Introduction
Do you sometimes fail to notice Outlook reminder window? Do you wish a chat window would remain always on top of other windows so that you never miss a message? Do you want to have a notepad always on top so that you can take quick notes anytime, while working on other apps?
We have an app for that.
It runs quietly on system tray and monitors open windows. Whenever it finds a window that you want to make always on top, it does that:

You can use this AlwaysOnTop utility to make any window remain always on top of other windows.

Using the code
You can just download the binaries, extract and run.
https://github.com/oazabir/AlwaysOnTop/blob/master/Binary/AlwaysOnTop.zip?raw=true
The source code is here:
https://github.com/oazabir/AlwaysOnTop
Points of Interest
The app periodically scans the open windows, and checks the title against a set of user defined matches. If the window title contains the user defined words, then it calls SetWindowPos in user32.dll to make the window appear always on top.
Here's how it does it:
public static void AlwaysOnTop(string titlePart)
{
User32.EnumDelegate filter = delegate(IntPtr hWnd, int lParam)
{
StringBuilder strbTitle = new StringBuilder(255);
int nLength = User32.GetWindowText(hWnd, strbTitle, strbTitle.Capacity + 1);
string strTitle = strbTitle.ToString();
if (User32.IsWindowVisible(hWnd) && string.IsNullOrEmpty(strTitle) == false)
{
if (strTitle.Contains(titlePart))
SetWindowPos(hWnd, HWND_TOPMOST, 0, 0, 0, 0, SWP_NOMOVE | SWP_NOSIZE);
}
return true;
};
User32.EnumDesktopWindows(IntPtr.Zero, filter, IntPtr.Zero);
}
The EnumDesktopWindows is defined as:
[DllImport("user32.dll", EntryPoint = "EnumDesktopWindows",
ExactSpelling = false, CharSet = CharSet.Auto, SetLastError = true)]
public static extern bool EnumDesktopWindows(IntPtr hDesktop, EnumDelegate lpEnumCallbackFunction, IntPtr lParam);
Setting window always on top is easy. But removing always on top, is difficult. If you just loop through the windows and call SetWindowPos to remove the always on top flag, it hangs. You have to specifically check if the window is a normal window. For that, some additional code is required:
public static void RemoveAlwaysOnTop()
{
User32.EnumDelegate filter = delegate(IntPtr hWnd, int lParam)
{
StringBuilder strbTitle = new StringBuilder(255);
int nLength = User32.GetWindowText(hWnd, strbTitle, strbTitle.Capacity + 1);
string strTitle = strbTitle.ToString();
if (!string.IsNullOrEmpty(strTitle))
{
WINDOWPLACEMENT placement = GetPlacement(hWnd);
if (placement.showCmd == SW_SHOWNORMAL) SetWindowPos(hWnd, HWND_NOTOPMOST, 0, 0, 0, 0, SWP_NOMOVE | SWP_NOSIZE);
}
return true;
};
User32.EnumDesktopWindows(IntPtr.Zero, filter, IntPtr.Zero);
}
That's all the fun there is!
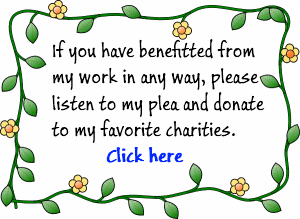