In this article, we are going to see some features of the brand new Visual Studio 2017. This is the second article of the Visual Studio 2017 series. Please be noted that this is not the complete series of new functionalities of Visual Studio 2017. Here, I am going to share only few things to get you started with the new Visual Studio 2017. I hope you will like this. Now let’s begin.
Background
You can always find the first part of this series here. If you never use Visual Studio, you can find some articles and code snippets related to it here.
Let’s Start Then
Let’s say you have an MVC application with a model Customer
as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace VS2017Features.Models
{
public class Customer
{
public string CustId { get; set; }
public string CustName { get; set; }
public string CustCode { get; set; }
}
}
And a controller as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using VS2017Features.Models;
namespace VS2017Features.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
List<Customer> lstCust = new List<Customer>();
Customer cst = new Customer()
{
CustId = "1",
CustName = "Royal Enfield",
CustCode = "CST01"
};
lstCust.Add(cst);
return View(lstCust);
}
}
}
As of now, I am not going to explain the codes, as it is pretty much clear and easy. Now, we are going to see the following topics:
- Run execution to here feature
- The new exception handler
- Redesigned Attach to process box
- Reattach to process
- What is there for JavaScript developer in VS2017
Run Execution to Here Feature
Let’s say we have a breakpoint in our code as follows:
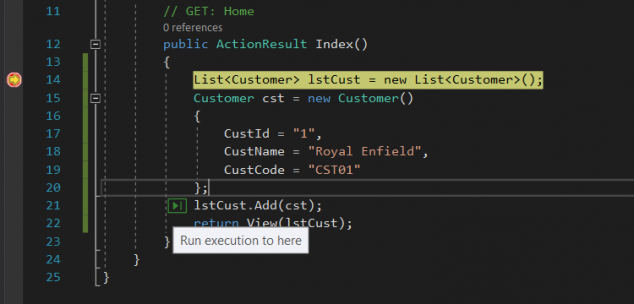
Run_execution_to_here_in_VS2017
Could you notice that there is a small green colour icon in the image, in which you get the text “Run execution to here” while hovering it. This is a new feature in VS2017, if you click on the icon which is near any line of codes, the execution will hit at that point. That’s pretty much simple, right? Now you don’t need to put any unwanted breakpoints for checking out the execution line by line while debugging.
Now what if you are using this feature in a loop. Let’s modify our controller code as follows:
public class HomeController : Controller
{
public ActionResult Index()
{
List<Customer> lstCust = BuildCustomer();
return View(lstCust);
}
private static List<Customer> BuildCustomer()
{
List<Customer> lstCust = new List<Customer>();
for (int i = 0; i < 5; i++)
{
Customer cst = new Customer()
{
CustId = i.ToString(),
CustName = $"CustName{i}",
CustCode = $"CST{i}"
};
lstCust.Add(cst);
}
return lstCust;
}
}
And view as shown below:
@model IEnumerable<VS2017Features.Models.Customer>
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<style>
th, td, tr {
border: 1px solid #ccc;
padding: 10px;
}
</style>
<table>
<thead>
<tr>
<th>Cust Id</th>
<th>Cust Name</th>
<th>Cust Code</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@item.CustId</td>
<td>@item.CustName</td>
<td>@item.CustCode</td>
</tr>
}
</tbody>
</table>
Now let’s run our application.

Application_output
Put a breakpoint in the loop. When you run, you can see that i
value is getting incremented which means, the iterations are happening. Once the iterations are over, the execution comes out. Please note that you can always come out of this loop by changing the execution point.

Run_execution_to_here_in_loop_1

Run_execution_to_here_in_loop_2
The New Exception Popup
The new exception box is really handy which can be resized. As additional features, the inner exceptions are added to the pop up itself. No need to search for the inner exceptions now. To create an exception, we can call the following function:
private void MakeDivideByZeroException()
{
throw new DivideByZeroException();
}
Once you call the above function, you can get an error as follows:

The_new_exception_box
If you click on the view details, a quick watch popup with the error information will be opened.

Quickwatch_in_VS2017
Under the exception settings, you can always set whether you need to throw this type of exception only for the particular DLL or for every DLL.
Redesigned Attach to Process Box
We all know how we can attach to a process in Visual Studio. But have you ever thought, if we have a search box to search a process and attach to it, it would make the task easier? No worries, in VS2017, you get that. To see the redesigned Attach to process window, please click on Debug -> Attach to process.

Redesigned_attach_to_process
Now we have attached our process which was running in Microsoft Edge browser. Wait, the game is not over yet. Please click on the Debug option, you can see an option called Reattach to process. What is that? This option gives you an advantage of reattaching the recent process that you already attached. That’s cool, right? If you have one or more instance of the same process, it will ask you to select whichever you needed.
What is There for JavaScript Developer in VS2017
VS2017 has lots of improvements for JavaScript language. Let’s find out a few of them.
- Advanced JavaScript intellisense
- Added ECMAScript 6
- Introduction of JS Doc
- New Rename options in JavaScript
- Find all references of functions or classes
Advanced JavaScript Intellisense
VS2017 has advanced intellisenses for JavaScript. We don’t need to remember the parameters for the in built functions of JavaScript. For example, if you typed jQuery.ajax()
, you can see the parameters of the functions as follows:

Advanced_JavaScript_intellisence
The best thing is, it even shows exactly the type of the parameter. Yeah, JavaScript types are dynamic
, so no one was creating a proper documentation for JavaScript functions. Now we have an option.
If you right click on the ajax()
function and click on Go To Definition, you can see the definition in a .ts file (TypeScript) as follows:
ajax(settings: JQueryAjaxSettings): JQueryXHR;
ajax(url: string, settings?: JQueryAjaxSettings): JQueryXHR;
In VS2017, all JavaScript related documentations are handled by these TypeScript files.
Added ECMAScript 6
In VS2017, the new ECMAScript 6 features are added which are more intuitive and OOP-style. So we can always use those in our application. Shall we start now?
class Operations {
constructor(x, y) {
this.x = x;
this.y = y;
}
add() {
return (this.x + this.y);
}
}
var myObj = new Operations(1, 2);
console.log(myObj.add());
If you run the above code, you can see an output as follows:

JavaScript_Class_Output
Now, how can you rewrite the above code to lower ECMAScript version? I leave it to you. You can always read more about ECMAScript6 here.
JSDoc
Documenting a JavaScript function was a tough task, but not anymore. In VS2017, this makes it simple. Let’s check it out. By pressing /**
, you can easily document your JavaScript functions and classes. Let us rewrite the class and function as shown below:
class Operations {
constructor(x, y) {
this.x = x;
this.y = y;
}
add() {
return (this.x + this.y);
}
}
var myObj = new Operations(1, 2);
console.log(myObj.add());
You can even set the type of the parameter.

Set_default_type_for_the_parameter_in_JSDoc
If you change the type in the JSDoc, the same will reflect when you create an instance or calling the functions.

JSDoc_reflects_while_creating_instances
New Rename Options in JavaScript
You can now right click on any function name or class name and easily rename those in all references.

New_Rename_option_in_JavaScript
Find All References of Functions or Classes
You can now find the references of your functions or classes easily, just right click and click on Find all references.

Find_all_references_in_JavaScript
That’s all for today. I will come with another set of features of Visual Studio 2017 very soon. Happy coding!
References
See Also
Conclusion
Did I miss anything that you may think is needed? Could you find this post useful? I hope you liked this article. Please share your valuable suggestions and feedback.
Your Turn. What Do You Think?
A blog isn't a blog without comments, but do try to stay on topic. If you have a question unrelated to this post, you're better off posting it on C# Corner, Code Project, Stack Overflow, ASP.NET Forum instead of commenting here. Tweet or email me a link to your question there and I'll definitely try to help if I can.