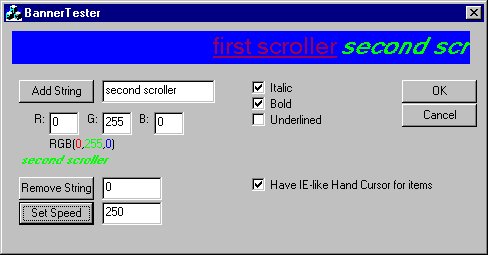
Introduction
Have you ever wanted to include a control in one of your applications that behaves like a web banner ad with scrolling text that whips by. This effect is also seen with the news ticker on AOL Instant Messenger. A bunch of hyperlinks whip by with news headlines, and you click on the headlines to go to the article. Of course, it wouldn't be very useful if all the strings had to have the same style and color. It would be better if each string was able to have its own individual style and color. This type of thing is possible with the CBannerStatic
class. So what do we need to use this control?
Explanation
First off, we're going to need a collection of strings with each string having its own color and style (underlined, italicized, etc) - enter the CString
derived CColorString
class. It uses a DWORD
to hold both the color and value parameters. Since a COLORREF
uses only the low 3 bytes to keep track of colors, I use the high byte to store style information such as whether the string is italicized, underlined or bold. CColorString
can also store a background color; I waste another DWORD
for this. In the future, I may move the style values to BOOL
types so that the code is more understandable; we're supposed to get away from bit-masking operations in C++!
Now that we have a class to encapsulate strings that know their own style, we need a control that knows how to display them. Enter CMultiColorStatic
. It's quite similar to CColorStatic
type controls but it is a static object that will allow multiple strings to be entered with each string having its own style. This is done by having a CPtrArray
to keep track of all of the CColorStrings
that have been added to the control. Granted, there isn't a whole lot of gain by using one CMultiColorStatic
instead of a bunch of CColorStatic
s; I just wanted one control to be the base for CBannerStatic
.
Speaking of CBannerStatic
, that's the last piece of the puzzle. CBannerStatic
combines scrolling with the flexability of multiple strings. For scrolling, it uses multimedia timers so that similar precision can be achieved on any windows platform (with normal timers, Windows NT has 10ms precision, while Win9x has only 55ms precision). I use the multimedia timers only for precision; when I receive the timer message, I post a WM_TIMER
message to the control and the drawing is done in the WM_TIMER
handler. When drawing becomes complicated, the app containing the banner could become unresponsive because the drawing takes longer than the scrolling. By this time the drawing is complete and it's time to scroll again. By retaining a message structure, the app will never become unresponsive.
The entire thing is fairly simple to use, but it may take some time getting used to. CBannerStatic
provides a neat way to allow the user to click on an item and get results. The client can set an item cursor as well with an ItemClick
callback function. It'd be too much to explain everything here, but the sample app BannerTester
is pretty comprehensive in demonstrating the feature-set of CBannerStatic
(as well as CMultiColorStatic
).
Some caveats: The control can be slow if it is made to be large and/or has a lot of message strings. It will bog down the entire system - so beware!
One last note: I don't like to use Precompiled Headers and you'll find that if you just use the classes as provided, the compiler will complain about "StdAfx.h"
not being included. If you get this problem, you can just add #include "StdAfx.h"
to each cpp file for each of the three classes.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.