadeDir * fadeSpeed * Time.deltaTime;
alpha = Mathf.Clamp01(alpha);
GUI.color = new Color (GUI.color.r, GUI.color.g, GUI.color.b, alpha);
GUI.depth = drawDepth;
GUI.DrawTexture(new Rect(0, 0, Screen.width, Screen.height),
fadeOutTexture);
}
public function BeginFade (direction : int)
{
fadeDir = direction;
return (fadeSpeed);
}
function OnLevelWasLoaded()
{
BeginFade(-1);
}
- Add the following script to the
Cube
object:
#pragma strict
var counter = 0;
function Start () {
}
function Update () {
}
function OnMouseDown(){
Debug.Log("clicked " + counter++);
var fadeTime = GameObject.Find ("FaderObj").GetComponent(FadingJS).BeginFade(1);
yield WaitForSeconds(fadeTime);
GameObject.Find("Cube").renderer.enabled = false;
fadeTime = GameObject.Find ("FaderObj").GetComponent(FadingJS).BeginFade(-1);
yield WaitForSeconds(fadeTime);
Application.LoadLevel(Application.loadedLevel + 1);
}
- Drag a 2×2 black png image to the
Texture
variable in the Fading script.